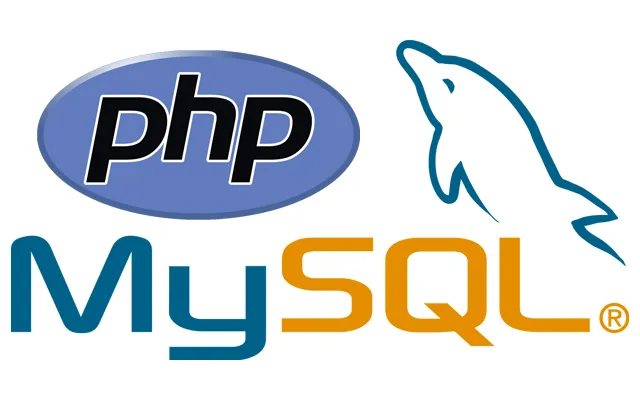
Building a Bioinformatics Database: MySQL and PHP Tutorial
January 5, 2024This comprehensive guide provides a step-by-step approach to building a bioinformatics database using MySQL and PHP, covering both the fundamental concepts and practical implementation aspects.
Table of Contents
Part 1: MySQL Basics for Bioinformatics Database
Introduction to MySQL:
Overview: MySQL is an open-source relational database management system (RDBMS) that plays a pivotal role in the world of database development. Developed by MySQL AB (now owned by Oracle Corporation), MySQL is known for its reliability, scalability, and ease of use. It is widely used by developers, businesses, and organizations to manage and organize their data.
Key Features:
- Relational Database: MySQL follows the relational model, organizing data into tables with rows and columns. It supports the SQL (Structured Query Language) for querying and managing the database.
- Open Source: MySQL is freely available under the GNU General Public License (GPL). Its open-source nature allows developers to access and modify the source code based on their requirements.
- Cross-Platform Compatibility: MySQL is designed to run on various platforms, including Windows, Linux, macOS, and more. This makes it a versatile choice for different types of applications.
- Scalability: MySQL can handle large amounts of data and is scalable for applications with growing data needs. It supports both vertical (adding more resources to a single server) and horizontal (adding more servers) scalability.
- Performance Optimization: MySQL is optimized for performance, offering various storage engines, indexing options, and caching mechanisms. This helps developers fine-tune the database for specific use cases.
- Data Security: MySQL provides robust security features, including user authentication, access control, and encryption. It ensures that only authorized users can access and manipulate the data.
- Community Support: With a large and active community of developers, MySQL benefits from ongoing improvements, updates, and a wealth of online resources, forums, and documentation.
Role in Database Development:
- Data Storage and Retrieval: MySQL serves as a reliable and efficient platform for storing and retrieving structured data. It allows developers to create, update, delete, and query data using SQL commands.
- Web Application Development: MySQL is commonly used as the backend database for web applications. It integrates seamlessly with programming languages like PHP, Python, Java, and others, providing a robust foundation for dynamic web content.
- Content Management Systems (CMS): Many popular CMS platforms, such as WordPress and Joomla, utilize MySQL as their database backend. It efficiently manages the content and user data for these systems.
- Business Applications: MySQL is widely employed in various business applications, including customer relationship management (CRM), enterprise resource planning (ERP), and other systems where structured data management is essential.
- Data Warehousing: MySQL can be used in data warehousing scenarios to store and manage large sets of data for analytical purposes. Its scalability and performance make it suitable for handling data-intensive tasks.
In conclusion, MySQL is a powerful and versatile relational database management system that plays a vital role in database development across a wide range of applications and industries. Its features, open-source nature, and active community support make it a popular choice for developers seeking a robust and scalable database solution.
Setting Up MySQL Environment:
1. Installation of MySQL Server:
On Windows:
- Download the MySQL Installer for Windows from the official MySQL website.
- Run the installer and follow the installation wizard.
- Choose the MySQL Server version you want to install.
- Configure MySQL Server by setting a root password and choosing the installation type (Development, Server Only, Full).
- Complete the installation process.
On Linux (Ubuntu as an example):
- Open a terminal.
- Update the package index:
sudo apt update
- Install the MySQL server package:
sudo apt install mysql-server
- During installation, you will be prompted to set a root password.
- Complete the installation process.
On macOS:
- You can use Homebrew to install MySQL on macOS.
- Open a terminal.
- Install Homebrew if you haven’t:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install MySQL:
brew install mysql
- Start the MySQL service:
brew services start mysql
- Set up the root password:
mysql_secure_installation
2. Configuration and Basic Security Measures:
Configuration:
- Locate the MySQL configuration file. On Linux, it is often found at
/etc/mysql/my.cnf
or/etc/my.cnf
. On Windows, it may be in the installation directory. - Edit the configuration file based on your requirements. Common configurations include adjusting buffer sizes, changing the default character set, etc.
- Restart the MySQL service after making changes.
Basic Security Measures:
a. Change the Root Password:
- Log in to MySQL:
mysql -u root -p
- Enter the current root password.
- Change the password:
ALTER USER 'root'@'localhost' IDENTIFIED BY 'new_password';
- Replace ‘new_password’ with your desired password.
b. Remove Anonymous Users:
- Run the following SQL command to remove anonymous users:
DROP USER ''@'localhost';
c. Remove Remote Root Logins:
- By default, MySQL allows root logins from any host. You may want to restrict this for security.
- Run the following SQL command to remove remote root logins:
DELETE FROM mysql.user WHERE User='root' AND Host NOT IN ('localhost', '127.0.0.1', '::1');
d. Remove the Test Database:
- MySQL comes with a test database that is not needed for production.
- Run the following SQL command to remove the test database:
DROP DATABASE IF EXISTS test;
e. Reload Privileges:
- After making changes to the user privileges, run:
FLUSH PRIVILEGES;
f. Enable the MySQL Firewall:
- MySQL 8.0 and later versions come with a built-in firewall.
- Run the following SQL command to enable the firewall:
ALTER USER 'root'@'localhost' REQUIRE SSL;
g. Regular Backups:
- Implement a regular backup strategy to prevent data loss. MySQL provides utilities like
mysqldump
for this purpose.
These steps provide a basic setup and security measures for your MySQL environment. Always refer to the official MySQL documentation for the version you are using for detailed instructions and security best practices.
Creating a Bioinformatics Database
Designing a database involves creating a schema that defines the structure and relationships between tables. For the “Disease Protein Database,” you can define tables to store information related to proteins, diseases, and external references. Here’s an example of a basic database schema using MySQL syntax:
-- Create Database
CREATE DATABASE IF NOT EXISTS DiseaseProteinDatabase;
USE DiseaseProteinDatabase;-- Table for Proteins
CREATE TABLE Proteins (
ProteinID INT AUTO_INCREMENT PRIMARY KEY,
ProteinName VARCHAR(255) NOT NULL,
ProteinLength INT,
ProteinFastaSequence TEXT,
PBDID VARCHAR(10), -- External link to Protein Data Bank
PubmedID VARCHAR(10) -- External link to PubMed
);
-- Table for Diseases
CREATE TABLE Diseases (
DiseaseID INT AUTO_INCREMENT PRIMARY KEY,
DiseaseName VARCHAR(255) NOT NULL
);
-- Table for Protein-Disease Relationships
CREATE TABLE ProteinDisease (
ProteinID INT,
DiseaseID INT,
PRIMARY KEY (ProteinID, DiseaseID),
FOREIGN KEY (ProteinID) REFERENCES Proteins(ProteinID),
FOREIGN KEY (DiseaseID) REFERENCES Diseases(DiseaseID)
);
Explanation:
- Proteins Table:
- Diseases Table:
DiseaseID
: Auto-incremented unique identifier for each disease.DiseaseName
: Name of the disease.
- ProteinDisease Table:
- Many-to-Many relationship table linking proteins to diseases.
ProteinID
: Foreign key referencingProteins
table.DiseaseID
: Foreign key referencingDiseases
table.- This table allows the association of multiple proteins with multiple diseases.
Note:
- Adjust the data types and lengths based on your specific requirements.
- Ensure to create indexes and foreign key constraints to maintain data integrity.
- This is a simplified example; in a real-world scenario, you might need additional tables and fields depending on your specific use case.
This database schema provides a foundation for storing information about proteins, diseases, and their relationships in the context of a disease protein database. Adjustments can be made based on your specific data and application requirements.
Basic MySQL Queries
Below are basic MySQL queries for inserting data into the database, retrieving data using SELECT statements, and updating/deleting records based on the previously defined “Disease Protein Database” schema:
1. Inserting Data:
-- Insert data into Proteins table
INSERT INTO Proteins (ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID)
VALUES
('Protein1', 300, 'ATGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGC', 'PBD001', 'PMID001'),
('Protein2', 450, 'ATGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGC', 'PBD002', 'PMID002'),
('Protein3', 600, 'ATGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGCTAGC', 'PBD003', 'PMID003');-- Insert data into Diseases table
INSERT INTO Diseases (DiseaseName)
VALUES
('DiseaseA'),
('DiseaseB'),
('DiseaseC');
-- Insert data into ProteinDisease table (associating proteins with diseases)
INSERT INTO ProteinDisease (ProteinID, DiseaseID)
VALUES
(1, 1), -- Protein1 associated with DiseaseA
(2, 2), -- Protein2 associated with DiseaseB
(3, 3); -- Protein3 associated with DiseaseC
2. Retrieving Data (SELECT Statements):
-- Retrieve all proteins
SELECT * FROM Proteins;-- Retrieve all diseases
SELECT * FROM Diseases;
-- Retrieve proteins associated with a specific disease (e.g., DiseaseA)
SELECT Proteins.*
FROM Proteins
JOIN ProteinDisease ON Proteins.ProteinID = ProteinDisease.ProteinID
JOIN Diseases ON ProteinDisease.DiseaseID = Diseases.DiseaseID
WHERE Diseases.DiseaseName = 'DiseaseA';
3. Updating Records:
-- Update the ProteinName for Protein1
UPDATE Proteins
SET ProteinName = 'UpdatedProtein1'
WHERE ProteinID = 1;
4. Deleting Records:
-- Delete Protein2 from Proteins table
DELETE FROM Proteins
WHERE ProteinID = 2;-- Delete the association of Protein3 with DiseaseC from ProteinDisease table
DELETE FROM ProteinDisease
WHERE ProteinID = 3 AND DiseaseID = 3;
Remember to adjust the data and conditions based on your specific use case. These queries provide a basic understanding of inserting, retrieving, updating, and deleting data in a MySQL database. Always ensure to handle data modifications with caution, especially when dealing with production databases.
To insert data into MySQL from a text file, you can use the LOAD DATA INFILE
statement. This statement allows you to load data from a text file into a MySQL table. Here’s a basic example:
Assuming you have a text file named data.txt
with the following content:
ProteinName,ProteinLength,ProteinFastaSequence,PBDID,PubmedID
Protein1,300,ATGCTAGCTAGCTAGCTAGCTAGC,PBD001,PMID001
Protein2,450,ATGCTAGCTAGCTAGCTAGCTAGC,PBD002,PMID002
Protein3,600,ATGCTAGCTAGCTAGCTAGCTAGC,PBD003,PMID003
And you want to insert this data into the Proteins
table, you can use the following MySQL query:
-- Use the appropriate file path based on your system
LOAD DATA INFILE '/path/to/data.txt'
INTO TABLE Proteins
FIELDS TERMINATED BY ','
LINES TERMINATED BY '\n'
IGNORE 1 LINES; -- Skip the header row
Explanation:
LOAD DATA INFILE '/path/to/data.txt'
: Specifies the path to your text file.INTO TABLE Proteins
: Specifies the target table where data should be loaded.FIELDS TERMINATED BY ','
: Specifies that fields in the text file are separated by commas.LINES TERMINATED BY '\n'
: Specifies that each line in the text file is terminated by a newline character.IGNORE 1 LINES
: Skips the first line of the file (assumed to be headers).
Make sure to replace /path/to/data.txt
with the actual path to your text file, and adjust the table and file format settings based on your specific use case.
Note: The user executing the LOAD DATA INFILE
statement needs the FILE
privilege. If you encounter issues related to file permissions, you may need to adjust MySQL’s security settings or use the --local-infile=1
option when starting the MySQL client.
1. Security Considerations:
- Be cautious when using
LOAD DATA INFILE
as it poses security risks. Ensure that only trusted users have theFILE
privilege. - Avoid using this method with untrusted input or files from unauthenticated sources.
2. File Path Handling:
- Use the correct file path based on your operating system. Paths might be different on Windows, Linux, or macOS.
- Make sure MySQL has the necessary file permissions to read from the specified file path.
3. Field and Line Terminators:
- Adjust the
FIELDS TERMINATED BY
andLINES TERMINATED BY
clauses based on your text file’s format. For example, if fields are separated by tabs, useFIELDS TERMINATED BY '\t'
.
4. Column Mapping:
- Ensure that the order of columns in your text file matches the order of columns in the MySQL table. MySQL will map fields in the order they appear in the
LOAD DATA INFILE
statement.
5. Character Encoding:
- If your text file contains special characters, specify the character set using the
CHARACTER SET
clause. For example, addCHARACTER SET utf8
if your file uses UTF-8 encoding.
Here’s an extended example with additional considerations:
-- Use the appropriate file path based on your system
LOAD DATA INFILE '/path/to/data.txt'
INTO TABLE Proteins
FIELDS TERMINATED BY ','
LINES TERMINATED BY '\n'
IGNORE 1 LINES -- Skip the header row
(PROTEINNAME, PROTEINLENGTH, PROTEINFASTASEQUENCE, PBDID, PUBMEDID) -- Specify column names
SET CREATED_AT = NOW(); -- Set a default value for the CREATED_AT column
In this example, we added a column mapping using the (column1, column2, ...)
syntax to explicitly specify which columns in the text file correspond to which columns in the MySQL table. Also, we used the SET
clause to set a default value for the CREATED_AT
column.
Remember to adjust the query based on the specifics of your text file and MySQL table schema.
Advanced MySQL Concepts
1. Using JOIN to Fetch Data from Multiple Tables:
The JOIN
operation is used to combine rows from two or more tables based on a related column between them. Here’s an example using the “Disease Protein Database” schema:
-- Fetch proteins along with associated diseases
SELECT Proteins.ProteinID, ProteinName, ProteinLength, DiseaseName
FROM Proteins
JOIN ProteinDisease ON Proteins.ProteinID = ProteinDisease.ProteinID
JOIN Diseases ON ProteinDisease.DiseaseID = Diseases.DiseaseID;
In this example, the JOIN
clause is used to combine data from the Proteins
, ProteinDisease
, and Diseases
tables based on their relationships.
2. Indexing for Performance Optimization:
Indexes enhance the speed of data retrieval operations on a database table. Common types of indexes include PRIMARY KEY
, UNIQUE
, and INDEX
. Here’s how you might create an index:
-- Create an index on the ProteinName column for faster retrieval
CREATE INDEX idx_ProteinName ON Proteins (ProteinName);-- Create a composite index on multiple columns for a specific query
CREATE INDEX idx_ProteinDisease ON ProteinDisease (ProteinID, DiseaseID);
Indexes can significantly improve query performance, but be cautious not to over-index, as it may impact write performance.
3. Implementing Constraints (e.g., Foreign Keys):
Constraints ensure the integrity and consistency of data in the database. Foreign keys are commonly used to establish relationships between tables. Example:
-- Adding a foreign key constraint to the ProteinDisease table
ALTER TABLE ProteinDisease
ADD CONSTRAINT fk_ProteinID
FOREIGN KEY (ProteinID)
REFERENCES Proteins (ProteinID)
ON DELETE CASCADE; -- Cascade delete when a referenced protein is deleted
In this example, a foreign key constraint is added to the ProteinDisease
table, linking the ProteinID
column to the Proteins
table’s ProteinID
column. The ON DELETE CASCADE
option ensures that if a protein is deleted, corresponding entries in ProteinDisease
will be deleted as well.
These advanced MySQL concepts—JOIN
for data retrieval from multiple tables, indexing for performance optimization, and constraints like foreign keys—provide powerful tools for designing efficient and consistent database structures. When using these concepts, it’s important to understand the specific needs of your application and database design.
4. Transactions:
Transactions ensure the atomicity, consistency, isolation, and durability (ACID properties) of database operations. Use the BEGIN
, COMMIT
, and ROLLBACK
statements to manage transactions:
-- Begin a transaction
BEGIN;-- SQL statements for the transaction
-- Commit the transaction
COMMIT;
-- Rollback the transaction in case of an error
ROLLBACK;
Wrap multiple SQL statements within a transaction to ensure that either all changes are applied (COMMIT
) or none are applied (ROLLBACK
).
5. Stored Procedures:
Stored procedures are precompiled SQL statements that can be executed with a single call. They enhance code reusability and encapsulate complex logic. Here’s a basic example:
-- Create a stored procedure
DELIMITER //CREATE PROCEDURE GetProteinsByDisease(IN diseaseName VARCHAR(255))
BEGIN
SELECT Proteins.ProteinID, ProteinName, ProteinLength
FROM Proteins
JOIN ProteinDisease ON Proteins.ProteinID = ProteinDisease.ProteinID
JOIN Diseases ON ProteinDisease.DiseaseID = Diseases.DiseaseID
WHERE Diseases.DiseaseName = diseaseName;
END //
DELIMITER ;
Call the stored procedure:
-- Call the stored procedure
CALL GetProteinsByDisease('DiseaseA');
6. Views:
Views are virtual tables generated from the result of a SELECT query. They simplify complex queries and abstract the underlying structure. Here’s an example:
-- Create a view to get proteins associated with diseases
CREATE VIEW ProteinDiseaseView AS
SELECT Proteins.ProteinID, ProteinName, ProteinLength, DiseaseName
FROM Proteins
JOIN ProteinDisease ON Proteins.ProteinID = ProteinDisease.ProteinID
JOIN Diseases ON ProteinDisease.DiseaseID = Diseases.DiseaseID;
Query the view:
-- Query the view
SELECT * FROM ProteinDiseaseView;
These advanced MySQL concepts contribute to efficient database management and application development. Properly implementing transactions, stored procedures, and views can lead to better performance, maintainability, and security in your MySQL database. Always consider the specific needs and requirements of your application when applying these concepts.
Part 2: PHP Basics for Bioinformatics Database Interaction
Introduction to PHP for Database Connectivity:
1. Server-Side Scripting Language:
PHP (Hypertext Preprocessor) is a widely used server-side scripting language designed for web development. It is embedded in HTML code and executed on the server, producing dynamic web pages. PHP is particularly well-suited for database connectivity, making it a key technology for building interactive and data-driven web applications.
2. Basic Syntax and Concepts:
- Variables:php
$variable_name = value;
- Data Types:
- PHP supports various data types, including integers, floats, strings, booleans, arrays, and objects.
- Conditional Statements:php
if (condition) {
// code to be executed if the condition is true
} elseif (another_condition) {
// code to be executed if the first condition is false and the second is true
} else {
// code to be executed if both conditions are false
}
- Loops:
- For Loop:php
for ($i = 0; $i < 5; $i++) {
// code to be executed
}
- While Loop:php
while (condition) {
// code to be executed
}
- For Loop:
- Functions:php
function functionName($parameter1, $parameter2) {
// code to be executed
}
- Arrays:php
$colors = array("Red", "Green", "Blue");
- Associative Arrays:php
$person = array("name" => "John", "age" => 30, "city" => "New York");
- Super Global Variables:
- PHP provides several super global variables, including
$_GET
,$_POST
,$_SESSION
, and$_COOKIE
, which are used to collect form data, store user session information, and handle cookies.
- PHP provides several super global variables, including
Example: Connecting to MySQL Database:
$servername = "localhost";
$username = "username";
$password = "password";
$database = "database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
// Close connection
$conn->close();
In this example, replace “localhost,” “username,” “password,” and “database” with your MySQL server details. The script connects to a MySQL database using the mysqli
extension, checks the connection, and outputs a success message if the connection is established.
Understanding PHP’s basic syntax and concepts is crucial for effective web development, especially when working with databases. This knowledge forms the foundation for building dynamic and interactive web applications.
3. Database Connectivity in PHP:
PHP provides several extensions to connect to different database management systems. The mysqli
extension is commonly used for MySQL databases. Here’s a simple example of querying a MySQL database using PHP:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SQL query
$sql = "SELECT column1, column2 FROM your_table";
$result = $conn->query($sql);
// Check if the query was successful
if ($result) {
// Fetch data
while ($row = $result->fetch_assoc()) {
echo "Column1: " . $row["column1"] . " - Column2: " . $row["column2"] . "<br>";
}
} else {
echo "Error: " . $conn->error;
}
// Close connection
$conn->close();
In this example, replace “localhost,” “your_username,” “your_password,” “your_database,” “your_table,” “column1,” and “column2” with your specific database details. The script connects to the MySQL database, executes a SELECT query, and outputs the results.
4. Error Handling in PHP:
PHP provides mechanisms for error handling to ensure that potential issues are addressed gracefully. The die()
function is often used to display an error message and terminate the script execution:
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
Additionally, you can use try
, catch
, and finally
blocks for more advanced error handling using exceptions:
try {
// code that may throw an exception
} catch (Exception $e) {
echo "Caught exception: " . $e->getMessage();
} finally {
// code that will always run, regardless of an exception
}
5. Security Considerations:
When working with databases, it’s crucial to handle user input securely to prevent SQL injection attacks. Always use prepared statements and parameterized queries to sanitize and validate input data.
Example of a prepared statement in PHP:
$stmt = $conn->prepare("SELECT column1, column2 FROM your_table WHERE id = ?");
$stmt->bind_param("i", $id); // "i" represents integer type
$id = 1;
$stmt->execute();
$result = $stmt->get_result();
These are foundational concepts for working with PHP and databases. As you delve deeper into PHP development, you’ll encounter additional features, frameworks, and best practices for building robust and secure web applications.
6. Sessions and Cookies:
PHP provides built-in support for managing sessions and cookies, allowing you to persist user data between requests.
- Sessions:
- To start a session, use
session_start()
. You can then store and retrieve session variables:php// Start a session
session_start();// Store data in session
$_SESSION['user_id'] = 123;// Retrieve data from session
$userId = $_SESSION['user_id'];
- To start a session, use
- Cookies:
- Set cookies using
setcookie()
:php// Set a cookie
setcookie("user_name", "John Doe", time() + 3600, "/");
The above example sets a cookie named “user_name” with the value “John Doe” that expires in one hour.
- Set cookies using
7. Sending Email:
PHP allows you to send emails easily using the mail()
function. Here’s a basic example:
$to = "[email protected]";
$subject = "Hello";
$message = "This is a test email.";
$headers = "From: [email protected]";mail($to, $subject, $message, $headers);
Ensure that your server is configured to allow sending emails, and be cautious to prevent email header injections.
8. File Handling:
PHP provides functions for reading from and writing to files. Here’s a simple example of reading a file:
$filename = "example.txt";
$fileContent = file_get_contents($filename);
echo $fileContent;
For writing to a file:
$filename = "example.txt";
$data = "Hello, this is some data.";
file_put_contents($filename, $data);
9. Object-Oriented Programming (OOP):
PHP supports object-oriented programming, allowing you to organize code into classes and objects. Here’s a basic example:
class Car {
public $color;
public $model; public function __construct($color, $model) {
$this->color = $color;
$this->model = $model;
}
public function displayInfo() {
echo "Color: " . $this->color . ", Model: " . $this->model;
}
}
$myCar = new Car("Blue", "Sedan");
$myCar->displayInfo();
10. Frameworks and Libraries:
PHP has several popular frameworks and libraries that simplify and accelerate web development. Some notable examples include Laravel, Symfony, CodeIgniter, and Composer (for dependency management).
These advanced features and concepts provide a solid foundation for developing complex and feature-rich web applications with PHP. As you progress, explore PHP frameworks and libraries to streamline your development process and enhance the security and maintainability of your applications.
Connecting PHP to MySQL
To connect PHP to MySQL, you can use the mysqli
extension, which provides an interface to communicate with MySQL databases. Here’s a step-by-step guide on how to establish a connection and handle connection errors:
1. Establishing a Connection:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
// Perform database operations here
// Close connection
$conn->close();
- Replace “localhost,” “your_username,” “your_password,” and “your_database” with your MySQL server details.
- The
mysqli
constructor is used to create a new connection object ($conn
), andconnect_error
is checked to verify if the connection was successful. - If the connection fails, the script terminates and displays an error message using
die()
.
2. Handling Connection Errors:
When handling connection errors, it’s crucial to provide meaningful error messages during development and log errors for debugging in a production environment. Here’s an example of how to log connection errors:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
// Log the error to a file or a log database table
error_log("Connection failed: " . $conn->connect_error, 3, "error.log");
// Display a generic error message to the user
die("Oops! Something went wrong. Please try again later.");
}
echo "Connected successfully";
// Perform database operations here
// Close connection
$conn->close();
In this example:
- The
error_log()
function is used to log the error message to a file named “error.log.” - The third parameter (3) in
error_log()
specifies that the error message should be appended to the file. You may adjust this parameter based on your logging requirements. - A generic error message is displayed to the user, providing a friendly message while the detailed error is logged for developers.
By handling connection errors in a thoughtful way, you can ensure that issues are identified and addressed promptly during development and maintain a user-friendly experience in a production environment.
Executing Basic PHP-MySQL Queries
1. SELECT Queries:
Executing a SELECT query in PHP involves preparing the query, executing it, and handling the results. Here’s a basic example:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SELECT query
$sql = "SELECT column1, column2 FROM your_table";
$result = $conn->query($sql);
// Check if the query was successful
if ($result) {
// Fetch data
while ($row = $result->fetch_assoc()) {
echo "Column1: " . $row["column1"] . " - Column2: " . $row["column2"] . "<br>";
}
} else {
echo "Error: " . $conn->error;
}
// Close connection
$conn->close();
In this example:
- Replace “localhost,” “your_username,” “your_password,” “your_database,” and “your_table” with your specific database details.
- The SELECT query retrieves data from the specified table.
- The
query()
method executes the query, and the result is stored in the$result
variable. - The
fetch_assoc()
method fetches each row as an associative array, allowing you to access columns by their names.
2. INSERT Queries:
Executing an INSERT query in PHP involves preparing the query, executing it, and checking if the insertion was successful. Here’s an example:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// INSERT query
$sql = "INSERT INTO your_table (column1, column2) VALUES ('value1', 'value2')";
// Execute the query
if ($conn->query($sql) === TRUE) {
echo "Record inserted successfully";
} else {
echo "Error: " . $conn->error;
}
// Close connection
$conn->close();
In this example:
- The INSERT query inserts a new record into the specified table.
- The
query()
method is used to execute the query, and success is checked using=== TRUE
.
3. UPDATE Queries:
Executing an UPDATE query in PHP involves preparing the query, executing it, and checking if the update was successful. Here’s an example:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// UPDATE query
$sql = "UPDATE your_table SET column1 = 'new_value' WHERE condition";
// Execute the query
if ($conn->query($sql) === TRUE) {
echo "Record updated successfully";
} else {
echo "Error: " . $conn->error;
}
// Close connection
$conn->close();
In this example:
- The UPDATE query modifies existing records in the specified table based on a condition.
- The
query()
method is used to execute the query.
4. DELETE Queries:
Executing a DELETE query in PHP involves preparing the query, executing it, and checking if the deletion was successful. Here’s an example:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// DELETE query
$sql = "DELETE FROM your_table WHERE condition";
// Execute the query
if ($conn->query($sql) === TRUE) {
echo "Record deleted successfully";
} else {
echo "Error: " . $conn->error;
}
// Close connection
$conn->close();
In this example:
- The DELETE query removes records from the specified table based on a condition.
- The
query()
method is used to execute the query.
Handling Query Results:
- The
query()
method returns amysqli_result
object for SELECT queries. You can fetch results using methods likefetch_assoc()
orfetch_row()
. - For non-SELECT queries (INSERT, UPDATE, DELETE),
query()
returnsTRUE
on success andFALSE
on failure. - Use the
$conn->error
property to get detailed error information if a query fails.
These examples cover basic PHP-MySQL operations, and you can build on them as you explore more complex database interactions.
Building a Simple Interface with PHP
o build a simple interface with PHP for interacting with a bioinformatics database, you can create HTML forms to collect user input and integrate PHP scripts to handle form submissions. Here’s an example:
1. HTML Form (index.html):
Create an HTML form with input fields to collect information. Save this as index.html
:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bioinformatics Database Interface</title>
</head>
<body>
<h1>Bioinformatics Database Interface</h1> <form action="process.php" method="post">
<label for="proteinName">Protein Name:</label>
<input type="text" id="proteinName" name="proteinName" required>
<label for="proteinLength">Protein Length:</label>
<input type="number" id="proteinLength" name="proteinLength" required>
<label for="fastaSequence">Fasta Sequence:</label>
<textarea id="fastaSequence" name="fastaSequence" rows="4" required></textarea>
<label for="pbdID">PBD ID:</label>
<input type="text" id="pbdID" name="pbdID" required>
<label for="pubmedID">Pubmed ID:</label>
<input type="text" id="pubmedID" name="pubmedID" required>
<button type="submit">Submit</button>
</form>
</body>
</html>
This form includes input fields for Protein Name, Protein Length, Fasta Sequence, PBD ID, and Pubmed ID. The form’s action
attribute is set to “process.php,” indicating that form submissions will be handled by the PHP script.
2. PHP Script (process.php):
Create a PHP script to handle form submissions. Save this as process.php
:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Process form submission
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$proteinName = $_POST["proteinName"];
$proteinLength = $_POST["proteinLength"];
$fastaSequence = $_POST["fastaSequence"];
$pbdID = $_POST["pbdID"];
$pubmedID = $_POST["pubmedID"];
// Perform INSERT query
$sql = "INSERT INTO Proteins (ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID)
VALUES ('$proteinName', $proteinLength, '$fastaSequence', '$pbdID', '$pubmedID')";
if ($conn->query($sql) === TRUE) {
echo "Record inserted successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
// Close connection
$conn->close();
This PHP script handles the form submission by extracting data from $_POST
, preparing an INSERT query, and executing it. The script then provides feedback to the user.
Note:
- Always sanitize and validate user input to prevent SQL injection.
- Ensure proper error handling and validation for real-world applications.
Remember to replace “localhost,” “your_username,” “your_password,” and “your_database” with your specific database details.
Displaying Bioinformatics Data with PHP
To retrieve and display bioinformatics data from a MySQL database using PHP, you can build on the previous example. Here’s an extended version that fetches data from the database and displays it dynamically:
1. HTML Interface (index.html):
Assume you have a page to display bioinformatics data. Update your index.html
to include a section for displaying data:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bioinformatics Database Interface</title>
</head>
<body>
<h1>Bioinformatics Database Interface</h1> <!-- Bioinformatics Data Form -->
<form action="process.php" method="post">
<!-- Form fields as before... -->
<!-- ... -->
<button type="submit">Submit</button>
</form>
<hr>
<!-- Display Bioinformatics Data -->
<h2>Bioinformatics Data</h2>
<div id="bioinformaticsData">
</div>
</body>
</html>
This form includes an additional section to display bioinformatics data. It includes a placeholder div
with the id “bioinformaticsData” where data will be dynamically loaded.
2. PHP Script for Displaying Data (display_data.php):
Create a separate PHP script to handle the display of bioinformatics data. Save this as display_data.php
:
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SELECT query to retrieve bioinformatics data
$sql = "SELECT ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID FROM Proteins";
$result = $conn->query($sql);
// Check if the query was successful
if ($result->num_rows > 0) {
// Output data in a dynamic table
echo "<table border='1'>
<tr>
<th>Protein Name</th>
<th>Protein Length</th>
<th>Protein Fasta Sequence</th>
<th>PBD ID</th>
<th>Pubmed ID</th>
</tr>";
while ($row = $result->fetch_assoc()) {
echo "<tr>
<td>{$row['ProteinName']}</td>
<td>{$row['ProteinLength']}</td>
<td>{$row['ProteinFastaSequence']}</td>
<td>{$row['PBDID']}</td>
<td>{$row['PubmedID']}</td>
</tr>";
}
echo "</table>";
} else {
echo "No bioinformatics data found.";
}
// Close connection
$conn->close();
In this script:
- A SELECT query retrieves bioinformatics data from the “Proteins” table.
- If the query is successful, the data is displayed in an HTML table.
- If no data is found, a message is displayed.
Note:
- Always validate and sanitize user input to prevent SQL injection.
- Consider implementing pagination or other techniques for handling large datasets.
Remember to replace “localhost,” “your_username,” “your_password,” and “your_database” with your specific database details.
Part 3: Integrating MySQL and PHP for the Bioinformatics Database
Building a Complete Bioinformatics Web Application
Building a complete bioinformatics web application involves combining MySQL database functionality with a PHP interface to design an interactive web interface. Below is an example that includes various features like data display, form submissions, and interactivity.
1. HTML Interface (index.html):
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Disease Protein Database</title>
<style>
table {
border-collapse: collapse;
width: 100%;
} table, th, td {
border: 1px solid black;
}
th, td {
padding: 8px;
text-align: left;
}
</style>
</head>
<body>
<h1>Disease Protein Database</h1> <!-- Disease Protein Data Form -->
<form action="process.php" method="post">
<label for="proteinName">Protein Name:</label>
<input type="text" id="proteinName" name="proteinName" required>
<label for="proteinLength">Protein Length:</label>
<input type="number" id="proteinLength" name="proteinLength" required>
<label for="fastaSequence">Fasta Sequence:</label>
<textarea id="fastaSequence" name="fastaSequence" rows="4" required></textarea>
<label for="pbdID">PBD ID:</label>
<input type="text" id="pbdID" name="pbdID" required>
<label for="pubmedID">Pubmed ID:</label>
<input type="text" id="pubmedID" name="pubmedID" required>
<button type="submit">Submit</button>
</form>
<hr>
<!-- Display Disease Protein Data -->
<h2>Disease Protein Data</h2>
<div id="bioinformaticsData">
</div>
</body>
</html>
2. PHP Script for Displaying Data (display_data.php):
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SELECT query to retrieve disease protein data
$sql = "SELECT ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID FROM Proteins";
$result = $conn->query($sql);
// Check if the query was successful
if ($result->num_rows > 0) {
// Output data in a dynamic table
echo "<table>
<tr>
<th>Protein Name</th>
<th>Protein Length</th>
<th>Protein Fasta Sequence</th>
<th>PBD ID</th>
<th>Pubmed ID</th>
</tr>";
while ($row = $result->fetch_assoc()) {
echo "<tr>
<td>{$row['ProteinName']}</td>
<td>{$row['ProteinLength']}</td>
<td>{$row['ProteinFastaSequence']}</td>
<td>{$row['PBDID']}</td>
<td>{$row['PubmedID']}</td>
</tr>";
}
echo "</table>";
} else {
echo "No disease protein data found.";
}
// Close connection
$conn->close();
3. PHP Script for Processing Form (process.php):
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Process form submission
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$proteinName = $_POST["proteinName"];
$proteinLength = $_POST["proteinLength"];
$fastaSequence = $_POST["fastaSequence"];
$pbdID = $_POST["pbdID"];
$pubmedID = $_POST["pubmedID"];
// Perform INSERT query
$sql = "INSERT INTO Proteins (ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID)
VALUES ('$proteinName', $proteinLength, '$fastaSequence', '$pbdID', '$pubmedID')";
if ($conn->query($sql) === TRUE) {
echo "Record inserted successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
}
// Close connection
$conn->close();
Note:
- Always validate and sanitize user input to prevent SQL injection.
- The above examples assume a simplified structure; real-world applications would require more robust validation, error handling, and security measures.
Implementing External Links
To implement clickable external links for the PBD ID and Pubmed ID in your bioinformatics web application, you can modify the PHP script for displaying data (display_data.php
). Here’s an updated version:
Updated PHP Script for Displaying Data (display_data.php):
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// SELECT query to retrieve disease protein data
$sql = "SELECT ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID FROM Proteins";
$result = $conn->query($sql);
// Check if the query was successful
if ($result->num_rows > 0) {
// Output data in a dynamic table
echo "<table>
<tr>
<th>Protein Name</th>
<th>Protein Length</th>
<th>Protein Fasta Sequence</th>
<th>PBD ID</th>
<th>Pubmed ID</th>
</tr>";
while ($row = $result->fetch_assoc()) {
echo "<tr>
<td>{$row['ProteinName']}</td>
<td>{$row['ProteinLength']}</td>
<td>{$row['ProteinFastaSequence']}</td>
<td><a href='https://www.rcsb.org/structure/{$row['PBDID']}' target='_blank'>{$row['PBDID']}</a></td>
<td><a href='https://pubmed.ncbi.nlm.nih.gov/{$row['PubmedID']}' target='_blank'>{$row['PubmedID']}</a></td>
</tr>";
}
echo "</table>";
} else {
echo "No disease protein data found.";
}
// Close connection
$conn->close();
In this updated version:
- The PBD ID and Pubmed ID columns now contain hyperlinks (
<a>
tags) with thehref
attribute pointing to external URLs. - The
target='_blank'
attribute is added to the<a>
tags to open the links in a new browser tab or window, enhancing the user experience. - Clicking on the PBD ID will open the corresponding entry on the RCSB PDB website, and clicking on the Pubmed ID will open the respective entry on the PubMed website.
Make sure to replace “localhost,” “your_username,” “your_password,” and “your_database” with your specific database details.
Security Measures
Implementing secure practices is crucial to protect your bioinformatics web application from vulnerabilities. Here are some measures to prevent SQL injection and considerations for user authentication and authorization:
1. Preventing SQL Injection:
To prevent SQL injection, always use parameterized queries or prepared statements. Modify your PHP scripts accordingly:
Updated PHP Script for Processing Form (process.php):
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$database = "your_database";// Create connection
$conn = new mysqli($servername, $username, $password, $database);
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Process form submission
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$proteinName = mysqli_real_escape_string($conn, $_POST["proteinName"]);
$proteinLength = mysqli_real_escape_string($conn, $_POST["proteinLength"]);
$fastaSequence = mysqli_real_escape_string($conn, $_POST["fastaSequence"]);
$pbdID = mysqli_real_escape_string($conn, $_POST["pbdID"]);
$pubmedID = mysqli_real_escape_string($conn, $_POST["pubmedID"]);
// Perform parameterized INSERT query
$sql = $conn->prepare("INSERT INTO Proteins (ProteinName, ProteinLength, ProteinFastaSequence, PBDID, PubmedID)
VALUES (?, ?, ?, ?, ?)");
$sql->bind_param("sisss", $proteinName, $proteinLength, $fastaSequence, $pbdID, $pubmedID);
if ($sql->execute()) {
echo "Record inserted successfully";
} else {
echo "Error: " . $sql->error;
}
$sql->close();
}
// Close connection
$conn->close();
In this updated script:
mysqli_real_escape_string()
is used to escape special characters in user input, preventing SQL injection.- The
prepare()
method is used to create a prepared statement with placeholders, andbind_param()
binds variables to the placeholders, ensuring secure parameterized queries.
2. User Authentication and Authorization Considerations:
- User Authentication:
- Implement a secure user authentication system to ensure that only authorized users can access the application.
- Use secure hashing algorithms (e.g., bcrypt) to store user passwords.
- Store user credentials securely and avoid storing plaintext passwords.
- User Authorization:
- Define roles and permissions for different user types (e.g., admin, regular user).
- Restrict access to certain functionalities based on user roles.
- Validate user permissions before allowing actions such as data modification or deletion.
3. Additional Security Measures:
- Input Validation:
- Implement client-side and server-side input validation to ensure that users enter data in the expected format.
- HTTPS:
- Use HTTPS to encrypt data transmitted between the client and server, preventing man-in-the-middle attacks.
- Error Handling:
- Implement proper error handling to avoid exposing sensitive information to users.
- Session Security:
- Secure session management to prevent session hijacking or fixation.
- Regular Security Audits:
- Regularly audit your application for security vulnerabilities.
Always stay informed about the latest security best practices and keep your software and libraries up to date to address any security patches or updates. Consider consulting security experts for a thorough security assessment of your bioinformatics web application.
Optimizing Database Performance
Optimizing database performance is essential for ensuring the responsiveness and efficiency of your bioinformatics web application. Here are strategies for optimizing database queries and PHP scripts, as well as using caching and indexing to improve speed:
1. Optimize Database Queries:
- Use Indexing:
- Identify columns frequently used in WHERE clauses or JOIN conditions and create indexes on those columns.
- Be cautious with over-indexing, as it can lead to increased storage requirements and slower write operations.
- *Avoid SELECT :
- Only retrieve the columns that are necessary for your application instead of using SELECT *.
- This reduces the amount of data transferred between the database and the application, improving performance.
- Use LIMIT and OFFSET:
- When retrieving large result sets, use LIMIT to restrict the number of rows returned.
- Use OFFSET for paginated results to efficiently navigate through large datasets.
- Optimize JOIN Operations:
- Optimize JOIN operations by selecting only the necessary columns.
- Use INNER JOIN instead of OUTER JOIN if possible, as INNER JOIN is generally faster.
- Batch Processing:
- Use batch processing for bulk inserts, updates, or deletes instead of individual queries.
- Wrap multiple operations in a transaction to ensure atomicity and consistency.
2. Optimize PHP Scripts:
- Minimize Database Queries:
- Reduce the number of database queries by fetching only the necessary data.
- Cache frequently accessed or static data to avoid unnecessary queries.
- Use Prepared Statements:
- Use prepared statements and parameterized queries to prevent SQL injection.
- Prepared statements can be cached by the database, improving performance for repeated queries.
- Avoid Resource-Intensive Operations:
- Minimize resource-intensive operations in PHP, such as large file processing or complex computations.
- Offload resource-heavy tasks to background processes or dedicated services.
3. Implement Caching:
- Query Result Caching:
- Cache frequently executed database queries or query results to reduce the load on the database.
- Consider using a caching mechanism like Redis or Memcached for storing key-value pairs.
- Page Caching:
- Cache entire pages or components of pages to serve static content for a predefined duration.
- Tools like Varnish or caching modules in web servers can be employed for page caching.
4. Use Indexing:
- Database Indexing:
- Implement proper indexing on columns used in WHERE clauses, JOIN conditions, and ORDER BY clauses.
- Regularly review and optimize indexes based on query patterns and usage.
- Full-Text Indexing:
- If your application involves searching text fields, consider using full-text indexing for better search performance.
- Full-text indexing is available in many relational databases.
5. Leverage Connection Pooling:
- Use connection pooling to reduce the overhead of opening and closing database connections.
- Connection pooling maintains a pool of database connections that can be reused, improving performance and scalability.
6. Monitor and Analyze Performance:
- Regularly monitor and analyze database and application performance using profiling tools.
- Identify and address bottlenecks, slow queries, or inefficient code.
7. Optimize Web Server Configuration:
- Tune your web server configuration settings, such as the number of worker processes, to accommodate the expected load.
- Adjust PHP settings, such as memory limits and execution times, based on the requirements of your application.
Implementing these strategies will help you achieve better database performance and a more responsive bioinformatics web application. Regularly test and profile your application to identify areas for improvement and keep abreast of emerging optimization techniques.
Testing and Debugging
Testing and debugging are crucial phases in the development lifecycle of a bioinformatics web application. Here are techniques for testing the application and debugging PHP code for common issues:
1. Testing Techniques:
- Unit Testing:
- Use unit testing frameworks like PHPUnit to test individual units of code (functions or methods) in isolation.
- Write test cases for different scenarios, including edge cases and expected behaviors.
- Integration Testing:
- Perform integration testing to ensure that different components of the application work well together.
- Test interactions between database queries, PHP scripts, and external services.
- Functional Testing:
- Conduct functional testing to verify that the application functions as expected from a user perspective.
- Test user interactions, form submissions, and key features of the bioinformatics application.
- End-to-End Testing:
- Implement end-to-end testing to simulate user journeys through the application.
- Use tools like Selenium or Cypress for browser automation to test the entire application stack.
- Performance Testing:
- Perform performance testing to evaluate the application’s responsiveness and scalability under different loads.
- Use tools like Apache JMeter or Gatling to simulate concurrent users and measure response times.
- Security Testing:
- Conduct security testing to identify vulnerabilities, such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Use security testing tools or services to scan for common security issues.
- User Acceptance Testing (UAT):
- Engage stakeholders and end-users in user acceptance testing to ensure the application meets their expectations.
- Gather feedback on usability, functionality, and any potential improvements.
2. Debugging PHP Code:
- Error Reporting:
- Enable error reporting in your development environment to display PHP errors and warnings.
- Set
error_reporting(E_ALL)
andini_set('display_errors', 1)
in your PHP scripts or configure these settings in yourphp.ini
file.
- Logging:
- Implement logging to capture detailed information about the execution flow, variable values, and potential errors.
- Use the
error_log()
function to write messages to the PHP error log or implement a logging library.
- Xdebug:
- Install and configure Xdebug, a powerful PHP extension for debugging.
- Xdebug provides features like step debugging, variable inspection, and profiling.
- Print and Echo Statements:
- Insert
print
orecho
statements strategically in your code to output variable values and trace the program’s execution. - Use these statements to identify the flow of control and inspect the values of variables.
- Insert
- Use a Debugger:
- Utilize an integrated development environment (IDE) with built-in debugging capabilities.
- IDEs like PhpStorm, Visual Studio Code, and Eclipse support debugging features with breakpoints, step-by-step execution, and variable inspection.
- Check Server and PHP Logs:
- Examine server logs and PHP error logs for any issues or error messages.
- Logs can provide valuable information about server-level errors or misconfigurations.
- Code Reviews:
- Conduct code reviews with peers to identify potential issues, ensure code quality, and share knowledge.
- Collaborative debugging can catch errors that individual developers might overlook.
- Browser Developer Tools:
- Use browser developer tools to inspect and debug client-side issues, such as JavaScript errors or rendering problems.
- The browser console, network tab, and debugger tools can be invaluable for frontend debugging.
By employing a combination of testing techniques and effective debugging practices, you can enhance the reliability and performance of your bioinformatics web application. Regular testing and debugging contribute to the ongoing improvement of your application as it evolves over time.