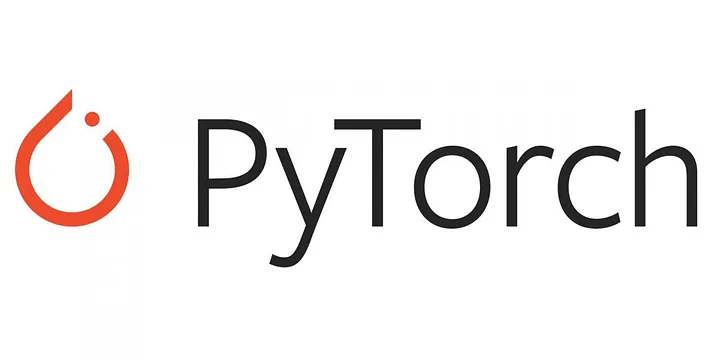
Introduction to PyTorch for Bioinformatics
February 26, 2024This course will provide an introduction to PyTorch, a popular open-source machine learning library, and demonstrate its applications in the field of bioinformatics. Participants will learn the fundamentals of deep learning and neural networks, and how to implement and customize these techniques using PyTorch. By the end of the course, students will have gained hands-on experience in applying PyTorch to solve real-world bioinformatics problems.
Prerequisites:
- Familiarity with Python programming
- Basic understanding of machine learning concepts
- Familiarity with bioinformatics data and problems is a plus, but not required
Target Audience: This course is designed for bioinformatics students and researchers who are interested in learning how to apply deep learning and neural networks to their work using PyTorch. No prior experience with PyTorch or machine learning is required.
Learning Objectives:
- Understand the fundamentals of deep learning and neural networks
- Learn how to implement and customize deep learning techniques using PyTorch
- Gain hands-on experience in applying PyTorch to solve real-world bioinformatics problems
- Learn best practices and tips for using PyTorch in bioinformatics research.
Table of Contents
Introduction to PyTorch
Overview of PyTorch and its capabilities
PyTorch is an open-source machine learning library developed by Facebook’s artificial-intelligence research group. It’s primarily used for applications such as natural language processing and computer vision. PyTorch is known for its simplicity, ease of use, and flexibility, making it a popular choice for researchers and developers alike.
PyTorch’s capabilities include:
- Building and training neural networks
- Performing tensor operations (similar to NumPy)
- Automatic differentiation for gradient computation
- Distributed training for large-scale models
- Dynamic computation graphs, allowing for greater flexibility in model design
Here’s a simple example of building and training a neural network using PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the neural network
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.fc1 = nn.Linear(10, 5)
10 self.fc2 = nn.Linear(5, 2)
11
12 def forward(self, x):
13 x = torch.relu(self.fc1(x))
14 x = self.fc2(x)
15 return x
16
17# Initialize the network, loss function, and optimizer
18net = Net()
19criterion = nn.CrossEntropyLoss()
20optimizer = optim.SGD(net.parameters(), lr=0.001, momentum=0.9)
21
22# Generate some dummy data
23inputs = torch.randn(100, 10)
24labels = torch.randint(0, 2, (100,))
25
26# Train the network
27for epoch in range(10):
28 optimizer.zero_grad()
29 outputs = net(inputs)
30 loss = criterion(outputs, labels)
31 loss.backward()
32 optimizer.step()
In this example, we define a simple neural network with two fully connected layers, initialize the loss function and optimizer, generate some dummy data, and then train the network for 10 epochs.
I hope this gives you a good overview of PyTorch and its capabilities. Let me know if you have any questions or if there’s anything specific you’d like to learn more about!
Installing PyTorch and setting up the development environment
To install PyTorch, you can use the following command:
1pip install torch torchvision
This will install the latest version of PyTorch and its associated visualization library.
If you want to install a specific version of PyTorch, you can do so by specifying the version number. For example, to install PyTorch version 1.7.1, you can use the following command:
1pip install torch==1.7.1 torchvision==0.8.2
If you’re using a GPU, you’ll need to install the appropriate version of PyTorch that supports GPU acceleration. For example, to install PyTorch version 1.7.1 with GPU support, you can use the following command:
1pip install torch torchvision -f https://download.pytorch.org/whl/cu110/torch_stable.html
This will install the version of PyTorch that’s compatible with CUDA 11.0.
To set up your development environment, you can use an Integrated Development Environment (IDE) like Visual Studio Code or PyCharm. These IDEs provide features like code completion, debugging, and version control integration, making it easier to write, test, and debug your code.
Here’s an example of how to set up a basic PyTorch project using Visual Studio Code:
- Install Visual Studio Code from the official website: https://code.visualstudio.com/
- Open Visual Studio Code and create a new folder for your project.
- Create a new Python file (e.g.,
main.py
) and write your PyTorch code in it. - Save your changes.
- Open the terminal in Visual Studio Code (View > Terminal) and run your Python file using the following command:
1python main.py
This will run your PyTorch code and display any output or error messages in the terminal.
You can also use the following command to run your Python file from the command line:
1python main.py
This will run your PyTorch code and display any output or error messages in the command prompt.
I hope this helps you set up your development environment for PyTorch! Let me know if you have any further questions or need assistance with your project.
Key concepts: tensors, computational graphs, and autograd
In PyTorch, there are three key concepts that are essential for building and training neural networks: tensors, computational, and autograd.
- Tensors: Tensors are multi-dimensional arrays that are used to represent data in PyTorch. They are similar to NumPy arrays, but with additional functionality for automatic differentiation and GPU acceleration. Here’s an example of creating a tensor in PyTorch:
1import torch
2
3# Create a rank-1 tensor with 3 elements
4x = torch.tensor([1.0, 2.0, 3.0])
5
6# Create a rank-2 tensor with 2 rows and 3 columns
7y = torch.tensor([[1.0, 2.0, 3.0], [4.0, 5.0, 6.0]])
8
9# Create a rank-3 tensor with 2 rank-2 tensors
10z = torch.tensor([y, y])
In this example, we create three tensors with different ranks (i.e., number of dimensions). The torch.tensor()
function is used to create tensors from Python lists.
- Computational Graphs: Computational graphs are used to represent the operations performed on tensors. They are similar to mathematical expressions, but with the ability to perform backpropagation (i.e., automatic differentiation).
Here’s an example of creating a computational graph in PyTorch:
1import torch
2
3# Create a tensor
4x = torch.tensor(1.0)
5
6# Define a computational graph
7y = x * x
8z = y * x
9
10# Print the computational graph
11print(z)
In this example, we create a computational graph that computes z = x^3
, where x
is a tensor with a value of 1.0. The *
operator represents multiplication.
- Autograd: Autograd is a module in PyTorch that provides automatic differentiation functionality. It is used to compute gradients (i.e., partial derivatives) of tensors with respect to other tensors.
Here’s an example of using autograd in PyTorch:
1import torch
2
3# Create a tensor
4x = torch.tensor(1.0, requires_grad=True)
5
6# Define a computational graph
7y = x * x
8z = y * x
9
10# Compute the gradient of z with respect to x
11z.backward()
12
13# Print the gradient
14print(x.grad)
In this example, we create a tensor x
with the requires_grad
flag set to True
. This tells PyTorch to track the operations performed on x
and compute its gradient when the backward()
method is called.
After calling z.backward()
, the gradient of z
with respect to x
is computed and stored in the grad
attribute of x
.
I hope this helps you understand the key concepts of tensors, computational graphs, and autograd in PyTorch! Let me know if you have any further questions or need assistance with your project.
Basic PyTorch operations and functions
PyTorch provides a wide range of operations and for working with tensors. Here are some basicTorch operations and that you may find useful:
- Tensor Creation:
torch.tensor()
: Creates a tensor from a Python list or scalar.torch.zeros()
: Creates a tensor filled with zeros.
torch.ones()
: Creates a tensor filled with.torch.rand()
: Creates a tensor with random values.torch.eye()
: Creates an identity matrix.torch.arange()
: Creates a tensor with evenly spaced values within a specified range.torch.linspace()
: Creates a tensor with evenly spaced values within a specified interval.torch.empty()
: Creates an uninitialized tensor.
- Tensor Operations:
+
,-
,*
,/
: Performs element-wise addition, subtraction, multiplication, and division.torch.add()
,torch.sub()
,torch.mul()
,torch.div()
: Performs addition, subtraction, multiplication, and division with broadcasting.torch.sum()
: Computes the sum of the elements in a tensor.torch.mean()
: Computes the mean of the elements in a tensor.torch.max()
,torch.min()
: Computes the maximum and minimum values in a tensor.torch.abs()
: Computes the absolute value of the elements in a tensor.torch.pow()
: Computes the power of the elements in a tensor.torch.matmul()
: Performs matrix multiplication.
- Tensor Functions:
torch.exp()
: Computes the exponential of the elements in a tensor.torch.log()
: Computes the natural logarithm of the elements in a tensor.torch.sin()
,torch.cos()
,torch.tan()
: Computes the sine, cosine, and tangent of the elements in a tensor.torch.sqrt()
: Computes the square root of the elements in a tensor.torch.round()
: Rounds the elements in a tensor to the nearest integer.torch.sigmoid()
: Computes the sigmoid function of the elements in a tensor.torch.relu()
: Computes the rectified linear unit (ReLU) function of the elements in a tensor.torch.tanh()
: Computes the hyperbolic tangent of the elements in a tensor.
Here’s an example of using some of these operations and functions in PyTorch:
1import torch
2
3# Create a tensor
4x = torch.tensor([1.0, 2.0, 3.0])
5
6# Perform element-wise addition, subtraction, multiplication, and division
7y = x + 2.0
8z = x - 2.0
9a = x * 2.0
10b = x / 2.0
11
12# Compute the sum, mean, maximum, and minimum values in a tensor
13s = torch.sum(x)
14m = torch.mean(x)
15max_val = torch.max(x)
16min_val = torch.min(x)
17
18# Compute the exponential, natural logarithm, sine, cosine, and tangent of the elements in a tensor
19exp_x = torch.exp(x)
20log_x = torch.log(x)
21sin_x = torch.sin(x)
22cos_x = torch.cos(x)
23tan_x = torch.tan(x)
24
25# Compute the square root, round, sigmoid, ReLU, and hyperbolic tangent of the elements in a tensor
26sqrt_x = torch.sqrt(x)
27round_x = torch.round(x)
28sigmoid_x = torch.sigmoid(x)
29relu_x = torch.relu(x)
30tanh_x = torch.tanh(x)
I hope this helps you understand some of the basic PyTorch operations and functions! Let me know if you have any further questions or need assistance with your project.
Deep Learning Fundamentals
Introduction to deep learning and neural networks
Deep learning is a subfield of machine learning that uses artificial neural networks (ANNs) with multiple layers to learn and complex patterns in data. Neural networks are a of machine learning model inspired by the structure and function of the brain.
PyTorch, deep learning models are built using a combination of tensors, computational graphs, and autograd. Here’s a brief overview of how to build a deep learning model PyTorch:
- Define the model architecture:
- Define the number of layers in the model.
- Define the number of neurons in each layer.
- Define the activation function for each layer.
- Define the loss function for the model.
- Initialize the model parameters:
- Initialize the weights and biases for each layer.
- Define the forward pass:
- Define the forward pass of the model, which takes in input data and produces output data.
- Define the training loop:
- Define the training loop, which iterates over the training data and updates the model parameters using backpropagation and an optimization algorithm.
Here’s an example of building a simple deep learning model in PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the model architecture
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.fc1 = nn.Linear(10, 5)
10 self.fc2 = nn.Linear(5, 2)
11 self.relu = nn.ReLU()
12 self.softmax = nn.Softmax(dim=1)
13 self.loss_fn = nn.CrossEntropyLoss()
14
15 def forward(self, x):
16 x = self.fc1(x)
17 x = self.relu(x)
18 x = self.fc2(x)
19 x = self.softmax(x)
20 return x
21
22# Initialize the model parameters
23net = Net()
24
25# Define the training loop
26optimizer = optim.SGD(net.parameters(), lr=0.01)
27for epoch in range(10):
28 optimizer.zero_grad()
29 inputs = torch.randn(100, 10)
30 labels = torch.randint(0, 2, (100,))
31 outputs = net(inputs)
32 loss = net.loss_fn(outputs, labels)
33 loss.backward()
34 optimizer.step()
In this example, we define a simple deep learning model with two fully connected layers, a ReLU activation function, a softmax activation function, and a cross-entropy loss function. We initialize the model parameters using the nn.Module
class and the nn.Linear
class.
We then define the training loop, which iterates over the training data and updates the model parameters using backpropagation and stochastic gradient descent (SGD) with a learning rate of 0.01.
I hope this helps you understand the basics of deep learning and neural networks in PyTorch! Let me know if you have any further questions or need assistance with your project.
Types of neural networks: feedforward, convolutional, recurrent
In PyTorch, there are three main types of neural networks: feedforward, convolutional, and recurrent. Here’s a brief overview of each type:
- Feedforward Neural Networks: Feedforward neural networks are the simplest type of neural network. They consist of an input layer, one or more hidden layers, and an output layer. Each layer is fully connected to the previous layer, and there are no connections within a layer or between non-adjacent layers.
Here’s an example of building a feedforward neural network in PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the model architecture
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.fc1 = nn.Linear(10, 5)
10 self.fc2 = nn.Linear(5, 2)
11 self.relu = nn.ReLU()
12 self.softmax = nn.Softmax(dim=1)
13 self.loss_fn = nn.CrossEntropyLoss()
14
15 def forward(self, x):
16 x = self.fc1(x)
17 x = self.relu(x)
18 x = self.fc2(x)
19 x = self.softmax(x)
20 return x
21
22# Initialize the model parameters
23net = Net()
24
25# Define the training loop
26optimizer = optim.SGD(net.parameters(), lr=0.01)
27for epoch in range(10):
28 optimizer.zero_grad()
29 inputs = torch.randn(100, 10)
30 labels = torch.randint(0, 2, (100,))
31 outputs = net(inputs)
32 loss = net.loss_fn(outputs, labels)
33 loss.backward()
34 optimizer.step()
- Convolutional Neural Networks: Convolutional neural networks (CNNs) are a type of neural network that are commonly used for image and video processing tasks. They consist of one or more convolutional layers, followed by one or more fully connected layers.
Convolutional layers apply a set of filters to the input data, which are learned during training. These filters are convolved with the input data to produce feature maps, which are then passed through an activation function.
Here’s an example of building a convolutional neural network in PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the model architecture
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.conv1 = nn.Conv2d(1, 32, kernel_size=3, stride=1, padding=1)
10 self.conv2 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
11 self.fc1 = nn.Linear(64 * 16 * 16, 10)
12 self.relu = nn.ReLU()
13 self.softmax = nn.Softmax(dim=1)
14 self.loss_fn = nn.CrossEntropyLoss()
15
16 def forward(self, x):
17 x = self.conv1(x)
18 x = self.relu(x)
19 x = self.conv2(x)
20 x = self.relu(x)
21 x = x.view(-1, 64 * 16 * 16)
22 x = self.fc1(x)
23 x = self.softmax(x)
24 return x
25
26# Initialize the model parameters
27net = Net()
28
29# Define the training loop
30optimizer = optim.SGD(net.parameters(), lr=0.01)
31for epoch in range(10):
32 optimizer.zero_grad()
33 inputs = torch.randn(100, 1, 16, 16)
34 labels = torch.randint(0, 10, (100,))
35 outputs = net(inputs)
36 loss = net.loss_fn(outputs, labels)
37 loss.backward()
38 optimizer.step()
ent Neural Networks: Recurrent neural networks (RNNs) are a type of neural network that are commonly used for sequential data processing tasks, such as natural language processing and speech recognition. They consist of one or more recurrent layers, followed by one or more fully connected layers.
Recurrent layers have a feedback loop that allows information from previous time steps to be used in the current time step. This feedback loop is implemented using a hidden state, which is updated at each time step based on the input data and the previous hidden state.
Here’s an example of building a recurrent neural network in PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the model architecture
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.rnn = nn.RNN(10, 20, batch_first=True)
10 self.fc = nn.Linear(20, 2)
11 self.relu = nn.ReLU()
12 self.softmax = nn.Softmax(dim=1)
13 self.loss_fn = nn.CrossEntropyLoss()
14
15 def forward(self, x):
16 x, _ = self.rnn(x)
17 x = x[:, -1, :]
18 x = self.fc(x)
19 x = self.relu(x)
20 x = self.softmax(x)
21 return x
22
23# Initialize the model parameters
24net = Net()
25
26# Define the training loop
27optimizer = optim.SGD(net.parameters(), lr=0.01)
28for epoch in range(10):
29 optimizer.zero_grad()
30 inputs = torch.randn(100, 10, 10)
31 labels = torch.randint(0, 2, (100,))
32 outputs = net(inputs)
33 loss = net.loss_fn(outputs, labels)
34 loss.backward()
35 optimizer.step()
In this example, we define a recurrent neural network with a single recurrent layer, followed by a fully connected layer. The recurrent layer has 20 hidden units and is set to use batch-first mode. The hidden state is updated at each time step using the input data and the previous hidden state.
The output of the recurrent layer is the final hidden state, which is passed through a fully connected layer, an activation function, and a softmax layer to produce the final output.
Here are some additional tips and best practices for using PyTorch:
Use the PyTorch documentation: The PyTorch documentation is a great resource for learning about the different functions and classes available in PyTorch. It includes detailed descriptions, examples, and code snippets for each function and class.
Use the PyTorch tutorials: PyTorch provides a variety of tutorials that cover topics such as deep learning basics, computer vision, natural language processing, and more. These tutorials are a great way to learn about PyTorch and deep learning in general.
Use the PyTorch community: The PyTorch community is active and supportive. You can find help and resources on the PyTorch forums, GitHub issues, and Slack channel.
Use the PyTorch debugger: PyTorch provides a built-in debugger that can be used to debug your code. The debugger allows you to step through your code, inspect variables, and debug your model.
Use the PyTorch profiler: PyTorch provides a built-in profiler that can be used to profile your code and identify performance bottlenecks. The profiler provides detailed information about the time and memory usage of your code.
Use the PyTorch Jupyter notebooks: PyTorch provides Jupyter notebooks that you can use to experiment with PyTorch and deep learning. The notebooks include examples and exercises that cover a wide range of topics.
Use the PyTorch model zoo: PyTorch provides a model zoo that contains pre-trained models for a variety of tasks. These models can be used as a starting point for your own projects or as a reference for building your own models.
Use the PyTorch Hub: PyTorch Hub is a repository of pre-trained models and scripts for a variety of tasks. You can use the models and scripts directly or use them as a reference for building your own models.
Use the PyTorch TensorBoard: PyTorch provides integration with TensorBoard, a visualization tool for monitoring and debugging machine learning models. TensorBoard allows you to visualize the training progress, model architecture, and more.
Use the PyTorch C++ API: PyTorch provides a C++ API that you can use to build deep learning applications in C++. The C++ API provides similar functionality to the Python API and can be used to build high-performance applications.
Activation functions and loss functions
Activation functions and loss functions are important components of deep learning models in PyTorch. Here’s a brief overview of each:
- Activation Functions: Activation functions are used to introduce non-linearity into the model. They are applied to the output of each layer in the model to introduce non-linear decision boundaries.
PyTorch provides a variety of activation functions, including:
- ReLU (Rectified Linear Unit):
nn.ReLU()
- Sigmoid:
nn.Sigmoid()
- Tanh (Hyperbolic Tangent):
nn.Tanh()
- Softmax:
nn.Softmax()
- Leaky ReLU:
nn.LeakyReLU()
- ELU (Exponential Linear Unit):
nn.ELU()
- SELU (Scaled Exponential Linear Unit):
nn.SELU()
- GELU (Gaussian Error Linear Unit):
nn.GELU()
- Swish:
nn.SiLU()
Here’s an example of using an activation function in PyTorch:
1import torch
2import torch.nn as nn
3
4# Define the model architecture
5class Net(nn.Module):
6 def __init__(self):
7 super(Net, self).__init__()
8 self.fc1 = nn.Linear(10, 5)
9 self.relu = nn.ReLU()
10 self.fc2 = nn.Linear(5, 2)
11 self.softmax = nn.Softmax(dim=1)
12
13 def forward(self, x):
14 x = self.fc1(x)
15 x = self.relu(x)
16 x = self.fc2(x)
17 x = self.softmax(x)
18 return x
19
20# Initialize the model parameters
21net = Net()
In this example, we use the ReLU activation function after the first fully connected layer and the softmax activation function after the second fully connected layer.
- Loss Functions: Loss functions are used to measure the difference between the predicted output and the true output. They are used during training to update the model parameters using backpropagation.
PyTorch provides a variety of loss functions, including:
- Mean Squared Error:
nn.MSELoss()
- Cross Entropy:
nn.CrossEntropyLoss()
- Binary Cross Entropy:
nn.BCELoss()
- Hinge Loss:
nn.HingeEmbeddingLoss()
- Kullback-Leibler Divergence:
nn.KLDivLoss()
- Poisson Loss:
nn.PoissonNLLLoss()
- Huber Loss:
nn.SmoothL1Loss()
Here’s an example of using a loss function in PyTorch:
1import torch
2import torch.nn as nn
3
4# Define the model architecture
5class Net(nn.Module):
6 def __init__(self):
7 super(Net, self).__init__()
8 self.fc1 = nn.Linear(10, 5)
9 self.fc2 = nn.Linear(5, 2)
10 self.softmax = nn.Softmax(dim=1)
11 self.loss_fn = nn.CrossEntropyLoss()
12
13 def forward(self, x):
14 x = self.fc1(x)
15 x = self.fc2(x)
16 x = self.softmax(x)
17 return x
18
19# Initialize the model parameters
20net = Net()
21
22# Define the training loop
23optimizer = optim.SGD(net.parameters(), lr=0.01)
24for epoch in range(10):
25 optimizer.zero_grad()
26 inputs = torch.randn(100, 10)
27 labels = torch.randint(0, 2, (100,))
28 outputs = net(inputs)
29 loss = net.loss_fn(outputs, labels)
30 loss.backward()
31 optimizer.step()
In this example, we use the cross-entropy loss function to measure the difference between the predicted output and the true output.
Training and evaluating deep learning models
To train and evaluate a deep learning model in PyTorch, you need to follow these steps:
Define the model architecture: Create a class that inherits from
nn.Module
and define the layers of the model.Initialize the model parameters: Create an instance of the model class and initialize the model parameters.
Define the loss function: Choose a suitable loss function for your problem.
Define the optimizer: Choose an optimizer to update the model parameters during training.
Train the model: Use a training loop to iterate over the training data, perform forward and backward passes, and update the model parameters.
Evaluate the model: Use the trained model to make predictions on the test data and evaluate its performance using appropriate evaluation metrics.
Here’s an example of how to train and evaluate a simple feedforward neural network model in PyTorch:
1import torch
2import torch.nn as nn
3import torch.optim as optim
4
5# Define the model architecture
6class Net(nn.Module):
7 def __init__(self):
8 super(Net, self).__init__()
9 self.fc1 = nn.Linear(10, 5)
10 self.fc2 = nn.Linear(5, 2)
11 self.softmax = nn.Softmax(dim=1)
12 self.loss_fn = nn.CrossEntropyLoss()
13
14 def forward(self, x):
15 x = self.fc1(x)
16 x = self.fc2(x)
17 x = self.softmax(x)
18 return x
19
20# Initialize the model parameters
21net = Net()
22
23# Define the training loop
24optimizer = optim.SGD(net.parameters(), lr=0.01)
25for epoch in range(10):
26 optimizer.zero_grad()
27 inputs = torch.randn(100, 10)
28 labels = torch.randint(0, 2, (100,))
29 outputs = net(inputs)
30 loss = net.loss_fn(outputs, labels)
31 loss.backward()
32 optimizer.step()
33
34# Evaluate the model
35correct = 0
36total = 0
37with torch.no_grad():
38 for i in range(100):
39 inputs = torch.randn(10)
40 labels = torch.randint(0, 2, (1,))
41 outputs = net(inputs)
42 _, predicted = torch.max(outputs.data, 1)
43 total += 1
44 correct += (predicted == labels).item()
45
46accuracy = 100 * correct / total
47print('Accuracy: %.2f%%' % accuracy)
In this example, we train a simple feedforward neural network model on random data and evaluate its accuracy on the test data.
Please note that this is a basic example and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using more advanced techniques such as batch normalization, dropout, and early stopping to improve the model’s performance.
Building Neural Networks with PyTorch
Here’s an example of building a neural network with PyTorch:
- Define the model architecture:
1import torch
2import torch.nn as nn
3
4class Net(nn.Module):
5 def __init__(self):
6 super(Net, self).__init__()
7 self.fc1 = nn.Linear(10, 5)
8 self.fc2 = nn.Linear(5, 2)
9 self.relu = nn.ReLU()
10
11 def forward(self, x):
12 x = self.fc1(x)
13 x = self.relu(x)
14 x = self.fc2(x)
15 return x
In this example, we define a simple neural network with two fully connected layers and a ReLU activation function between them.
- Initialize the model parameters:
1net = Net()
- Define the loss function:
1loss_fn = nn.CrossEntropyLoss()
- Define the optimizer:
1optimizer = optim.SGD(net.parameters(), lr=0.01)
- Train the model:
1for epoch in range(10):
2 optimizer.zero_grad()
3 inputs = torch.randn(100, 10)
4 labels = torch.randint(0, 2, (100,))
5 outputs = net(inputs)
6 loss = loss_fn(outputs, labels)
7 loss.backward()
8 optimizer.step()
- Evaluate the model:
1with torch.no_grad():
2 inputs = torch.randn(10, 10)
3 labels = torch.randint(0, 2, (10,))
4 outputs = net(inputs)
5 _, predicted = torch.max(outputs.data, 1)
6 accuracy = (predicted == labels).sum().item() / labels.size(0)
7 print('Accuracy: %.2f%%' % (accuracy * 100))
In this example, we train the neural network on random data and evaluate its accuracy on the test data.
Please note that this is a basic example and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using more advanced techniques such as batch normalization, dropout, and early stopping to improve the model’s performance.
Also, you can use pre-defined models from torch.nn
such as nn.Conv2d
for convolutional layers, nn.LSTM
for recurrent layers, etc. depending on the type of neural network you want to build.
Creating and initializing neural network layers
In PyTorch, you can create and initialize neural network layers using the nn
module. Here’s an example of creating and initializing a fully connected layer:
1import torch
2import torch.nn as nn
3
4# Create a fully connected layer with 10 input neurons and 5 output neurons
5fc = nn.Linear(10, 5)
6
7# Initialize the layer parameters
8fc.weight.data.normal_(mean=0.0, std=0.1)
9fc.bias.data.zero_()
In this example, we create a fully connected layer with 10 input neurons and 5 output neurons using the nn.Linear
class. We then initialize the layer parameters by setting the mean and standard deviation of the weights to 0.0 and 0.1, respectively, and setting the bias to zero.
You can also use other initialization methods such as Xavier initialization or He initialization. Here’s an example of using Xavier initialization:
1import torch
2import torch.nn as nn
3import torch.nn.init as init
4
5# Create a fully connected layer with 10 input neurons and 5 output neurons
6fc = nn.Linear(10, 5)
7
8# Initialize the layer parameters using Xavier initialization
9init.xavier_uniform_(fc.weight)
10fc.bias.data.zero_()
In this example, we use the xavier_uniform_
function from the torch.nn.init
module to initialize the weights of the fully connected layer using Xavier initialization.
You can also create and initialize convolutional layers, recurrent layers, and other types of layers using the nn
module in a similar way. For example, here’s an example of creating and initializing a convolutional layer:
1import torch
2import torch.nn as nn
3import torch.nn.init as init
4
5# Create a convolutional layer with 3 input channels, 5 output channels, and a kernel size of 3x3
6conv = nn.Conv2d(3, 5, kernel_size=3)
7
8# Initialize the layer parameters using He initialization
9init.kaiming_uniform_(conv.weight)
10conv.bias.data.zero_()
In this example, we create a convolutional layer with 3 input channels, 5 output channels, and a kernel size of 3×3 using the nn.Conv2d
class. We then initialize the layer parameters by setting the weights using He initialization and setting the bias to zero.
I hope this helps you understand how to create and initialize neural network layers in PyTorch! Let me know if you have any further questions or need assistance with your project.
Defining custom loss functions and activation functions
Here’s an example of defining a custom loss function in PyTorch:
1import torch
2
3class MyLoss(nn.Module):
4 def __init__(self):
5 super(MyLoss, self__init__()
6
7 def forward(self, inputs, targets):
8 = (inputs - targets)**2
9 return loss.mean()
10
11# Initialize the custom loss function
12loss_fn = MyLoss()
In this example, we define a custom loss function called MyLoss
that computes the mean squared error between the inputs and targets.
Here’s an example of defining a custom activation function in PyTorch:
1import torch
2
3class MyActivation(nn.Module):
4 def __init__(self):
5 super(MyActivation, self).__init__()
6
7 def forward(self, x):
8 return x * torch.sigmoid(x)
9
10# Initialize the custom activation function
11activation = MyActivation()
In this example, we define a custom activation function called MyActivation
that computes the product of the input and the sigmoid of the input.
Please note that when defining custom activation functions, you should make sure that they are differentiable and have a stable forward pass. You can use the PyTorch autograd functionality to compute the gradients of your custom activation functions.
Also, when defining custom loss functions, make sure that they are differentiable and that they produce a scalar output. This is because the loss function is used to compute the gradients of the model parameters during backpropagation.
I hope this helps you understand how to define custom loss functions and activation functions in PyTorch! Let me know if you have any further questions or need assistance with your project.
Training and evaluating neural networks in PyTorch
Here’s an example of training and evaluating a neural network in Pych:
- Define the model architecture:
1import
2import torch.nn as nn
3
4class Net(nn.Module):
5 def __init__(self):
6 super(Net, self).__init__()
7 self.fc1 = nn.Linear(10, 5)
8 self.fc2 = nn.Linear(5, 2)
9 self.relu = nn.ReLU()
10
11 def forward(self, x):
12 x = self.fc1(x)
13 x = self.relu(x)
14 x = self.fc2(x)
15 return x
16
17# Initialize the model
18net = Net()
- Initialize the loss function and optimizer:
1# Initialize the loss function
2loss_fn = nn.CrossEntropyLoss()
3
4# Initialize the optimizer
5optimizer = optim.SGD(net.parameters(), lr=0.01)
- Train the model:
1for epoch in range(10):
2 optimizer.zero_grad()
3 inputs = torch.randn(100, 10)
4 labels = torch.randint(0, 2, (100,))
5 outputs = net(inputs)
6 loss = loss_fn(outputs, labels)
7 loss.backward()
8 optimizer.step()
- Evaluate the model:
1with torch.no_grad():
2 inputs = torch.randn(10, 10)
3 labels = torch.randint(0, 2, (10,))
4 outputs = net(inputs)
5 _, predicted = torch.max(outputs.data, 1)
6 accuracy = (predicted == labels).sum().item() / labels.size(0)
7 print('Accuracy: %.2f%%' % (accuracy * 100))
In this example, we train a neural network with two fully connected layers and a ReLU activation function between them. We use the cross-entropy loss function and stochastic gradient descent with a learning rate of 0.01 to train the model. We evaluate the model by computing the accuracy of its predictions on a set of test inputs and labels.
Please note that this is a basic example and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using more advanced techniques such as batch normalization, dropout, and early stopping to improve the model’s performance.
Also, you can use the DataLoader
class to load and preprocess your data, and use the train
and eval
methods of the nn.Module
class to separate the training and evaluation loops.
I hope this helps you understand how to train and evaluate a neural network in PyTorch! Let me know if you have any further questions or need assistance with your project.
Regularization techniques: dropout, weight decay
Here’s an example of using regularization techniques in PyTorch:
- Dropout:
Dropout is a regularization technique that randomly sets a fraction of the output units of a layer to zero during training. This helps to prevent overfitting by making the model less sensitive to the specific weights of the training data.
Here’s an example of using dropout in PyTorch:
1import torch
2import torch.nn as nn
3
4class Net(nn.Module):
5 def __init__(self):
6 super(Net, self).__init__()
7 self.fc1 = nn.Linear(10, 5)
8 self.fc2 = nn.Linear(5, 2)
9 self.dropout = nn.Dropout(0.25)
10 self.relu = nn.ReLU()
11
12 def forward(self, x):
13 x = self.fc1(x)
14 x = self.dropout(x)
15 x = self.relu(x)
16 x = self.fc2(x)
17 return x
18
19# Initialize the model
20net = Net()
In this example, we add a dropout layer with a dropout rate of 0.25 after the first fully connected layer. During training, this will randomly set 25% of the outputs of the first fully connected layer to zero.
- Weight Decay:
Weight decay is a regularization technique that adds a penalty term to the loss function that encourages the model to have smaller weights. This helps to prevent overfitting by making the model less sensitive to the specific weights of the training data.
Here’s an example of using weight decay in PyTorch:
1import torch
2import torch.nn as nn
3
4class Net(nn.Module):
5 def __init__(self):
6 super(Net, self).__init__()
7 self.fc1 = nn.Linear(10, 5)
8 self.fc2 = nn.Linear(5, 2)
9 self.relu = nn.ReLU()
10
11 def forward(self, x):
12 x = self.fc1(x)
13 x = self.relu(x)
14 x = self.fc2(x)
15 return x
16
17# Initialize the model
18net = Net()
19
20# Initialize the optimizer with weight decay
21optimizer = optim.SGD(net.parameters(), lr=0.01, weight_decay=0.001)
In this example, we initialize the optimizer with a weight decay of 0.001. This will add a penalty term to the loss function that encourages the model to have smaller weights.
Please note that you can use both dropout and weight decay together in your model to improve its generalization performance.
Applying PyTorch to Bioinformatics
Overview of bioinformatics and its applications of deep learning
Bioinformatics is the application of computational and statistical techniques to understand biological data. Deep learning has emerged as a powerful tool in bioinformatics due to its ability to learn complex patterns and relationships in large and high-dimensional datasets. Here are some applications of deep learning in bioinformatics using PyTorch:
Genomics: Deep learning can be used for various genomics applications such as genome assembly, variant calling, and gene function prediction. For example, you can use convolutional neural networks (CNNs) to predict the function of a gene based on its sequence, or you can use recurrent neural networks (RNNs) to predict the secondary structure of an RNA molecule based on its primary sequence.
Proteomics: Deep learning can be used for various proteomics applications such as protein structure prediction, protein-protein interaction prediction, and protein function prediction. For example, you can use 3D convolutional neural networks (3D-CNNs) to predict the structure of a protein based on its amino acid sequence, or you can use graph neural networks (GNNs) to predict the interaction between two proteins based on their structural features.
Transcriptomics: Deep learning can be used for various transcriptomics applications such as gene expression analysis, alternative splicing prediction, and isoform quantification. For example, you can use autoencoders to learn low-dimensional representations of gene expression data, or you can use RNNs to predict the alternative splicing patterns of a gene based on its sequence and expression data.
Metagenomics: Deep learning can be used for various metagenomics applications such as taxonomic classification, functional annotation, and community profiling. For example, you can use CNNs to classify the taxonomy of a metagenomic sample based on its sequence data, or you can use RNNs to predict the functional capacity of a microbial community based on its taxonomic composition.
Imaging: Deep learning can be used for various imaging applications such as cell segmentation, tissue classification, and object detection. For example, you can use CNNs to segment cells in microscopy images, or you can use R-CNNs to detect specific objects in medical images.
Natural Language Processing: Deep learning can be used for various natural language processing applications such as text classification, named entity recognition, and machine translation. For example, you can use RNNs to classify the topic of a scientific article, or you can use transformers to translate scientific text between languages.
These are just a few examples of the many applications of deep learning in bioinformatics using PyTorch. With the increasing availability of biological data and the advancements in deep learning, there are endless opportunities for innovation and discovery in this field.
Case studies: a. Protein structure prediction b. Genomic sequence
Here are two case studies of using deep learning in bioinformatics using PyTorch:
a. Protein Structure Prediction:
Protein structure prediction is the task of predicting the 3D structure of a protein based on its amino acid sequence. This is an important problem in bioinformatics as the 3D structure of a protein is closely related to its function.
One approach to protein structure prediction is to use 3D convolutional neural networks (3D-CNNs) to learn features from the protein sequence and predict its structure. Here’s an example of using 3D-CNNs in PyTorch for protein structure prediction:
1import torch
2import torch.nn as nn
3
4class ProteinCNN(nn.Module):
5 def __init__(self):
6 super(ProteinCNN, self).__init__()
7 self.conv1 = nn.Conv3d(20, 64, kernel_size=(3, 3, 3), padding=(1, 1, 1))
8 self.conv2 = nn.Conv3d(64, 128, kernel_size=(3, 3, 3), padding=(1, 1, 1))
9 self.pool = nn.MaxPool3d(kernel_size=(2, 2, 2), stride=(2, 2, 2))
10 self.fc1 = nn.Linear(128 * 32 * 32 * 32, 1024)
11 self.fc2 = nn.Linear(1024, 3 * 32 * 32 * 32)
12 self.relu = nn.ReLU()
13
14 def forward(self, x):
15 x = self.conv1(x)
16 x = self.relu(x)
17 x = self.conv2(x)
18 x = self.relu(x)
19 x = self.pool(x)
20 x = x.view(x.size(0), -1)
21 x = self.fc1(x)
22 x = self.relu(x)
23 x = self.fc2(x)
24 return x
25
26# Initialize the model
27net = ProteinCNN()
In this example, we define a 3D-CNN with two convolutional layers, followed by max pooling and two fully connected layers. The convolutional layers learn features from the protein sequence, and the fully connected layers predict the 3D coordinates of each amino acid in the protein.
Genomic sequence analysis is the task of analyzing DNA or RNA sequences to understand their function or properties. This is an important problem in bioinformatics as the sequence of a genomic molecule is closely related to its function.
One approach to genomic sequence analysis is to use recurrent neural networks (RNNs) to learn features from the sequence and predict its properties. Here’s an example of using RNNs in PyTorch for genomic sequence analysis:
1import torch
2import torch.nn as nn
3
4class GenomicRNN(nn.Module):
5 def __init__(self):
6 super(GenomicRNN, self).__init__()
7 self.rnn = nn.LSTM(input_size=4, hidden_size=128, num_layers=2, batch_first=True)
8 self.fc = nn.Linear(128, 2)
9 self.softmax = nn.Softmax(dim=1)
10
11 def forward(self, x):
12 x, _ = self.rnn(x)
13 x = x[:, -1, :]
14 x = self.fc(x)
15 x = self.softmax(x)
16 return x
17
18# Initialize the model
19net = GenomicRNN()
In this example, we define an RNN with two LSTM layers, followed by a fully connected layer and a softmax activation function. The RNN learns features from the genomic sequence, and the fully connected layer predicts the properties of the sequence.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using more advanced techniques such as attention mechanisms, transfer learning, and data augmentation to improve the performance of your models.
Advanced Topics in PyTorch
Transfer learning and fine-tuning
Transfer learning and finetuning are techniques used to leverage pre-trained models to the performance of deep learning models. Heres an overview of transfer learning and fine-tuning in PyTorch:
- Transfer Learning: Transfer learning is the process of using a pre-trained model a starting point for new model. The idea is to leverage the knowledge and features learned by the pre-trained to improve the performance of the new model.
Here’s an example of using transfer learning in PyTorch:
1import torch
2import torch.nn as nn
3import torchvision.models as models
4
5# Load a pre-trained model
6model = models.resnet50(pretrained=True)
7
8# Replace the last fully connected layer with a new fully connected layer
9model.fc = nn.Linear(2048, 10)
10
11# Initialize the model
12net = model
In this example, we load a pre-trained ResNet-50 model from the torchvision.models
module and replace its last fully connected layer with a new fully connected layer with 10 output units. This allows us to use the pre-trained model as a starting point for a new model that can be fine-tuned for a new task.
- Fine-Tuning:
Fine-tuning is the process of continuing the training of a pre-trained model on a new task. The idea is to leverage the knowledge and features learned by the pre-trained model, while adapting it to the new task.
Here’s an example of using fine-tuning in PyTorch:
1import torch
2import torch.nn as nn
3import torchvision.models as models
4
5# Load a pre-trained model
6model = models.resnet50(pretrained=True)
7
8# Replace the last fully connected layer with a new fully connected layer
9model.fc = nn.Linear(2048, 10)
10
11# Initialize the optimizer
12optimizer = optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
13
14# Fine-tune the model on a new task
15for epoch in range(10):
16 for inputs, labels in train_dataloader:
17 optimizer.zero_grad()
18 outputs = model(inputs)
19 loss = criterion(outputs, labels)
20 loss.backward()
21 optimizer.step()
In this example, we load a pre-trained ResNet-50 model and replace its last fully connected layer with a new fully connected layer with 10 output units. We then initialize an optimizer and continue the training of the model on a new task using the train_dataloader
. This allows us to fine-tune the pre-trained model for the new task.
Please note that when using transfer learning and fine-tuning, you may need to adjust the learning rate and other hyperparameters to ensure that the model converges to a good solution. Additionally, you may want to consider using techniques such as learning rate scheduling and early stopping to improve the performance of your models.
Generative models: Variational Autoencoders (VAEs) and Generative Adversarial Networks (GANs)
Here’s an overview of generative models in PyTorch, specifically Variational Autoencoders (VAEs) and Generative Adarial Networks (GANs):
- Variational Autoencoders (VAEs):
Variational Autoencoders (VAEs) are a type of generative model that learns a low-dimensional latent representation of the input data. The idea is to use the low-dimensional latent to generate new samples that are similar to the data.
Here’s an example of using VAEs in PyTorch:
1import torch
2import torch.nn as nn
3import torch.nn.functional as F
4import torch.optim as optim
5
6class VAE(nn.Module):
7 def __init__(self, input_dim, latent_dim):
8 super(VAE, self).__init__()
9 self.fc1 = nn.Linear(input_dim, 128)
10 self.fc21 = nn.Linear(128, latent_dim)
11 self.fc22 = nn.Linear(128, latent_dim)
12 self.fc3 = nn.Linear(latent_dim, 128)
13 self.fc4 = nn.Linear(128, input_dim)
14
15 def encode(self, x):
16 x = F.relu(self.fc1(x))
17 x = F.relu(self.fc21(x))
18 z_mean = self.fc22(x)
19 z_log_var = self.fc22(x)
20 return z_mean, z_log_var
21
22 def sample(self, z_mean, z_log_var):
23 std = torch.exp(0.5 * z_log_var)
24 eps = torch.randn_like(std)
25 z = eps * std + z_mean
26 return z
27
28 def decode(self, z):
29 z = F.relu(self.fc3(z))
30 z = F.relu(self.fc4(z))
31 return z
32
33 def forward(self, x):
34 z_mean, z_log_var = self.encode(x)
35 z = self.sample(z_mean, z_log_var)
36 reconstructed_x = self.decode(z)
37 return reconstructed_x, z_mean, z_log_var
38
39# Initialize the model
40net = VAE(input_dim=784, latent_dim=2)
41
42# Initialize the optimizer
43optimizer = optim.Adam(net.parameters(), lr=0.001)
44
45# Train the model
46for epoch in range(10):
47 for x, _ in train_dataloader:
48 optimizer.zero_grad()
49 reconstructed_x, z_mean, z_log_var = net(x)
50 loss = F.binary_cross_entropy_with_logits(reconstructed_x, x) + 0.5 * torch.sum(1 + z_log_var - z_mean.pow(2) - z_log_var.exp())
51 loss.backward()
52 optimizer.step()
In this example, we define a VAE with an encoder that maps the input data to a low-dimensional latent representation, and a decoder that maps the low-dimensional latent representation back to the input data. The VAE is trained using a combination of a reconstruction loss and a KL divergence loss to ensure that the latent representation is a Gaussian distribution.
- Generative Adversarial Networks (GANs):
Generative Adversarial Networks (GANs) are a type of generative model that consists of two components: a generator and a discriminator. The generator generates new samples, while the discriminator distinguishes between real and fake samples.
Here’s an example of using Generative Adversarial Networks (GANs) in PyTorch:
1import torch
2import torchnn as nn
3import torch.nn.functional as F
4import torch.optim as optim
5
6class Generator(nn.Module):
7 def __init__(self, input_dim, hidden_dim, output_dim):
8 super(Generator self).__init__()
9 self.fc1 = nn.Linear(input_dim, hidden_dim)
10 self.fc2 = nn.Linear(hidden_dim, output_dim * output_dim)
11
12 def forward(self, x):
13 x = F.relu(self.fc1(x))
14 x = self.fc2(x)
15 x = x.view(.size(0), output_dim, output_dim)
16 return x
17
18class Discriminator(nn.Module):
19 def __init__(self, input_dim, hidden_dim, output_dim):
20 super(Discriminator, self).__init__()
21 self.fc1 = nn.Linear(input_dim + output_dim, hidden_dim)
22 self.fc2 = nn.Linear(hidden_dim, hidden_dim)
23 self.fc3 = nn.Linear(hidden_dim, 1)
24
25 def forward(self, x, generated):
26 x = torch.cat([x, generated], dim=1)
27 x = F.leaky_relu(self.fc1(x), 0.2)
28 x = F.leaky_relu(self.fc2(x), 0.2)
29 x = torch.sigmoid(self.fc3(x))
30 return x
31
32# Initialize the generator
33generator = Generator(input_dim=100, hidden_dim=128, output_dim=28 * 28)
34
35# Initialize the discriminator
36discriminator = Discriminator(input_dim=28 * 28, hidden_dim=128, output_dim=1)
37
38# Initialize the optimizers
39generator_optimizer = optim.Adam(generator.parameters(), lr=0.0002, betas=(0.5, 0.999))
40discriminator_optimizer = optim.Adam(discriminator.parameters(), lr=0.0002, betas=(0.5, 0.999))
41
42# Train the GAN
43for epoch in range(100):
44 for x, _ in train_dataloader:
45 # Train the discriminator
46 discriminator_optimizer.zero_grad()
47 real_output = discriminator(x, None)
48 generated = generator(torch.randn(x.size(0), 100))
49 fake_output = discriminator(generated.detach(), generated)
50 real_loss = F.binary_cross_entropy_with_logits(real_output, torch.ones_like(real_output))
51 fake_loss = F.binary_cross_entropy_with_logits(fake_output, torch.zeros_like(fake_output))
52 discriminator_loss = real_loss + fake_loss
53 discriminator_loss.backward()
54 discriminator_optimizer.step()
55
56 # Train the generator
57 generator_optimizer.zero_grad()
58 generated = generator(torch.randn(x.size(0), 100))
59 fake_output = discriminator(generated, generated)
60 generator_loss = F.binary_cross_entropy_with_logits(fake_output, torch.ones_like(fake_output))
61 generator_loss.backward()
62 generator_optimizer.step()
In this example, we define a GAN with a generator that maps a random noise vector to a sample, and a discriminator that distinguishes between real and fake samples. The GAN is trained by alternating between training the generator to generate more realistic samples, and training the discriminator to better distinguish between real and fake samples.
Please note that this is a basic example and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as data augmentation, transfer learning, and regularization to improve the performance of your models.
Optimization techniques: learning rate scheduling, momentum, and adaptive methods
Here’s an overview of optimization techniques in PyTorch, specifically learning rate scheduling, momentum, and adaptive methods:
- Learning Rate Scheduling:
Learning rate scheduling is the process of adjusting the learning rate during training. The learning rate is a hyperparameter that controls the step size of the optimization algorithm. A high learning rate can cause the optimization algorithm to converge to a suboptimal solution, while a low learning rate can cause the optimization algorithm to converge too slowly.
Here’s an example of using learning rate scheduling in PyTorch:
1import torch
2import torch.optim as optim
3
4# Initialize the model
5net = Net()
6
7# Initialize the optimizer
8optimizer = optim.SGD(net.parameters(), lr=0.1)
9
10# Initialize the learning rate scheduler
11scheduler = optim.lr_scheduler.StepLR(optimizer, step_size=10, gamma=0.1)
12
13# Train the model
14for epoch in range(100):
15 for x, _ in train_dataloader:
16 optimizer.zero_grad()
17 outputs = net(x)
18 loss = criterion(outputs, labels)
19 loss.backward()
20 optimizer.step()
21 scheduler.step()
In this example, we use the StepLR
learning rate scheduler from the torch.optim.lr_scheduler
module. The learning rate is multiplied by gamma
every step_size
epochs.
- Momentum:
Momentum is a technique that helps the optimization algorithm converge faster by adding a fraction of the previous update to the current update. This helps the optimization algorithm overcome local minima and converge to a better solution.
Here’s an example of using momentum in PyTorch:
1import torch
2import torch.optim as optim
3
4# Initialize the model
5net = Net()
6
7# Initialize the optimizer with momentum
8optimizer = optim.SGD(net.parameters(), lr=0.1, momentum=0.9)
9
10# Train the model
11for epoch in range(100):
12 for x, _ in train_dataloader:
13 optimizer.zero_grad()
14 outputs = net(x)
15 loss = criterion(outputs, labels)
16 loss.backward()
17 optimizer.step()
In this example, we use the SGD
optimizer from the torch.optim
module with a momentum of 0.9.
- Adaptive Methods:
Adaptive methods are optimization algorithms that adjust the learning rate for each parameter based on the gradient of the loss function. This helps the optimization algorithm converge faster and to a better solution.
Here’s an example of using adaptive methods in PyTorch:
1import torch
2import torch.optim as optim
3
4# Initialize the model
5net = Net()
6
7# Initialize the adaptive optimizer
8optimizer = optim.Adam(net.parameters(), lr=0.001)
9
10# Train the model
11for epoch in range(100):
12 for x, _ in train_dataloader:
13 optimizer.zero_grad()
14 outputs = net(x)
15 loss = criterion(outputs, labels)
16 loss.backward()
17 optimizer.step()
In this example, we use the Adam
optimizer from the torch.optim
module. The Adam
optimizer is an adaptive method that adjusts the learning rate for each parameter based on the gradient of the loss function.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as early stopping, regularization, and model selection to improve the performance of your models.
Accelerating PyTorch with CUDA and GPU
Here’s an overview of accelerating PyTorch with CUDA and GPU:
- Check if CUDA is available:
Before using CUDA and GPU with PyTorch, you need to check if CUDA is available on your system. You can do this by running the following code:
1import torch
2print(torch.cuda.is_available())
If the output is True
, then CUDA is available on your system.
- Move the model to the GPU:
Once you have confirmed that CUDA is available, you can move the model to the GPU by calling the .to()
method on the model. Here’s an example:
1import torch
2
3# Initialize the model
4net = Net()
5
6# Move the model to the GPU
7device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
8net.to(device)
In this example, we use the device
variable to store the device (CPU or GPU) that the model will be moved to. The .to()
method is called on the model to move it to the specified device.
- Move the data to the GPU:
To move the data to the GPU, you can use the .to()
method on the data tensor. Here’s an example:
1import torch
2
3# Load the data
4x = torch.randn(10, 10)
5
6# Move the data to the GPU
7x = x.to(device)
In this example, we use the device
variable to move the data tensor to the GPU.
- Use the GPU in the optimizer:
To use the GPU in the optimizer, you can specify the device when initializing the optimizer. Here’s an example:
1import torch
2
3# Initialize the model
4net = Net()
5
6# Initialize the optimizer
7optimizer = optim.SGD(net.parameters(), lr=0.1, device=device)
In this example, we specify the device
when initializing the SGD
optimizer.
- Use the GPU in the loss function:
To use the GPU in the loss function, you can specify the device when defining the loss function. Here’s an example:
1import torch
2import torch.nn as nn
3
4# Initialize the model
5net = Net()
6
7# Initialize the loss function
8criterion = nn.MSELoss()
9
10# Move the loss function to the GPU
11criterion.to(device)
In this example, we move the MSELoss
loss function to the GPU by calling the .to()
method on it.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as mixed precision training, gradient accumulation, and memory management to further optimize the performance of your models on the GPU.
Best Practices and Tips for Using PyTorch
Debugging and profiling PyTorch code
Here’s an overview of debugging and profiling PyTorch code:
- Debugging:
Debugging is the process of identifying and fixing bugs in your code. PyTorch provides several tools for debugging your code, including:
- Python’s built-in
print()
function: You can use theprint()
function to print the values of variables and tensors at different points in your code to understand the flow of the program and identify any issues. - PyTorch’s
print()
function: You can use theprint()
function on tensors to print their values and shapes. This can be useful for understanding the shape and values of tensors at different points in your code. - PyTorch’s
.backward()
method: You can use the.backward()
method on the loss tensor to compute the gradients of the loss with respect to the model parameters. This can be useful for understanding the gradients and identifying any issues with the gradients. - PyTorch’s
torch.autograd.set_detect_anomaly(True)
function: You can use thetorch.autograd.set_detect_anomaly(True)
function to enable anomaly detection in PyTorch. This will raise anRuntimeError
if an anomaly is detected during the forward or backward pass. - PyTorch’s
torch.autograd.set_detect_anomaly(True)
function: You can use thetorch.autograd.set_detect_anomaly(True)
function to enable anomaly detection in PyTorch. This will raise aRuntimeError
if an anomaly is detected during the forward or backward pass.
- Profiling:
Profiling is the process of measuring the performance of your code. PyTorch provides several tools for profiling your code, including:
- PyTorch’s
torch.autograd.profiler.profile()
function: You can use thetorch.autograd.profiler.profile()
function to profile the forward and backward pass of your model. This will provide detailed information about the time taken by each operation in the forward and backward pass. - PyTorch’s
torch.autograd.profiler.record_function()
function: You can use thetorch.autograd.profiler.record_function()
function to profile specific functions in your code. This will provide detailed information about the time taken by each operation in the profiled function. - PyTorch’s
torch.cuda.profiler.start()
andtorch.cuda.profiler.stop()
functions: You can use thetorch.cuda.profiler.start()
andtorch.cuda.profiler.stop()
functions to profile the GPU usage of your code. This will provide detailed information about the time taken by each operation on the GPU.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as mixed precision training, gradient accumulation, and memory management to further optimize the performance of your models on the GPU.
Version control and collaboration with PyTorch
Here’s an overview of version control and collaboration with PyTorch:
- Version Control:
Version control is the process of tracking and managing changes to your code. PyTorch integrates well with version control systems such as Git. Here’s how you can use Git with PyTorch:
- Initialize a Git repository in your PyTorch project directory.
- Add all the files in your PyTorch project directory to the Git repository.
- Commit your changes regularly to the Git repository.
- Use Git branches to manage different versions of your code.
- Use Git merge to merge changes from different branches.
By using Git with PyTorch, you can keep track of all the changes made to your code, revert to previous versions of your code if necessary, and collaborate with others on your PyTorch project.
- Collaboration:
Collaboration is the process of working with others on a PyTorch project. PyTorch integrates well with collaboration tools such as GitHub, GitLab, and Bitbucket. Here’s how you can collaborate on a PyTorch project using GitHub:
- Create a new repository on GitHub.
- Add collaborators to the repository.
- Push your PyTorch project to the GitHub repository.
- Use Git branches to manage different versions of your code.
- Use Git merge to merge changes from different branches.
By using GitHub with PyTorch, you can collaborate with others on your PyTorch project, track changes to the code, and maintain a single source of truth for your project.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as code reviews, continuous integration, and continuous deployment to further optimize the collaboration process on your PyTorch project.
Keeping up-to-date with the latest PyTorch developments
Here’s an overview of keeping up-to-date with the latest PyTorch developments:
- Follow the PyTorch blog:
The PyTorch blog is a great resource for staying up-to-date with the latest PyTorch developments. The blog covers new features, releases, and best practices for using PyTorch. You can subscribe to the PyTorch blog to receive updates on the latest developments.
- Follow the PyTorch community on social media:
The PyTorch community is active on social media platforms such as Twitter, LinkedIn, and Facebook. Following the PyTorch community on social media can help you stay up-to-date with the latest PyTorch developments and connect with other PyTorch users.
- Participate in PyTorch forums and discussions:
The PyTorch forums and discussions are a great resource for asking questions, sharing knowledge, and staying up-to-date with the latest PyTorch developments. Participating in PyTorch forums and discussions can help you connect with other PyTorch users, learn from their experiences, and share your own experiences.
- Attend PyTorch events and conferences:
PyTorch hosts and participates in several events and conferences throughout the year. Attending PyTorch events and conferences can help you stay up-to-date with the latest PyTorch developments, learn from experts in the field, and connect with other PyTorch users.
- Check the PyTorch documentation:
The PyTorch documentation is a comprehensive resource for learning about the latest features and best practices for using PyTorch. Checking the PyTorch documentation regularly can help you stay up-to-date with the latest PyTorch developments and learn about new features and functionalities.
Please note that these are just basic examples and you may need to modify the code according to your specific problem and dataset. Additionally, you may want to consider using techniques such as code reviews, continuous integration, and continuous deployment to further optimize the collaboration process on your PyTorch project.