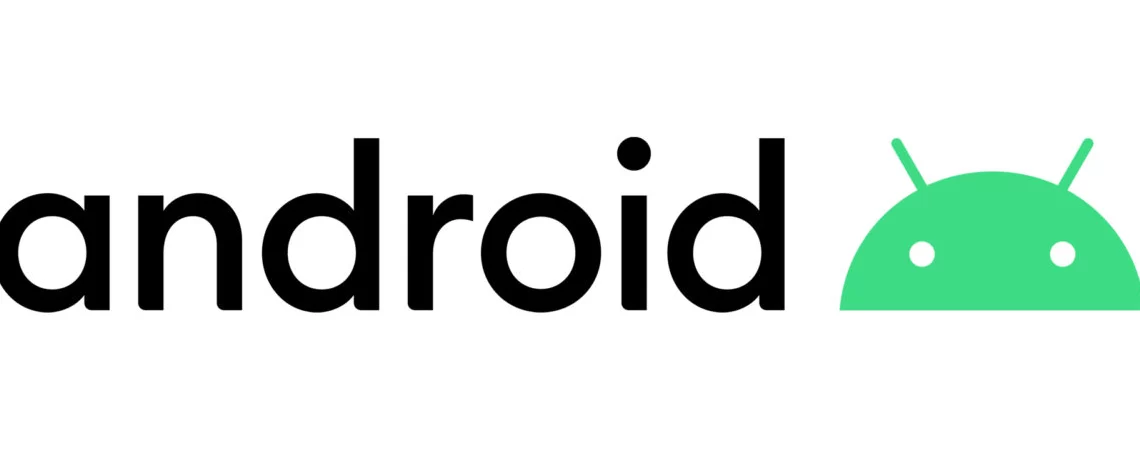
Basics of Android App Development for Bioinformatics
February 22, 2024Table of Contents
Set up Android Studio
To install Android Studio on your computer, follow these steps:
- Visit the official Android developer website at https://developer.android.com.
- Hover over the ‘Tools’ menu and click on ‘Android Studio.’
- On the Android Studio page, click on the ‘Download Android Studio’ button.
- Select your operating system (Windows, macOS, or Linux) and download the appropriate package.
- For Windows users, run the .exe file and follow the installation wizard. Make sure to select ‘Custom’ installation type to choose the installation path. For macOS users, open the downloaded .dmg file and drag the Android Studio icon into the Applications folder.
- After installation, open Android Studio and wait for the initial setup process to complete. This may take several minutes.
- Once the setup is complete, you will be prompted to import or create a new project. You can now start developing Android apps using Java or Kotlin.
Here’s a code snippet for a simple “Hello, World!” app in Java:
1package com.example.myapp;
2
3import android.os.Bundle;
4import android.widget.TextView;
5import androidx.appcompat.app.AppCompatActivity;
6
7public class MainActivity extends AppCompatActivity {
8 @Override
9 protected void onCreate(Bundle savedInstanceState) {
10 super.onCreate(savedInstanceState);
11 TextView textView = new TextView(this);
12 textView.setText("Hello, World!");
13 setContentView(textView);
14 }
15}
For a more user-friendly approach, you can create the UI using XML layout. Here’s the corresponding XML layout code for the “Hello, World!” app:
1<?xml version="1.0" encoding="utf-8"?>
2<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
3 xmlns:app="http://schemas.android.com/apk/res-auto"
4 xmlns:tools="http://schemas.android.com/tools"
5 android:layout_width="match_parent"
6 android:layout_height="match_parent"
7 tools:context=".MainActivity">
8
9 <TextView
10 android:layout_width="wrap_content"
11 android:layout_height="wrap_content"
12 android:text="Hello World!"
13 app:layout_constraintBottom_toBottomOf="parent"
14 app:layout_constraintLeft_toLeftOf="parent"
15 app:layout_constraintRight_toRightOf="parent"
16 app:layout_constraintTop_toTopOf="parent" />
17
18</androidx.constraintlayout.widget.ConstraintLayout>
Remember to replace the package name, activity name, and layout file name with your own.
To create a new project using the Empty Activity template in Android Studio, follow these steps:
- Open Android Studio and click on ‘Create New Project’.
- Select ‘Empty Activity’ as the project template.
- Enter a name for your project, choose the location where you want to save it, and select the minimum SDK version.
- Click on ‘Finish’ to create the project.
Android Studio will generate the necessary files and folders for your project. The main activity file will be named ‘MainActivity.java’ or ‘MainActivity.kt’, depending on the selected language. The layout file will be named ‘activity_main.xml’.
Here’s a code snippet for a simple “Hello, World!” app in Java:
1package com.example.myapp;
2
3import android.os.Bundle;
4import android.widget.TextView;
5import androidx.appcompat.app.AppCompatActivity;
6
7public class MainActivity extends AppCompatActivity {
8 @Override
9 protected void onCreate(Bundle savedInstanceState) {
10 super.onCreate(savedInstanceState);
11 TextView textView = new TextView(this);
12 textView.setText("Hello, World!");
13 setContentView(textView);
14 }
15}
For a more user-friendly approach, you can create the UI using XML layout. Here’s the corresponding XML layout code for the “Hello, World!” app:
1<?xml version="1.0" encoding="utf-8"?>
2<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
3 xmlns:app="http://schemas.android.com/apk/res-auto"
4 xmlns:tools="http://schemas.android.com/tools"
5 android:layout_width="match_parent"
6 android:layout_height="match_parent"
7 tools:context=".MainActivity">
8
9 <TextView
10 android:layout_width="wrap_content"
11 android:layout_height="wrap_content"
12 android:text="Hello World!"
13 app:layout_constraintBottom_toBottomOf="parent"
14 app:layout_constraintLeft_toLeftOf="parent"
15 app:layout_constraintRight_toRightOf="parent"
16 app:layout_constraintTop_toTopOf="parent" />
17
18</androidx.constraintlayout.widget.ConstraintLayout>
Remember to replace the package name, activity name, and layout file name with your own.
Android Studio has a user-friendly interface that includes several key areas:
- Menu Bar: Located at the top of the window, it contains the standard menu options such as File, Edit, View, Navigate, Code, Refactor, Build, Run, Tools, Window, and Help.
- Toolbars: Located below the Menu Bar, the toolbars provide quick access to common actions like creating a new project, opening an existing project, running the app, and debugging.
- Project Explorer: Located on the left side of the window, the Project Explorer displays the file structure of your project. It shows the app modules, libraries, and external libraries used in your project.
- Editor Area: Located in the center of the window, the Editor Area is where you write and edit your code. It supports syntax highlighting, code completion, and error checking.
- Build Variants: Located below the Editor Area, the Build Variants panel allows you to select the build variant for your app. This is useful when you want to build and run different versions of your app for different device configurations.
- Run/Debug Configuration: Located below the Editor Area, the Run/Debug Configuration panel allows you to configure how your app will be built and run. You can specify the device, emulator, or deployment target, as well as launch options.
- Event Log: Located at the bottom of the window, the Event Log displays messages related to the build, run, and debug processes. It also shows notifications about updates, warnings, and errors.
- Bottom Toolbar: Located at the very bottom of the window, the Bottom Toolbar displays the current device or emulator selected for running the app, as well as the app’s version code and version name.
Understanding the Basics of Android App Development
I will provide a brief overview of Activities, Services, Broadcast Receivers, and Content Providers, along with code snippets for each.
Activities
Activities represent a single screen with a user interface. They are the building blocks of an Android app. To create a new activity, you can extend the AppCompatActivity class and override the onCreate() method.
1class MainActivity : AppCompatActivity() {
2 override fun onCreate(savedInstanceState: Bundle?) {
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5 }
6}
Services
Services are used to perform long-running operations in the background, such as playing music, downloading files, or handling network requests. To create a new service, you can extend the Service class and override the onStartCommand() method.
1class MyService : Service() {
2 override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
3 // Perform long-running operation here
4 return super.onStartCommand(intent, flags, startId)
5 }
6
7 override fun onBind(intent: Intent?): IBinder? {
8 return null
9 }
10}
Broadcast Receivers
Broadcast Receivers listen for and respond to system-wide broadcasts. They can be used to perform actions when certain events occur, such as when the device boots up or when a new SMS message is received. To create a new broadcast receiver, you can extend the BroadcastReceiver class and override the onReceive() method.
1class MyBroadcastReceiver : BroadcastReceiver() {
2 override fun onReceive(context: Context?, intent: Intent?) {
3 // Perform action when broadcast received
4 }
5}
Content Providers
Content Providers manage access to a central repository of data. They allow data to be shared between different apps and provide a standard interface for accessing data. To create a new content provider, you can extend the ContentProvider class and override several methods, such as query(), insert(), update(), and delete().
Here’s an example of a simple content provider:
1class MyContentProvider : ContentProvider() {
2 override fun onCreate(): Boolean {
3 return true
4 }
5
6 override fun query(uri: Uri, projection: Array<String>?, selection: String?, selectionArgs: Array<String>?, sortOrder: String?): Cursor? {
7 // Perform query operation here
8 return null
9 }
10
11 override fun insert(uri: Uri, values: ContentValues?): Uri? {
12 // Perform insert operation here
13 return null
14 }
15
16 override fun update(uri: Uri, values: ContentValues?, selection: String?, selectionArgs: Array<String>?): Int {
17 // Perform update operation here
18 return 0
19 }
20
21 override fun delete(uri: Uri, selection: String?, selectionArgs: Array<String>?): Int {
22 // Perform delete operation here
23 return 0
24 }
25
26 override fun getType(uri: Uri): String? {
27 // Return MIME type of data
28 return null
29 }
30}
Remember to register your content provider in the AndroidManifest.xml file:
1<provider
2 android:name=".MyContentProvider"
3 android:authorities="com.example.myapp.MyContentProvider"
4 android:exported="false"
5 android:grantUriPermissions="true" />
These are just basic examples of Activities, Services, Broadcast Receivers, and Content Providers. You can find more detailed information and best practices in the Android documentation.
Understand the Android application architecture
The Android application architecture is a set of components and patterns that help developers build robust and maintainable Android apps. The architecture includes Activities, Services, Broadcast Receivers, and Content Providers, which I covered in my previous response. In addition to these components, the Android application architecture includes several best practices and design patterns.
Model-View-ViewModel (MVVM)
Model-View-ViewModel (MVVM) is a popular architecture pattern used in Android development. It separates the user interface (View) from the data and business logic (Model) using a ViewModel. The ViewModel acts as an intermediary between the View and the Model, holding the data and exposing it to the View through LiveData or other observable data holders.
Here’s a simple example of an MVVM architecture using LiveData:
- Model:
1data class User(val name: String, val age: Int)
- ViewModel:
1class UserViewModel : ViewModel() {
2 val user = MutableLiveData<User>()
3
4 fun fetchUser() {
5 // Perform network request or database query to fetch user data
6 user.value = User("John Doe", 30)
7 }
8}
- View:
1<TextView
2 android:id="@+id/name_text_view"
3 android:layout_width="wrap_content"
4 android:layout_height="wrap_content" />
5
6<TextView
7 android:id="@+id/age_text_view"
8 android:layout_width="wrap_content"
9 android:layout_height="wrap_content" />
1class MainActivity : AppCompatActivity() {
2 private lateinit var viewModel: UserViewModel
3 private lateinit var nameTextView: TextView
4 private lateinit var ageTextView: TextView
5
6 override fun onCreate(savedInstanceState: Bundle?) {
7 super.onCreate(savedInstanceState)
8 setContentView(R.layout.activity_main)
9
10 viewModel = ViewModelProvider(this).get(UserViewModel::class.java)
11 nameTextView = findViewById(R.id.name_text_view)
12 ageTextView = findViewById(R.id.age_text_view)
13
14 viewModel.user.observe(this, Observer { user ->
15 nameTextView.text = user.name
16 ageTextView.text = user.age.toString()
17 })
18
19 viewModel.fetchUser()
20 }
21}
Dependency Injection
Dependency Injection (DI) is a design pattern that helps manage dependencies between components. It allows you to inject dependencies into a component instead of creating them directly. This makes the code more modular, testable, and maintainable.
Here’s an example of using Dagger 2 for DI:
- Define an interface for a dependency:
1interface ApiService {
2 fun getUser(callback: Callback<User>)
3}
- Implement the interface:
1class RetrofitApiService @Inject constructor() : ApiService {
2 private val api = Retrofit.Builder()
3 .baseUrl("https://example.com/")
4 .build()
5 .create(Api::class.java)
6
7 override fun getUser(callback: Callback<User>) {
8 api.getUser(callback)
9 }
10}
- Define a module for the dependency:
1@Module
2class ApiModule {
3 @Provides
4 fun provideApiService(): ApiService {
5 return RetrofitApiService()
6 }
7}
- Define a component for the module:
1@Component(modules = [ApiModule::class])
2interface AppComponent {
3 fun inject(mainActivity: MainActivity)
4}
- Inject the dependency into the component:
1class MainActivity : AppCompatActivity() {
2 @Inject lateinit var apiService: ApiService
3
4 override fun onCreate(savedInstanceState: Bundle?) {
5 super.onCreate(savedInstanceState)
6 setContentView(R.layout.activity_main)
7
8 (application as
Learn about the AndroidManifest.xml file
The AndroidManifest.xml file is an essential configuration file for every Android app. It provides information about the app, such as its name, package name, permissions, components, and features. The AndroidManifest.xml file is located in the root directory of your app’s project structure.
Here are some of the key elements of the AndroidManifest.xml file:
Package Name
The package name is a unique identifier for your app. It should be in reverse domain name format, such as com.example.myapp.
1<manifest xmlns:android="http://schemas.android.com/apk/res/android"
2 package="com.example.myapp">
3</manifest>
Application
The application element contains information about the application as a whole, such as its name, icon, theme, and backup options.
1<application
2 android:name=".MyApplication"
3 android:icon="@mipmap/ic_launcher"
4 android:label="@string/app_name"
5 android:theme="@style/AppTheme">
6</application>
Activities
The activity element defines an activity and its properties, such as its name, label, and theme.
1<activity
2 android:name=".MainActivity"
3 android:label="@string/app_name">
4 <intent-filter>
5 <action android:name="android.intent.action.MAIN" />
6 <category android:name="android.intent.category.LAUNCHER" />
7 </intent-filter>
8</activity>
Services
The service element defines a service and its properties, such as its name and permissions.
1<service
2 android:name=".MyService"
3 android:exported="false" />
Broadcast Receivers
The receiver element defines a broadcast receiver and its properties, such as its name and permissions.
1<receiver
2 android:name=".MyBroadcastReceiver"
3 android:enabled="true"
4 android:exported="false">
5 <intent-filter>
6 <action android:name="android.intent.action.BOOT_COMPLETED" />
7 </intent-filter>
8</receiver>
Content Providers
The provider element defines a content provider and its properties, such as its name, authorities, and permissions.
1<provider
2 android:name=".MyContentProvider"
3 android:authorities="com.example.myapp.MyContentProvider"
4 android:exported="false"
5 android:grantUriPermissions="true" />
Permissions
The uses-permission element defines the permissions that your app requires to access certain features or services.
1<uses-permission android:name="android.permission.INTERNET" />
2<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
3<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
Features
The uses-feature element defines the features that your app requires to run, such as a camera or GPS.
1<uses-feature android:name="android.hardware.camera" />
2<uses-feature android:name="android.hardware.location.gps" />
These are just a few of the key elements of the AndroidManifest.xml file. You can find more detailed information and best practices in the Android documentation.
Create a WebView App
Create a new project and add a WebView to the main activity
The issue with the original code is that the findViewById()
method is called before setContentView()
. This results in a NullPointerException
because the layout has not been inflated yet.
To fix the issue, simply move the findViewById()
call after the setContentView()
method in the onCreate()
method of the MainActivity.java
file:
1@Override
2protected void onCreate(Bundle savedInstanceState) {
3 super.onCreate(savedInstanceState);
4 setContentView(R.layout.activity_main);
5
6 mWebView = (WebView) findViewById(R.id.activity_main_webview);
7 WebSettings webSettings = mWebView.getSettings();
8 webSettings.setJavaScriptEnabled(true);
9 mWebView.loadUrl("http://beta.html5test.com/");
10}
Additionally, ensure that the INTERNET
permission is declared before the <application>
tag in the AndroidManifest.xml
file:
1<uses-permission android:name="android.permission.INTERNET" />
2<application
3 android:allowBackup="true"
4 android:icon="@drawable/ic_launcher"
5 android:label="@string/app_name"
6 android:theme="@style/AppTheme" >
7 <!-- ... -->
8</application>
With these changes, the WebView should load the specified URL correctly.
Load a website in the WebView
The solution provided in the post you found is correct. The issue with the original code is that the WebView
is not properly set up to handle URL loading within the app itself. By setting a custom WebViewClient
for the WebView
, you can ensure that URLs are loaded within the WebView
instead of launching the web browser.
Here’s the corrected code for your Main
activity:
1import android.app.Activity;
2import android.os.Bundle;
3import android.webkit.WebResourceError;
4import android.webkit.WebResourceRequest;
5import android.webkit.WebView;
6import android.webkit.WebViewClient;
7import android.widget.Toast;
8import android.annotation.TargetApi;
9
10public class Main extends Activity {
11
12 private WebView mWebview;
13
14 @Override
15 public void onCreate(Bundle savedInstanceState) {
16
17 super.onCreate(savedInstanceState);
18
19 mWebview = new WebView(this);
20
21 mWebview.getSettings().setJavaScriptEnabled(true); // enable javascript
22
23 final Activity activity = this;
24
25 mWebview.setWebViewClient(new WebViewClient() {
26 @SuppressWarnings("deprecation")
27 @Override
28 public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) {
29 Toast.makeText(activity, description, Toast.LENGTH_SHORT).show();
30 }
31 @TargetApi(android.os.Build.VERSION_CODES.M)
32 @Override
33 public void onReceivedError(WebView view, WebResourceRequest req, WebResourceError rerr) {
34 // Redirect to deprecated method, so you can use it in all SDK versions
35 onReceivedError(view, rerr.getErrorCode(), rerr.getDescription().toString(), req.getUrl().toString());
36 }
37 });
38
39 mWebview .loadUrl("http://www.google.com");
40 setContentView(mWebview );
41
42 }
43
44}
Additionally, you should create a separate XML layout file for the WebView
, as shown in the post:
1<?xml version="1.0" encoding="utf-8"?>
2<WebView xmlns:android="http://schemas.android.com/apk/res/android"
3 android:id="@+id/help_webview"
4 android:layout_width="fill_parent"
5 android:layout_height="fill_parent"
6 android:scrollbars="none"
7/>
Then, in your activity, inflate this layout and find the WebView
by its ID:
1public class Main extends Activity {
2
3 private WebView mWebview;
4
5 @Override
6 public void onCreate(Bundle savedInstanceState) {
7
8 super.onCreate(savedInstanceState);
9
10 setContentView(R.layout.webviewlayout);
11
12 mWebview = findViewById(R.id.help_webview);
13
14 mWebview.getSettings().setJavaScriptEnabled(true); // enable javascript
15
16 final Activity activity = this;
17
18 mWebview.setWebViewClient(new WebViewClient() {
19 @SuppressWarnings("deprecation")
20 @Override
21 public void onReceivedError(WebView view, int errorCode, String description, String failingUrl) {
22 Toast.makeText(activity, description, Toast.LENGTH_SHORT).show();
23 }
24 @TargetApi(android.os.Build.VERSION_CODES.M)
25 @Override
26 public void onReceivedError(WebView view, WebResourceRequest req, WebResourceError rerr) {
27 // Redirect to deprecated method, so you can use it in all SDK versions
28 onReceivedError(view, rerr.getErrorCode(), rerr.getDescription().toString(), req.getUrl().toString());
29 }
30 });
31
32 mWebview .loadUrl("http://www.google.com");
33
34 }
35
36}
This should properly load the website within the WebView
in your Android app.
Handle WebView events
Handling WebView events in Android involves setting up listeners for various events that occur during the loading and interaction with web content. Here are some common WebView events and how to handle them:
- WebViewClient: This is the primary interface for controlling the WebView’s interaction with web content. You can override methods like
onPageStarted
,onPageFinished
,onLoadResource
, andonReceivedError
to handle various events during the loading process.
Example:
1mWebview.setWebViewClient(new WebViewClient() {
2 @Override
3 public void onPageStarted(WebView view, String url, Bitmap favicon) {
4 super.onPageStarted(view, url, favicon);
5 // Handle page start event
6 }
7
8 @Override
9 public void onPageFinished(WebView view, String url) {
10 super.onPageFinished(view, url);
11 // Handle page finished event
12 }
13
14 @Override
15 public void onLoadResource(WebView view, String url) {
16 super.onLoadResource(view, url);
17 // Handle resource loading event
18 }
19
20 @Override
21 public void onReceivedError(WebView view, WebResourceRequest request, WebResourceError error) {
22 super.onReceivedError(view, request, error);
23 // Handle loading error event
24 }
25});
- WebChromeClient: This interface provides additional functionality for WebView, such as handling JavaScript alerts, confirmations, and prompts, as well as managing progress and uploading files.
Example:
1mWebview.setWebChromeClient(new WebChromeClient() {
2 @Override
3 public boolean onJsAlert(WebView view, String url, String message, JsResult result) {
4 // Handle JavaScript alert event
5 return super.onJsAlert(view, url, message, result);
6 }
7
8 @Override
9 public boolean onJsConfirm(WebView view, String url, String message, JsResult result) {
10 // Handle JavaScript confirmation event
11 return super.onJsConfirm(view, url, message, result);
12 }
13
14 @Override
15 public boolean onJsPrompt(WebView view, String url, String message, String defaultValue, JsPromptResult result) {
16 // Handle JavaScript prompt event
17 return super.onJsPrompt(view, url, message, defaultValue, result);
18 }
19
20 @Override
21 public void onProgressChanged(WebView view, int newProgress) {
22 super.onProgressChanged(view, newProgress);
23 // Handle progress change event
24 }
25});
- OnTouchListener: You can set an OnTouchListener on the WebView to handle touch events. This can be useful for implementing custom gestures or handling user interactions.
Example:
1mWebview.setOnTouchListener(new View.OnTouchListener() {
2 @Override
3 public boolean onTouch(View v, MotionEvent event) {
4 // Handle touch event
5 return false;
6 }
7});
These are just a few examples of handling WebView events in Android. You can find more information on WebView events and other related topics in the Android documentation.
Create a RESTful API Client
Learn about RESTful APIs and how to consume them in Android
RESTful APIs (Representational State Transfer) are a popular way to build web services that allow clients to interact with resources over the HTTP protocol. In Android, you can consume RESTful APIs using various libraries and techniques. Here’s a brief overview of how to consume RESTful APIs in Android:
- Choose a library: There are several libraries available for consuming RESTful APIs in Android, such as Retrofit, Volley, and OkHttp. Retrofit is a popular choice due to its simplicity and ease of use.
- Define the API interface: Define an interface that describes the API endpoints and methods. Each method should return a type that corresponds to the expected response format, such as
Call<ResponseBody>
orCall<List<MyModel>>
.
Example:
1public interface MyApi {
2 @GET("users")
3 Call<List<User>> getUsers();
4
5 @POST("users")
6 Call<User> createUser(@Body User user);
7}
- Create a Retrofit instance: Create a Retrofit instance with the base URL of the API and any necessary configuration options.
Example:
1Retrofit retrofit = new Retrofit.Builder()
2 .baseUrl("https://myapi.com/")
3 .build();
- Create an API instance: Use the Retrofit instance to create an instance of the API interface.
Example:
1MyApi api = retrofit.create(MyApi.class);
- Make API calls: Use the API instance to make API calls and handle the responses.
Example:
1Call<List<User>> call = api.getUsers();
2call.enqueue(new Callback<List<User>>() {
3 @Override
4 public void onResponse(Call<List<User>> call, Response<List<User>> response) {
5 if (response.isSuccessful()) {
6 List<User> users = response.body();
7 // Handle successful response
8 } else {
9 // Handle error response
10 }
11 }
12
13 @Override
14 public void onFailure(Call<List<User>> call, Throwable t) {
15 // Handle network error
16 }
17});
- Error handling: Make sure to handle errors and exceptions that may occur during API calls, such as network errors, parsing errors, and server errors.
- Caching and offline support: Consider implementing caching and offline support to improve the user experience and reduce network usage.
These are just a few basic steps for consuming RESTful APIs in Android. You can find more detailed information and best practices in the Android documentation and various online resources.
Use Retrofit or Volley to make API calls
Retrofit and Volley are two popular libraries for making API calls in Android. Here’s a brief overview of how to use each library to make API calls:
Retrofit
- Add Retrofit dependency: Add the Retrofit dependency to your
build.gradle
file:
1implementation 'com.squareup.retrofit2:retrofit:2.9.0'
2implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
- Define the API interface: Define an interface that describes the API endpoints and methods. Each method should return a type that corresponds to the expected response format, such as
Call<ResponseBody>
orCall<List<MyModel>>
.
Example:
1public interface MyApi {
2 @GET("users")
3 Call<List<User>> getUsers();
4
5 @POST("users")
6 Call<User> createUser(@Body User user);
7}
Handle API responses and errors
To handle API responses and errors in Android using Retrofit, you can create a custom Callback class that invokes your APIs for success and failure based on your domain logic. Here’s an example implementation:
- Create a custom abstract class called
CustomCallback
that extendsCallback<T>
:
1abstract class CustomCallback<T> implements Callback<T> {
2
3 abstract void onSuccess(T response);
4 abstract void onFailure(Throwable throwable);
5
6 @Override
7 public void onResponse(Call<T> call, Response<T> response) {
8 if (response.isSuccessful()) {
9 onSuccess(response.body());
10 } else {
11 onFailure(new HttpException(response));
12 }
13 }
14
15 @Override
16 public void onFailure(Call<T> call, Throwable t) {
17 onFailure(t);
18 }
19}
- Modify your
ApiManager
class to use theCustomCallback
class:
1public class ApiManager {
2
3 private static ApiManager apiManager;
4
5 public static ApiManager getInstance(Context context) {
6 if (apiManager == null) {
7 apiManager = new ApiManager(context);
8 }
9 return apiManager;
10 }
11
12 private ApiManager(Context context) {
13 this.context = context;
14 apiInterface = ApiClient.getClient(context).create(ApiInterface.class);
15 }
16
17 public void getDevices(CustomCallback<ResponseBody> callback) {
18 apiInterface.getDevices(getAuthHeader()).enqueue(callback);
19 }
20
21 // Other API implementation
22}
- Use the
CustomCallback
class in your activities or fragments:
1ApiManager.getInstance(this).getDevices(new CustomCallback<ResponseBody>() {
2 @Override
3 void onSuccess(ResponseBody response) {
4 // Handle successful response
5 }
6
7 @Override
8 void onFailure(Throwable throwable) {
9 // Handle failure response
10 }
11});
This implementation allows you to handle success and failure responses in a centralized way, reducing boilerplate code and making it easier to manage UI side-effects.
Regarding your questions about handling 4xx, 5xx responses globally, you can use an interceptor in the ApiClient
class. To make an asynchronous call synchronous, you can use a CountDownLatch
to wait for the result. However, this is not recommended as it may block the UI thread and cause performance issues. Instead, consider using a callback or a library like RxJava to handle asynchronous calls.
For the Authenticator implementation, you can use the Authenticator
class provided by Retrofit. It allows you to handle authentication challenges and refresh tokens as needed. You can use a synchronous call to refresh the token, but it’s recommended to use an asynchronous call with a callback to avoid blocking the UI thread.
Create a Bioinformatics Tools App
Create a new project and design the app layout
To create a new Android project and design the app layout, follow these steps:
- Open Android Studio and create a new project. Choose “Empty Activity” as the project template.
- Open the
activity_main.xml
file in theres/layout
directory. - Replace the existing layout with a
ConstraintLayout
as the root layout. - Add a
TextView
to display the title of the app. Set its properties as follows:
1<TextView
2 android:id="@+id/titleTextView"
3 android:layout_width="wrap_content"
4 android:layout_height="wrap_content"
5 android:text="@string/app_name"
6 android:textSize="24sp"
7 android:textStyle="bold"
8 app:layout_constraintEnd_toEndOf="parent"
9 app:layout_constraintStart_toStartOf="parent"
10 app:layout_constraintTop_toTopOf="parent" />
- Add a
RecyclerView
to display the list of items. Set its properties as follows:
1<androidx.recyclerview.widget.RecyclerView
2 android:id="@+id/itemsRecyclerView"
3 android:layout_width="match_parent"
4 android:layout_height="0dp"
5 app:layout_constraintBottom_toBottomOf="parent"
6 app:layout_constraintEnd_toEndOf="parent"
7 app:layout_constraintStart_toStartOf="parent"
8 app:layout_constraintTop_toBottomOf="@id/titleTextView" />
- Add a
FloatingActionButton
to add new items. Set its properties as follows:
1<com.google.android.material.floatingactionbutton.FloatingActionButton
2 android:id="@+id/addButton"
3 android:layout_width="wrap_content"
4 android:layout_height="wrap_content"
5 android:layout_margin="16dp"
6 android:contentDescription="@string/add_button_content_description"
7 android:src="@drawable/ic_add"
8 app:layout_constraintBottom_toBottomOf="parent"
9 app:layout_constraintEnd_toEndOf="parent" />
- Add a
String
resource for thecontentDescription
attribute of theFloatingActionButton
in thestrings.xml
file:
1<string name="add_button_content_description">Add new item</string>
- Add a
Drawable
resource for thesrc
attribute of theFloatingActionButton
in theic_add.xml
file:
1<vector xmlns:android="http://schemas.android.com/apk/res/android"
2 android:width="24dp"
3 android:height="24dp"
4 android:viewportWidth="24"
5 android:viewportHeight="24"
6 android:tint="?attr/colorOnPrimary">
7 <path
8 android:fillColor="@android:color/white"
9 android:pathData="M19,13h-6v6h-2v-6H5v-2h6V5h2v6h6v2z"/>
10</vector>
- Add a
LinearLayoutManager
to theRecyclerView
in theMainActivity.java
file:
1itemsRecyclerView.setLayoutManager(new LinearLayoutManager(this));
- Add a
ClickListener
to theFloatingActionButton
in theMainActivity.java
file:
1addButton.setOnClickListener(new View.OnClickListener() {
2 @Override
3 public void onClick(View v) {
4 // Handle add button click
5 }
6});
This is a basic layout design for an Android app that displays a list of items with a title and an add button. You can customize the layout further based on your requirements.
Integrate the bioinformatics tools using RESTful APIs
Sources: journals.plos.org (1) ncbi.nlm.nih.gov (2) academic.oup.com (3) researchgate.net (4)
Based on the search results you provided, it seems that the article discusses best practices for building RESTful APIs for life sciences resources. While the article does not specifically mention Android, the principles for consuming RESTful APIs in Android can still be applied.
To integrate bioinformatics tools using RESTful APIs in Android, you can follow these steps:
- Choose a bioinformatics tool that provides a RESTful API. For example, you could use the Proteins API mentioned in the article.
- Determine the functionalities you want to provide in your Android app. This could include searching for proteins, retrieving protein information, etc.
- Use Retrofit or Volley to make API calls to the bioinformatics tool’s RESTful API.
- Handle API responses and errors using a custom Callback class, as described in my previous response.
- Design the app layout to display the data retrieved from the bioinformatics tool’s API. This could include a ListView or RecyclerView to display search results, and TextViews or ImageViews to display protein information.
- Implement the necessary functionality to interact with the bioinformatics tool’s API. For example, you could implement a search bar that sends a GET request to the API’s search endpoint when the user submits a query.
- Test your app to ensure that it is functioning correctly and providing accurate data from the bioinformatics tool’s API.
By following these steps, you can integrate bioinformatics tools using RESTful APIs in Android.
Display the results in a user-friendly format
Sources: ncbi.nlm.nih.gov (1) academic.oup.com (2) bioinformatics.org (3) github.com (4)
To display the results of the bioinformatics tools API in a user-friendly format on Android, you can follow these steps:
- Create a new Android project and design the app layout as described in my previous response.
- Use Retrofit or Volley to make API calls to the bioinformatics tool’s RESTful API, as described in my previous response.
- Handle API responses and errors using a custom Callback class, as described in my previous response.
- Parse the API response and extract the relevant data.
- Display the data in a user-friendly format using Android UI components.
For example, if the API response is a JSON object containing genetic information about the user, you can parse the JSON object and display the information in a ListView or RecyclerView. You can also use charts and graphs to visualize the data in a more engaging way.
Here’s an example of how to parse a JSON object and display the data in a ListView:
- Create a model class to represent the data:
1public class GeneticData {
2 private String geneName;
3 private String riskLevel;
4
5 public GeneticData(String geneName, String riskLevel) {
6 this.geneName = geneName;
7 this.riskLevel = riskLevel;
8 }
9
10 public String getGeneName() {
11 return geneName;
12 }
13
14 public String getRiskLevel() {
15 return riskLevel;
16 }
17}
- Parse the JSON object and create a list of
GeneticData
objects:
1List<GeneticData> geneticDataList = new ArrayList<>();
2JSONObject jsonObject = new JSONObject(apiResponse);
3JSONArray genesArray = jsonObject.getJSONArray("genes");
4for (int i = 0; i < genesArray.length(); i++) {
5 JSONObject geneObject = genesArray.getJSONObject(i);
6 String geneName = geneObject.getString("name");
7 String riskLevel = geneObject.getString("risk_level");
8 geneticDataList.add(new GeneticData(geneName, riskLevel));
9}
- Create an adapter for the ListView:
1public class GeneticDataAdapter extends ArrayAdapter<GeneticData> {
2 public GeneticDataAdapter(Context context, List<GeneticData> geneticDataList) {
3 super(context, 0, geneticDataList);
4 }
5
6 @NonNull
7 @Override
8 public View getView(int position, View convertView, ViewGroup parent) {
9 View listItemView = convertView;
10 if (listItemView == null) {
11 listItemView = LayoutInflater.from(getContext()).inflate(R.layout.list_item, parent, false);
12 }
13
14 GeneticData currentGeneticData = getItem(position);
15
16 TextView geneNameTextView = listItemView.findViewById(R.id.gene_name_text_view);
17 geneNameTextView.setText(currentGeneticData.getGeneName());
18
19 TextView riskLevelTextView = listItemView.findViewById(R.id.risk_level_text_view);
20 riskLevelTextView.setText(currentGeneticData.getRiskLevel());
21
22 return listItemView;
23 }
24}
- Set the adapter for the ListView:
1ListView geneticDataListView = findViewById(R.id.genetic_data_list_view);
2geneticDataListView.setAdapter(new GeneticDataAdapter(this, geneticDataList));
This is just an example of how to display genetic data in a user-friendly format on Android. You can customize the UI and the data representation based on your specific requirements.
Data Persistence
Data persistence in Android refers to the process of storing data on the device so that it can be accessed and used even when the app is not running. There are several ways to persist data in Android, including:
- SharedPreferences: This is a simple way to store small amounts of data as key-value pairs. It is useful for storing user preferences or settings.
Example:
1SharedPreferences sharedPreferences = PreferenceManager.getDefaultSharedPreferences(this);
2SharedPreferences.Editor editor = sharedPreferences.edit();
3editor.putString("username", "john_doe");
4editor.apply();
- SQLite Database: This is a relational database that is integrated with Android. It is useful for storing large amounts of structured data.
Example:
1SQLiteDatabase db = openOrCreateDatabase("mydatabase", Context.MODE_PRIVATE, null);
2db.execSQL("CREATE TABLE IF NOT EXISTS users (username TEXT, password TEXT)");
3db.execSQL("INSERT INTO users (username, password) VALUES ('john_doe', 'password123')");
- File Storage: This is a way to store data as files on the device. It is useful for storing large amounts of unstructured data.
Example:
1File file = new File(getExternalFilesDir(null), "myfile.txt");
2FileOutputStream fos = new FileOutputStream(file);
3fos.write("Hello, World!".getBytes());
4fos.close();
- Room: This is a library provided by Android that simplifies the process of working with SQLite databases. It provides an object-oriented interface for defining database schemas and performing database operations.
Example:
1@Entity
2public class User {
3 @PrimaryKey
4 private int id;
5 private String username;
6 private String password;
7
8 // Getters and setters
9}
10
11@Dao
12public interface UserDao {
13 @Insert
14 void insert(User user);
15
16 @Query("SELECT * FROM user")
17 List<User> getAll();
18}
19
20@Database(entities = {User.class}, version = 1)
21public abstract class AppDatabase extends RoomDatabase {
22 public abstract UserDao userDao();
23}
24
25AppDatabase db = Room.databaseBuilder(this, AppDatabase.class, "mydatabase").build();
26User user = new User(1, "john_doe", "password123");
27db.userDao().insert(user);
28List<User> users = db.userDao().getAll();
- Realm: This is a mobile database that is an alternative to SQLite. It provides an object-oriented interface for defining database schemas and performing database operations.
Example:
1Realm.init(this);
2Realm realm = Realm.getDefaultInstance();
3
4realm.executeTransaction(new Realm.Transaction() {
5 @Override
6 public void execute(Realm realm) {
7 User user = realm.createObject(User.class);
8 user.setUsername("john_doe");
9 user.setPassword("password123");
10 }
11});
12
13RealmResults<User> users = realm.where(User.class).findAll();
These are just a few examples of how to persist data in Android. You can choose the appropriate data persistence method based on your specific requirements.
Learn about SQLite databases and how to use them in Android
SQLite is a relational database management system that is integrated with Android. It is useful for storing large amounts of structured data. Here’s how to use SQLite databases in Android:
- Create a database helper class that extends
SQLiteOpenHelper
:
1public class MyDatabaseHelper extends SQLiteOpenHelper {
2 public static final String DATABASE_NAME = "mydatabase.db";
3 public static final int DATABASE_VERSION = 1;
4
5 public MyDatabaseHelper(Context context) {
6 super(context, DATABASE_NAME, null, DATABASE_VERSION);
7 }
8
9 @Override
10 public void onCreate(SQLiteDatabase db) {
11 String SQL_CREATE_ENTRIES = "CREATE TABLE " + MyContract.MyEntry.TABLE_NAME + " ("
12 + MyContract.MyEntry._ID + " INTEGER PRIMARY KEY,"
13 + MyContract.MyEntry.COLUMN_NAME + " TEXT,"
14 + MyContract.MyEntry.COLUMN_VALUE + " INTEGER)";
15 db.execSQL(SQL_CREATE_ENTRIES);
16 }
17
18 @Override
19 public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
20 String SQL_DELETE_ENTRIES = "DROP TABLE IF EXISTS " + MyContract.MyEntry.TABLE_NAME;
21 db.execSQL(SQL_DELETE_ENTRIES);
22 onCreate(db);
23 }
24}
- Define a contract class that contains the database schema:
1public final class MyContract {
2 public static abstract class MyEntry implements BaseColumns {
3 public static final String TABLE_NAME = "mytable";
4 public static final String COLUMN_NAME = "name";
5 public static final String COLUMN_VALUE = "value";
6 }
7}
- Use the database helper class to perform database operations:
1MyDatabaseHelper dbHelper = new MyDatabaseHelper(this);
2SQLiteDatabase db = dbHelper.getWritableDatabase();
3
4ContentValues values = new ContentValues();
5values.put(MyContract.MyEntry.COLUMN_NAME, "example_name");
6values.put(MyContract.MyEntry.COLUMN_VALUE, 123);
7db.insert(MyContract.MyEntry.TABLE_NAME, null, values);
8
9String selection = MyContract.MyEntry.COLUMN_NAME + " = ?";
10String[] selectionArgs = {"example_name"};
11Cursor cursor = db.query(
12 MyContract.MyEntry.TABLE_NAME,
13 null,
14 selection,
15 selectionArgs,
16 null,
17 null,
18 null
19);
20
21if (cursor != null) {
22 cursor.moveToFirst();
23 int nameColumnIndex = cursor.getColumnIndex(MyContract.MyEntry.COLUMN_NAME);
24 int valueColumnIndex = cursor.getColumnIndex(MyContract.MyEntry.COLUMN_VALUE);
25 String name = cursor.getString(nameColumnIndex);
26 int value = cursor.getInt(valueColumnIndex);
27 Log.d("MyDatabase", "Name: " + name + ", Value: " + value);
28 cursor.close();
29}
30
31db.close();
32dbHelper.close();
This is just a simple example of how to use SQLite databases in Android. You can customize the database schema and operations based on your specific requirements.
Note: It is recommended to use Room library instead of SQLiteOpenHelper for new projects, as it provides a more convenient and safer way to work with SQLite databases in Android.
Implement data persistence in the bioinformatics tools app
Sources: academic.oup.com (1) ncbi.nlm.nih.gov (2) academic.oup.com (3) rna-seqblog.com (4)
To implement data persistence in the bioinformatics tools app for Android, you can use SQLite databases to store and manage the data generated by the app. Here are the steps to implement data persistence using SQLite databases:
- Create a new database helper class that extends
SQLiteOpenHelper
and override theonCreate()
andonUpgrade()
methods to create and upgrade the database schema. - Define the database schema by creating a class that implements the
SQLiteOpenHelper
interface and specifying the database name, version, and theonCreate()
andonUpgrade()
methods. - Create a data access object (DAO) class to manage the CRUD (Create, Read, Update, Delete) operations on the database.
- Use the DAO class to perform database operations such as inserting, querying, updating, and deleting data.
- Use the
SQLiteDatabase
object to execute SQL queries and transactions. - Close the database connection when it is no longer needed to release system resources.
Here is an example of how to create a SQLite database and perform CRUD operations in Android:
- Create a new database helper class:
1public class MyDatabaseHelper extends SQLiteOpenHelper {
2 public static final String DATABASE_NAME = "mydatabase.db";
3 public static final int DATABASE_VERSION = 1;
4
5 public MyDatabaseHelper(Context context) {
6 super(context, DATABASE_NAME, null, DATABASE_VERSION);
7 }
8
9 @Override
10 public void onCreate(SQLiteDatabase db) {
11 String SQL_CREATE_ENTRIES = "CREATE TABLE " + MyContract.MyEntry.TABLE_NAME + " ("
12 + MyContract.MyEntry._ID + " INTEGER PRIMARY KEY,"
13 + MyContract.MyEntry.COLUMN_NAME + " TEXT,"
14 + MyContract.MyEntry.COLUMN_VALUE + " INTEGER)";
15 db.execSQL(SQL_CREATE_ENTRIES);
16 }
17
18 @Override
19 public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
20 String SQL_DELETE_ENTRIES = "DROP TABLE IF EXISTS " + MyContract.MyEntry.TABLE_NAME;
21 db.execSQL(SQL_DELETE_ENTRIES);
22 onCreate(db);
23 }
24}
- Define the database schema:
1public final class MyContract {
2 public static abstract class MyEntry implements BaseColumns {
3 public static final String TABLE_NAME = "mytable";
4 public static final String COLUMN_NAME = "name";
5 public static final String COLUMN_VALUE = "value";
6 }
7}
- Create a DAO class:
1public class MyDao {
2 private SQLiteDatabase db;
3 private MyDatabaseHelper dbHelper;
4
5 public MyDao(Context context) {
6 dbHelper = new MyDatabaseHelper(context);
7 }
8
9 public void open() {
10 db = dbHelper.getWritableDatabase();
11 }
12
13 public void close() {
14 if (db != null) {
15 db.close();
16 dbHelper.close();
17 }
18 }
19
20 public void insert(String name, int value) {
21 ContentValues values = new ContentValues();
22 values.put(MyContract.MyEntry.COLUMN_NAME, name);
23 values.put(MyContract.MyEntry.COLUMN_VALUE, value);
24 db.insert(MyContract.MyEntry.TABLE_NAME, null, values);
25 }
26
27 public Cursor query(String name) {
28 String selection = MyContract.MyEntry.COLUMN_NAME + " = ?";
29 String[] selectionArgs = {name};
30 return db.query(
31 MyContract.MyEntry.TABLE_NAME,
32 null,
33 selection,
34 selectionArgs,
35 null,
36 null,
37 null
38 );
39 }
40
41 public int update(String name, int value) {
42 ContentValues values = new ContentValues();
43 values.put(MyContract.MyEntry.COLUMN_NAME, name);
44 values.put(MyContract.MyEntry.COLUMN_VALUE, value);
45 String selection =
UI/UX Best Practices
Learn about Material Design and how to implement it in Android
Material Design is a design language developed by Google that provides a consistent and cohesive design system for digital products. It emphasizes simplicity, usability, and consistency, and provides a set of guidelines and components for creating visually appealing and user-friendly interfaces.
To implement Material Design in Android, you can use the Material Components for Android library, which provides a set of pre-built UI components that follow the Material Design guidelines. Here are the steps to implement Material Design in Android:
- Add the Material Components for Android library to your project by adding the following line to your app-level build.gradle file:
1implementation 'com.google.android.material:material:1.4.0'
- Use the Material Components in your layout XML files by replacing the standard Android views with their Material Design equivalents, such as
MaterialButton
instead ofButton
,MaterialCardView
instead ofCardView
, andMaterialTextView
instead ofTextView
. - Customize the Material Components by setting their attributes, such as
app:cornerRadius
,app:elevation
,app:backgroundTint
, andapp:textAppearance
. - Use the Material Design themes and styles to apply a consistent look and feel to your app. You can apply a Material Design theme to your entire app by setting the
android:theme
attribute in your app’s style.xml file, or you can apply a theme to individual views by setting theandroid:theme
attribute in your layout XML files. - Use the Material Design guidelines to create a consistent and cohesive user interface, such as using the correct spacing, typography, and color schemes.
Here is an example of how to use Material Components in Android:
- Add the Material Components for Android library to your project:
1implementation 'com.google.android.material:material:1.4.0'
- Use the Material Components in your layout XML files:
1<com.google.android.material.button.MaterialButton
2 android:id="@+id/myButton"
3 android:layout_width="wrap_content"
4 android:layout_height="wrap_content"
5 android:text="@string/my_button_text"
6 app:cornerRadius="4dp"
7 app:elevation="2dp"
8 app:backgroundTint="@color/colorPrimary"
9 app:textAppearance="@style/TextAppearance.MaterialComponents.Button" />
10
11<com.google.android.material.card.MaterialCardView
12 android:layout_width="match_parent"
13 android:layout_height="wrap_content"
14 android:layout_margin="16dp"
15 app:cardCornerRadius="4dp"
16 app:cardElevation="2dp"
17 app:contentPadding="16dp">
18
19 <com.google.android.material.textview.MaterialTextView
20 android:layout_width="wrap_content"
21 android:layout_height="wrap_content"
22 android:text="@string/my_text"
23 app:textAppearance="@style/TextAppearance.MaterialComponents.Body1" />
24
25</com.google.android.material.card.MaterialCardView>
- Customize the Material Components by setting their attributes:
1<com.google.android.material.button.MaterialButton
2 android:id="@+id/myButton"
3 android:layout_width="wrap_content"
4 android:layout_height="wrap_content"
5 android:text="@string/my_button_text"
6 app:cornerRadius="4dp"
7 app:elevation="2dp"
8 app:backgroundTint="@color/colorPrimary"
9 app:textAppearance="@style/TextAppearance.MaterialComponents.Button" />
- Use the Material Design themes and styles:
1<style name="AppTheme" parent="Theme.MaterialComponents.Light.NoActionBar">
2 <item name="colorPrimary">@color/colorPrimary</item>
3 <item name="colorPrimaryVariant">@color/colorPrimaryVariant</item>
4 <item name="colorOnPrimary">@color/colorOnPrimary</item>
5 <item name="colorSecondary">@color/colorSecondary</item>
6 <item name="colorSecondaryVariant
Follow best practices for designing a user-friendly app
To create a user-friendly app in Android, follow these best practices:
Use the Material Design guidelines:
- Follow the Material Design guidelines for spacing, typography, and color schemes.
- Use the Material Components for Android library to implement the Material Design guidelines.
Provide clear and concise information:
- Use clear and concise labels for your UI elements.
- Use appropriate icons and images to enhance the user experience.
Ensure consistency and coherence:
- Use a consistent color scheme and typography throughout your app.
- Use a consistent layout and spacing for your UI elements.
Use appropriate gestures and interactions:
- Use gestures and interactions that are familiar to users, such as swipe, tap, and drag.
- Provide feedback to users when they interact with your app, such as visual cues, sound effects, and vibration.
Optimize for different screen sizes and orientations:
- Ensure that your app looks and functions well on different screen sizes and orientations.
- Use the ConstraintLayout or other responsive layouts to create flexible and adaptable UI designs.
Ensure accessibility and usability:
- Follow the Android accessibility guidelines to make your app usable by as many users as possible.
- Provide alternative text for images and other non-text content.
- Ensure that your app can be used with a keyboard and other assistive technologies.
Test your app on different devices and Android versions:
- Test your app on a variety of devices and Android versions to ensure compatibility and performance.
- Use the Android Studio emulator and other testing tools to simulate different devices and Android versions.
By following these best practices, you can create a user-friendly and visually appealing app in Android that provides a consistent and cohesive user experience.
Testing and Debugging
Learn about the different testing tools available in Android Studio
Android Studio provides several testing tools to help you test your Android app. Here are some of the testing tools available in Android Studio:
- Android JUnit Test: This is a testing framework for unit testing your app’s code. It allows you to write and run unit tests for your app’s Java and Kotlin code.
- Espresso: This is a testing framework for UI testing your app. It allows you to write and run UI tests for your app’s user interface.
- UI Automator: This is a testing framework for UI testing your app. It allows you to write and run UI tests for your app’s user interface using a more flexible and expressive API than Espresso.
- Robolectric: This is a testing framework for unit testing your app’s code. It allows you to write and run unit tests for your app’s Java and Kotlin code without launching an emulator or device.
- Mockito: This is a mocking framework for unit testing your app’s code. It allows you to create mock objects for your app’s dependencies and test your code in isolation.
- Firebase Test Lab: This is a cloud-based testing service for your app. It allows you to test your app on a variety of devices and configurations, and provides detailed reports and logs.
- Layout Inspector: This is a tool for inspecting and debugging your app’s layout. It allows you to view the layout hierarchy, measure and style information, and inspect the properties of UI elements.
- Android Profiler: This is a tool for profiling your app’s performance. It allows you to monitor your app’s CPU, memory, network, and disk usage in real-time.
- Device File Explorer: This is a tool for browsing and managing the files on your connected device or emulator. It allows you to view, copy, and delete files on your device or emulator.
These are just a few of the testing tools available in Android Studio. You can choose the appropriate testing tool based on your specific requirements.
Note: It is recommended to use a combination of unit tests, UI tests, and profiling tools to ensure the quality and performance of your app.
Write unit tests and UI tests
To write unit tests and UI tests for an Android app, you can use the following tools and frameworks:
- Android JUnit Test: This is a testing framework for unit testing your app’s code. It allows you to write and run unit tests for your app’s Java and Kotlin code.
Example of a unit test using JUnit:
1import org.junit.Test;
2import static org.junit.Assert.assertEquals;
3
4public class ExampleUnitTest {
5 @Test
6 public void testAddition() {
7 int result = Calculator.add(2, 3);
8 assertEquals(5, result);
9 }
10}
- Espresso: This is a testing framework for UI testing your app. It allows you to write and run UI tests for your app’s user interface.
Example of a UI test using Espresso:
1import androidx.test.espresso.Espresso.onView;
2import androidx.test.espresso.action.ViewActions.click;
3import androidx.test.espresso.assertion.ViewAssertions.matches;
4import androidx.test.espresso.matcher.ViewMatchers.withId;
5import androidx.test.ext.junit.rules.ActivityTestRule;
6import androidx.test.filters.LargeTest;
7import androidx.test.runner.AndroidJUnit4;
8
9import org.junit.Rule;
10import org.junit.Test;
11import org.junit.runner.RunWith;
12
13@RunWith(AndroidJUnit4.class)
14@LargeTest
15public class ExampleEspressoTest {
16 @Rule
17 public ActivityTestRule<MainActivity> activityRule = new ActivityTestRule<>(MainActivity.class);
18
19 @Test
20 public void testClickButton() {
21 onView(withId(R.id.button)).perform(click());
22 onView(withId(R.id.textView)).check(matches(withText("Hello, World!"));
23 }
24}
- UI Automator: This is a testing framework for UI testing your app. It allows you to write and run UI tests for your app’s user interface using a more flexible and expressive API than Espresso.
Example of a UI test using UI Automator:
1import androidx.test.uiautomator.UiDevice;
2import androidx.test.uiautomator.UiObject;
3import androidx.test.uiautomator.UiSelector;
4import androidx.test.uiautomator.Until;
5import androidx.test.filters.LargeTest;
6import androidx.test.rule.ActivityTestRule;
7import androidx.test.runner.AndroidJUnit4;
8
9import org.junit.Rule;
10import org.junit.Test;
11import org.junit.runner.RunWith;
12
13@RunWith(AndroidJUnit4.class)
14@LargeTest
15public class ExampleUIAutomatorTest {
16 @Rule
17 public ActivityTestRule<MainActivity> activityRule = new ActivityTestRule<>(MainActivity.class);
18
19 @Test
20 public void testClickButton() {
21 UiDevice device = UiDevice.getInstance(InstrumentationRegistry.getInstrumentation());
22 UiObject button = device.findObject(new UiSelector().text("Button"));
23 button.click();
24 UiObject textView = device.findObject(new UiSelector().text("Hello, World!"));
25 textView.waitForExists(Until.hasObject(button));
26 }
27}
These are just a few examples of how to write unit tests and UI tests for an Android app. You can customize the tests based on your specific requirements.
Note: It is recommended to use a combination of unit tests and UI tests to ensure the quality and functionality of your app.
Also, it’s important to use testing tools and frameworks that are appropriate for the type of testing you want to perform. For example, use JUnit for unit testing and Espresso or UI Automator for UI testing.
Learn how to debug your app
To debug your Android app, you can use the following tools and techniques:
- Logcat: This is a logging tool in Android Studio that allows you to view the log messages generated by your app. You can use the
Log
class to write log messages in your code, and then view them in the Logcat window in Android Studio.
Example of using Logcat:
1Log.d("MyApp", "This is a debug message");
- Debugging with breakpoints: This is a technique for pausing the execution of your app and inspecting the state of the app at that point. You can set breakpoints in your code, and then run your app in debug mode to pause the execution at those breakpoints.
Example of using breakpoints:
- Set a breakpoint in your code by clicking on the left gutter next to the line of code where you want to pause the execution.
- Run your app in debug mode by clicking on the “Debug” button in Android Studio.
- When the execution reaches the breakpoint, the app will pause and you can inspect the state of the app, such as the values of variables and the call stack.
- Debugging with the Debugger: This is a tool in Android Studio that allows you to step through your code and inspect the state of the app at each step. You can use the Debugger to set breakpoints, step through the code, and inspect the state of the app.
Example of using the Debugger:
- Set a breakpoint in your code by clicking on the left gutter next to the line of code where you want to pause the execution.
- Run your app in debug mode by clicking on the “Debug” button in Android Studio.
- When the execution reaches the breakpoint, the app will pause and you can use the Debugger to step through the code, inspect the values of variables, and modify the values of variables.
- Debugging with the Android Profiler: This is a tool in Android Studio that allows you to monitor the performance of your app in real-time. You can use the Android Profiler to view the CPU, memory, network, and disk usage of your app.
Example of using the Android Profiler:
- Run your app in profiling mode by clicking on the “Profile” button in Android Studio.
- Use the Android Profiler to view the performance of your app, such as the CPU usage, memory usage, network usage, and disk usage.
These are just a few examples of how to debug your Android app. You can customize the debugging techniques based on your specific requirements.
Note: It is recommended to use a combination of logging, breakpoints, the Debugger, and the Android Profiler to ensure the performance and functionality of your app.
Also, it’s important to use the appropriate debugging tool for the type of debugging you want to perform. For example, use Logcat for logging, breakpoints and the Debugger for inspecting the state of the app, and the Android Profiler for monitoring the performance of the app
Publishing Your App
Learn about the different ways to publish your app
To publish your Android app, you can use the following ways:
- Google Play Store: This is the official app store for Android apps. You can publish your app on the Google Play Store by creating a Google Play Console account, preparing your app for release, and uploading your app to the Google Play Store.
Example of publishing your app on the Google Play Store:
- Create a Google Play Console account.
- Prepare your app for release by signing your app, creating a release build, and testing your app.
- Upload your app to the Google Play Store by creating a new release, uploading your app, and providing the necessary information, such as the app title, description, and price.
- Amazon Appstore: This is an app store for Android apps. You can publish your app on the Amazon Appstore by creating an Amazon Developer account, preparing your app for release, and uploading your app to the Amazon Appstore.
Example of publishing your app on the Amazon Appstore:
- Create an Amazon Developer account.
- Prepare your app for release by signing your app, creating a release build, and testing your app.
- Upload your app to the Amazon Appstore by creating a new app, uploading your app, and providing the necessary information, such as the app title, description, and price.
- Samsung Galaxy Store: This is an app store for Samsung devices. You can publish your app on the Samsung Galaxy Store by creating a Samsung Developer account, preparing your app for release, and uploading your app to the Samsung Galaxy Store.
Example of publishing your app on the Samsung Galaxy Store:
- Create a Samsung Developer account.
- Prepare your app for release by signing your app, creating a release build, and testing your app.
- Upload your app to the Samsung Galaxy Store by creating a new app, uploading your app, and providing the necessary information, such as the app title, description, and price.
- Third-party app stores: There are many third-party app stores that allow you to publish your Android app. You can publish your app on a third-party app store by creating an account, preparing your app for release, and uploading your app to the third-party app store.
Example of publishing your app on a third-party app store:
- Create an account on the third-party app store.
- Prepare your app for release by signing your app, creating a release build, and testing your app.
- Upload your app to the third-party app store by creating a new app, uploading your app, and providing the necessary information, such as the app title, description, and price.
These are just a few examples of how to publish your Android app. You can choose the appropriate app store based on your specific requirements.
Note: It is recommended to publish your app on multiple app stores to reach a wider audience. Also, it’s important to follow the guidelines and policies of each app store to ensure the successful publication of your app.
Prepare your app for release
1To prepare your Android app for release, you can follow these steps:
- Sign your app: This is the process of adding a digital signature to your app to identify the author of the app. You can sign your app using the Android Studio’s built-in signing tool or using the command line.
Example of signing your app using Android Studio:
- Open your app in Android Studio.
- Go to
Build > Generate Signed Bundle / APK
. - Follow the prompts to create a new key store or select an existing key store.
- Provide the necessary information, such as the key store password, key alias, and key password.
- Select the release build variant and generate the signed APK or AAB.
Example of signing your app using the command line:
- Open a terminal or command prompt.
- Navigate to the root directory of your app.
- Run the following command to generate a new key store:
1keytool -genkey -v -keystore my-release-key.jks -keyalg RSA -keysize 2048 -validity 10000 -alias my-alias
- Run the following command to sign your app:
1./gradlew assembleRelease
- Create a release build: This is the process of creating a build of your app that is optimized for release. You can create a release build using the Android Studio’s built-in build tools or using the command line.
Example of creating a release build using Android Studio:
- Open your app in Android Studio.
- Go to
Build > Generate Signed Bundle / APK
. - Follow the prompts to create a new key store or select an existing key store.
- Select the release build variant and generate the signed APK or AAB.
Example of creating a release build using the command line:
- Open a terminal or command prompt.
- Navigate to the root directory of your app.
- Run the following command to create a release build:
1./gradlew assembleRelease
- Test your app: This is the process of testing your app to ensure that it is working correctly and is free of bugs. You can test your app using the Android Emulator, a physical device, or a testing tool.
Example of testing your app using the Android Emulator:
- Open the Android Virtual Device Manager in Android Studio.
- Create a new virtual device or select an existing virtual device.
- Install your app on the virtual device and test it.
Example of testing your app using a physical device:
- Connect your physical device to your computer.
- Enable USB debugging on your physical device.
- Install your app on your physical device and test it.
Example of testing your app using a testing tool:
- Use a testing tool, such as Espresso or UI Automator, to write and run UI tests for your app.
- Optimize your app: This is the process of optimizing your app to improve its performance and reduce its size. You can optimize your app using the Android Profiler, the Android Profiler, or a performance optimization tool.
Example of optimizing your app using the Android Profiler:
- Open your app in Android Studio.
- Go to
View > Tool Windows > Android Profiler
. - Use the Android Profiler to monitor the performance of your app, such as the CPU usage, memory usage, network usage, and disk usage.
Example of optimizing your app using a performance optimization tool:
- Use a performance optimization tool, such as ProGuard or R8, to optimize the code and reduce the size of your app.
These are just a few examples of how to prepare your Android app for release. You can customize the preparation based on your specific requirements.
Note: It is recommended to test your app thoroughly and optimize it to ensure the best user experience. Also, it’s important to follow the guidelines and policies of the app store where you plan to publish your app
Publish your app on the Google Play Store
1To publish your Android app on the Google Play Store, you can follow these steps:
- Create a Google Play Console account: This is the process of creating a Google Play Console account to manage your app on the Google Play Store. You can create a Google Play Console account using a Google account.
Example of creating a Google Play Console account:
- Go to the Google Play Console website.
- Click on the “Create an account” button.
- Follow the prompts to create a new account or link an existing account.
- Provide the necessary information, such as the account type, contact information, and payment information.
- Prepare your app for release: This is the process of signing your app, creating a release build, and testing your app. You can prepare your app for release using the Android Studio’s built-in signing tool or using the command line.
Example of preparing your app for release using Android Studio:
- Open your app in Android Studio.
- Go to
Build > Generate Signed Bundle / APK
. - Follow the prompts to create a new key store or select an existing key store.
- Select the release build variant and generate the signed APK or AAB.
Example of preparing your app for release using the command line:
- Open a terminal or command prompt.
- Navigate to the root directory of your app.
- Run the following command to generate a new key store:
1keytool -genkey -v -keystore my-release-key.jks -keyalg RSA -keysize 2048 -validity 10000 -alias my-alias
- Run the following command to sign your app:
1./gradlew assembleRelease
- Create a new release: This is the process of creating a new release of your app on the Google Play Console. You can create a new release by providing the necessary information, such as the app title, description, and price.
Example of creating a new release on the Google Play Console:
- Go to the Google Play Console website.
- Select your app.
- Go to
Release management > App releases
. - Click on the “Create release” button.
- Follow the prompts to provide the necessary information, such as the app title, description, and price.
- Upload the signed APK or AAB.
- Publish your app: This is the process of making your app available to users on the Google Play Store. You can publish your app by clicking the “Review” button and then the “Rollout release” button on the Google Play Console.
Example of publishing your app on the Google Play Console:
- Go to the Google Play Console website.
- Select your app.
- Go to
Release management > App releases
. - Click on the “Review” button for the release you want to publish.
- Review the information and click the “Rollout release” button.
These are just a few examples of how to publish your Android app on the Google Play Store. You can customize the publication based on your specific requirements.
Note: It is recommended to test your app thoroughly and optimize it to ensure the best user experience. Also, it’s important to follow the guidelines and policies of the Google Play Store, such as the content guidelines and the app quality guidelines.
Here’s a code snippet for creating a WebView app:
MainActivity.kt
1class MainActivity : AppCompatActivity() {
2 override fun onCreate(savedInstanceState: Bundle?) {
3 super.onCreate(savedInstanceState)
4 setContentView(R.layout.activity_main)
5
6 val webView = findViewById<WebView>(R.id.webview)
7 webView.loadUrl("https://www.example.com")
8 webView.webViewClient = WebViewClient()
9 }
10}
activity_main.xml
1<?xml version="1.0" encoding="utf-8"?>
2<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
3 xmlns:tools="http://schemas.android.com/tools"
4 android:layout_width="match_parent"
5 android:layout_height="match_parent"
6 tools:context=".MainActivity">
7
8 <WebView
9 android:id="@+id/webview"
10 android:layout_width="match_parent"
11 android:layout_height="match_parent" />
12
13</RelativeLayout>
This is a basic example and can be expanded to include more features such as navigation, bookmarks, and offline support.