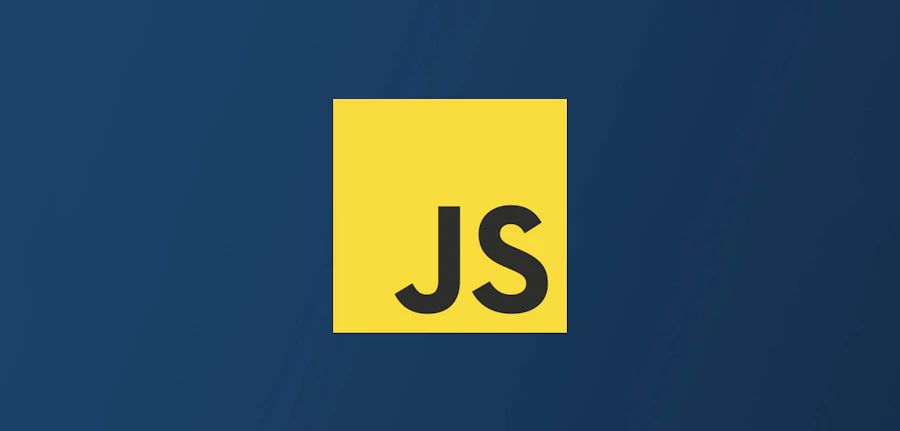
Introduction to JavaScript for Bioinformatics
February 26, 2024Table of Contents
JavaScript Basics
Welcome to our JavaScript learning session! I’m here to help you understand and master JavaScript, a powerful and versatile programming language used for web development, server-side programming, and various other applications.
First, let’s start with a basic introduction to JavaScript:
What is JavaScript? JavaScript (JS) is a high-level, dynamic, and interpreted programming language. It is primarily used for enhancing web interactivity and responsiveness by allowing developers to create dynamic content on client-side web pages.
JavaScript and the Web: JS is one of the three core technologies of the World Wide Web, alongside HTML and CSS. HTML is responsible for the structure of web pages, CSS for styling, and JavaScript for interactivity and dynamic functionality.
JavaScript Engines: Modern web browsers have built-in JavaScript engines, such as V8 (used in Google Chrome) and SpiderMonkey (used in Firefox), which interpret and execute JS code.
JavaScript Versions and Standards: JavaScript has evolved over time, with new features and improvements added in various versions. ECMAScript (ES) is the standard that defines the syntax and semantics of JavaScript. The latest version of ECMAScript is ES2022, and it is fully supported in most modern browsers.
JavaScript Outside the Browser: While JavaScript is primarily used in web development, it can also be used for server-side programming with Node.js, mobile app development with frameworks like React Native and Ionic, and even for creating desktop applications with Electron.
Now that you have a basic understanding of JavaScript, let’s dive into some code! Here’s a simple JavaScript example that displays a message in the browser’s console:
1console.log("Hello, JavaScript!");
To run this code, open your web browser’s developer tools (usually by pressing F12 or right-clicking on a webpage and selecting “Inspect”), navigate to the “Console” tab, and paste the code snippet. Press Enter, and you should see the message “Hello, JavaScript!” displayed in the console.
To start learning and working with JavaScript, you’ll need to set up a development environment. Here’s a step-by-step guide on how to do this:
Install a Code Editor: First, you’ll need a code editor to write and edit your JavaScript code. Some popular code editors include Visual Studio Code, Sublime Text, and Atom. Download and install your preferred code editor.
Create a New JavaScript File: Create a new file with a “.js” extension in your code editor. This will be your main JavaScript file where you’ll write your code.
Write Your JavaScript Code: Start writing your JavaScript code in the “.js” file you created. Here’s a simple example to get you started:
javascript1console.log("Hello, JavaScript!");
Run Your JavaScript Code: To run your JavaScript code, you have a few options:
Browser Console: You can open your web browser’s developer tools (usually by pressing F12 or right-clicking on a webpage and selecting “Inspect”), navigate to the “Console” tab, and paste your JavaScript code directly into the console. Press Enter, and your code should run and display the output in the console.
HTML File: Alternatively, you can create an HTML file, include your JavaScript code within a
<script>
tag, and open the HTML file in your web browser. Here’s an example:html1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <meta name="viewport" content="width=device-width, initial-scale=1.0">
6 <title>JavaScript Example</title>
7</head>
8<body>
9 <script>
10 console.log("Hello, JavaScript!");
11 </script>
12</body>
13</html>Node.js: If you’re planning to use JavaScript for server-side programming, you can install Node.js, which includes a JavaScript runtime built on Chrome’s V8 JavaScript engine. After installing Node.js, you can run your JavaScript code using the
node
command in your terminal or command prompt.
Learn JavaScript: To learn more about JavaScript, you can refer to various online resources, such as Mozilla Developer Network (MDN) Web Docs, W3Schools, and freeCodeCamp. Additionally, you can practice your JavaScript skills by solving coding challenges on platforms like LeetCode, HackerRank, and Codewars.
That’s it! You’re now set up to start learning and working with JavaScript. Happy coding!
Variables, data types, and operators
In JavaScript, you’ll often work with variables, data types, and operators. Let’s explore each of these concepts in detail.
Variables: Variables are used to store data and values in JavaScript. You can declare variables using the let
, const
, or var
keywords. Here’s an example:
1let message = "Hello, JavaScript!";
2console.log(message); // Output: Hello, JavaScript!
Data Types: JavaScript has several data types, including:
String: A sequence of characters, enclosed in single or double quotes.
javascript1let message = "Hello, JavaScript!";
2console.log(typeof message); // Output: stringNumber: A numeric value, which can be an integer or a floating-point number.
javascript1let age = 25;
2console.log(typeof age); // Output: numberBoolean: A logical value that can be either
true
orfalse
.javascript1let isStudent = true;
2console.log(typeof isStudent); // Output: booleanNull: Represents the intentional absence of any object value.
javascript1let empty = null;
2console.log(typeof empty); // Output: object (this is a historical bug in JavaScript)Undefined: Represents the value of a variable that has not been initialized.
javascript1let name;
2console.log(typeof name); // Output: undefinedObject: A collection of properties, where each property is an association between a key and a value.
javascript1let person = {
2 name: "John Doe",
3 age: 25
4};
5console.log(typeof person); // Output: objectSymbol: A unique and immutable primitive value used for creating unique property keys.
javascript1let id = Symbol("unique-id");
2console.log(typeof id); // Output: symbol
Operators: JavaScript supports various operators, including:
Arithmetic Operators: Perform arithmetic calculations.
javascript1let a = 10;
2let b = 5;
3console.log(a + b); // Output: 15
4console.log(a - b); // Output: 5
5console.log(a * b); // Output: 50
6console.log(a / b); // Output: 2
7console.log(a % b); // Output: 0
8console.log(a ** b); // Output: 100000 (a to the power of b)
9console.log(++a); // Output: 11 (pre-increment)
10console.log(a--); // Output: 10 (post-decrement)Comparison Operators: Compare values and return a boolean value.
javascript1console.log(a == b); // Output: false
2console.log(a != b); // Output: true
3console.log(a > b); // Output: true
4console.log(a < b); // Output: false
5console.log(a >= b); // Output: true
6console.log(a <= b); // Output: false
7console.log(a === b); // Output: false (strict equality)
8console.log(a !== b); // Output: true (strict inequality)Logical Operators: Combine boolean expressions and return a boolean value.
javascript1console.log(true && false); // Output: false
2console.log(true || false); // Output: true
3console.log(!true); // Output: falseAssignment Operators: Assign values to variables.
javascript1let x = 5;
2x += 3; // x = x + 3;
3console.log(x); // Output: 8
4x -= 2; // x = x - 2;
5console.log(x); // Output: 6
6x *= 2; // x = x * 2;
7console.log(x); // Output: 12Control structures: if/else, switch, loops
Control structures are essential in JavaScript for making decisions and controlling the flow of code execution. Here are some common control structures in JavaScript:
If/Else Statements: The
if
statement tests a condition and executes a block of code if the condition is true. Theelse
statement provides an alternative block of code to execute if the condition is false.javascript1let age = 18;
2
3if (age >= 18) {
4 console.log("You are an adult.");
5} else {
6 console.log("You are not an adult yet.");
7}Switch Statements: The
switch
statement tests a value against multiple cases and executes a block of code if a match is found.javascript1let day = "Monday";
2
3switch (day) {
4 case "Monday":
5 console.log("Today is Monday.");
6 break;
7 case "Tuesday":
8 console.log("Today is Tuesday.");
9 break;
10 default:
11 console.log("I don't know what day it is.");
12}Loops: Loops are used to repeat a block of code multiple times. JavaScript supports several types of loops, including:
For Loop: The
for
loop is used to iterate over a sequence of values, typically an array or a range of numbers.javascript1for (let i = 0; i < 5; i++) {
2 console.log(i);
3}While Loop: The
while
loop continues to execute a block of code as long as a condition is true.javascript1let i = 0;
2while (i < 5) {
3 console.log(i);
4 i++;
5}Do-While Loop: The
do-while
loop is similar to thewhile
loop, but the block of code is executed at least once before the condition is tested.javascript1let i = 0;
2do {
3 console.log(i);
4 i++;
5} while (i < 5);For-In Loop: The
for-in
loop is used to iterate over the properties of an object.javascript1const person = {
2 name: "John Doe",
3 age: 25
4};
5
6for (const key in person) {
7 console.log(`${key}: ${person[key]}`);
8}For-Of Loop: The
for-of
loop is used to iterate over the values of an iterable object, such as an array or a string.javascript1const numbers = [1, 2, 3, 4, 5];
2
3for (const number of numbers) {
4 console.log(number);
5}
These are the basic control structures in JavaScript. Mastering these concepts will help you write more complex and dynamic code.
Functions and Arrays
Functions are reusable blocks of code that perform a specific task in JavaScript. Here’s how to define and call functions:
Function Declaration: Function declarations are defined using the function
keyword, followed by the function name, parentheses ()
, and curly braces {}
.
1function greet() {
2 console.log("Hello, world!");
3}
To call a function, simply use its name followed by parentheses ()
.
1greet(); // Output: Hello, world!
Function Expression: Functions can also be defined as expressions, assigned to a variable.
1const greet = function() {
2 console.log("Hello, world!");
3};
To call a function expression, simply use its variable name followed by parentheses ()
.
1greet(); // Output: Hello, world!
Function Parameters: Functions can accept parameters, which are values passed to the function when it is called.
1function greet(name) {
2 console.log(`Hello, ${name}!`);
3}
4
5greet("John Doe"); // Output: Hello, John Doe!
Function Return Values: Functions can return a value using the return
keyword.
1function square(number) {
2 return number * number;
3}
4
5const result = square(5);
6console.log(result); // Output: 25
Arrow Functions: Arrow functions are a concise syntax for defining functions.
1const greet = (name) => {
2 console.log(`Hello, ${name}!`);
3};
4
5greet("John Doe"); // Output: Hello, John Doe!
6
7const square = (number) => number * number;
8const result = square(5);
9console.log(result); // Output: 25
These are the basics of defining and calling functions in JavaScript. Understanding these concepts will help you write reusable and modular code.
Function arguments and return values
In JavaScript, functions can accept arguments and return values. Let’s explore these concepts in more detail:
Function Arguments: Arguments are values passed to a function when it is called. functions can accept any number of arguments, and they can be of any data type. However, if a function is defined with parameters, it will expect a corresponding number of arguments when it is called.
Here’s an example of a function that accepts two arguments:
1function add(a, b) {
2 return a + b;
3}
4
5const result = add(2, 3);
6console.log(result); // Output: 5
If you call the add
function with only one argument, JavaScript will not throw an error. Instead, the second argument will be undefined
.
1const result = add(2); // Output: NaN (Not a Number)
To handle a variable number of arguments, you can use the arguments
object, which is an array-like object that contains all the arguments passed to the function.
1function sum() {
2 let total = 0;
3 for (let i = 0; i < arguments.length; i++) {
4 total += arguments[i];
5 }
6 return total;
7}
8
9const result = sum(1, 2, 3, 4, 5);
10console.log(result); // Output: 15
Function Return Values: Functions can return a value using the return
keyword. If a function does not explicitly return a value, it will return undefined
.
Here’s an example of a function that returns a value:
1function square(number) {
2 return number * number;
3}
4
5const result = square(5);
6console.log(result); // Output: 25
A function can return any data type, including objects, arrays, and other functions.
1function createPerson(name, age) {
2 return {
3 name,
4 age
5 };
6}
7
8const person = createPerson("John Doe", 25);
9console.log(person); // Output: { name: 'John Doe', age: 25 }
Functions can also return multiple values using an array or an object.
1function getUserData() {
2 return {
3 name: "John Doe",
4 age: 25,
5 email: "[email protected]"
6 };
7}
8
9const { name, age, email } = getUserData();
10console.log(name, age, email); // Output: John Doe 25 [email protected]
These are the basics of function arguments and return values in JavaScript. Understanding these concepts will help you write more flexible and reusable code.
Arrays and array methods
In JavaScript, arrays are used to store and manipulate collections of values. Here’s how to define and manipulate arrays in JavaScript:
Array Declaration: Arrays are defined using square brackets []
and comma-separated values.
1const numbers = [1, 2, 3, 4, 5];
2const fruits = ["apple", "banana", "orange"];
3const mixed = [1, "apple", true, { name: "John Doe" }];
Array Length: The length
property of an array returns the number of elements in the array.
1const numbers = [1, 2, 3, 4, 5];
2console.log(numbers.length); // Output: 5
Array Methods: JavaScript arrays have several built-in methods that can be used to manipulate and iterate over the elements of an array. Here are some common array methods:
push: Adds one or more elements to the end of an array and returns the new length of the array.
javascript1const numbers = [1, 2, 3];
2numbers.push(4, 5);
3console.log(numbers); // Output: [1, 2, 3, 4, 5]pop: Removes the last element from an array and returns the removed element.
javascript1const numbers = [1, 2, 3];
2const lastNumber = numbers.pop();
3console.log(numbers); // Output: [1, 2]
4console.log(lastNumber); // Output: 3shift: Removes the first element from an array and returns the removed element.
javascript1const numbers = [1, 2, 3];
2const firstNumber = numbers.shift();
3console.log(numbers); // Output: [2, 3]
4console.log(firstNumber); // Output: 1unshift: Adds one or more elements to the beginning of an array and returns the new length of the array.
javascript1const numbers = [2, 3];
2numbers.unshift(1);
3console.log(numbers); // Output: [1, 2, 3]slice: Returns a shallow copy of a portion of an array into a new array object.
javascript1const numbers = [1, 2, 3, 4, 5];
2const slice = numbers.slice(1, 4);
3console.log(slice); // Output: [2, 3, 4]splice: Changes the content of an array by removing, replacing, or adding elements.
javascript1const numbers = [1, 2, 3, 4, 5];
2numbers.splice(1, 2, 6, 7);
3console.log(numbers); // Output: [1, 6, 7, 4, 5]forEach: Executes a provided function once for each element in the array.
javascript1const numbers = [1, 2, 3, 4, 5];
2numbers.forEach((number) => console.log(number));map: Creates a new array with the results of calling a provided function on every element in the array.
javascript1const numbers = [1, 2, 3, 4, 5];
2const squares = numbers.map((number) => number * number);
3console.log(squares); // Output: [1, 4, 9, 16, 25]filter: Creates a new array with all elements that pass the test implemented by the provided function.
javascript1const numbers = [1, 2, 3, 4, 5];
2const evenNumbers = numbers.filter((number) => number % 2 === 0);
3console.log(evenNumbers); // Output: [2, 4]reduce: Applies a function against an accumulator and each element in the array (from left to right) to reduce it to a
single output value.
javascript1const numbers = [1, 2, 3, 4, 5];
2const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
3console.log(sum); // Output: 15These are some of the most commonly used array methods in JavaScript. Understanding these methods will help you manipulate and iterate over arrays more efficiently.
Additionally, you can use the spread operator
...
to copy or merge arrays, and the destructuring assignment syntax to extract values from arrays or objects.javascript1const numbers1 = [1, 2, 3];
2const numbers2 = [4, 5, 6];
3const mergedNumbers = [...numbers1, ...numbers2];
4console.log(mergedNumbers); // Output: [1, 2, 3, 4, 5, 6]
5
6const [first, ...rest] = numbers1;
7console.log(first, rest); // Output: 1 [2, 3]These are the basics of arrays and array methods in JavaScript. Understanding these concepts will help you write more efficient and expressive code.
Objects and object literals
In JavaScript, objects are used to store and manipulate collections of key-value pairs. Here’s how to define and manipulate objects in JavaScript:
Object Declaration: Objects are defined using curly braces
{}
and key-value pairs, where keys are strings and values can be of any data type.javascript1const person = {
2 name: "John Doe",
3 age: 25,
4 isStudent: true,
5 hobbies: ["reading", "writing"],
6 address: {
7 street: "123 Main St",
8 city: "Anytown",
9 state: "CA",
10 zip: "12345"
11 }
12};Object Properties: You can access an object’s properties using dot notation or bracket notation.
javascript1const person = {
2 name: "John Doe",
3 age: 25
4};
5
6console.log(person.name); // Output: John Doe
7console.log(person["age"]); // Output: 25Object Methods: Objects can have methods, which are functions defined as properties of the object.
javascript1const person = {
2 name: "John Doe",
3 age: 25,
4 introduce: function() {
5 console.log(`Hi, I'm ${this.name} and I'm ${this.age} years old.`);
6 }
7};
8
9person.introduce(); // Output: Hi, I'm John Doe and I'm 25 years old.Computed Property Names: You can use computed property names to define object properties with dynamic keys.
javascript1const key = "name";
2const person = {
3 [key]: "John Doe"
4};
5
6console.log(person.name); // Output: John DoeObject Methods Shorthand: You can use object method shorthand to define methods in object literals.
javascript1const person = {
2 name: "John Doe",
3 age: 25,
4 introduce() {
5 console.log(`Hi, I'm ${this.name} and I'm ${this.age} years old.`);
6 }
7};
8
9person.introduce(); // Output: Hi, I'm John Doe and I'm 25 years old.Object Spread Operator: You can use the object spread operator
...
to copy or merge objects.javascript1const person1 = {
2 name: "John Doe",
3 age: 25
4};
5
6const person2 = {
7 ...person1,
8 isStudent: true
9};
10
11console.log(person2); // Output: { name: 'John Doe', age: 25, isStudent: true }These are the basics of objects and object literals in JavaScript. Understanding these concepts will help you write more efficient and expressive code.
Additionally, you can use the
Object.keys()
,Object.values()
, andObject.entries()
methods to get arrays of keys, values, or key-value pairs from an object.javascript1const person = {
2 name: "John Doe",
3 age: 25
4};
5
6console.log(Object.keys(person)); // Output: [ 'name', 'age' ]
7console.log(Object.values(person)); // Output: [ 'John Doe', 25 ]
8console.log(Object.entries(person)); // Output: [ [ 'name', 'John Doe' ], [ 'age', 25 ] ]These methods can be useful for iterating over objects or converting objects to arrays.
DOM Manipulation
The Document Object Model (DOM) is a programming interface for HTML and XML documents. It represents the structure of a document as a tree of nodes, where each node represents an element, attribute, or text content in the document. The DOM allows JavaScript to interact with the content and structure of a web page, enabling dynamic content and user interactivity.
In JavaScript, you can manipulate the DOM using the built-in Document
object, which represents the entire HTML document. Here are some common DOM manipulation techniques:
Selecting Elements: You can select elements in the DOM using various methods, such as document.getElementById()
, document.getElementsByClassName()
, document.getElementsByTagName()
, and document.querySelector()
.
1// Select an element by ID
2const header = document.getElementById("header");
3
4// Select elements by class name
5const paragraphs = document.getElementsByClassName("paragraph");
6
7// Select elements by tag name
8const links = document.getElementsByTagName("a");
9
10// Select the first <p> element with class "intro"
11const intro = document.querySelector(".intro");
12
13// Select all <li> elements with class "active"
14const activeItems = document.querySelectorAll("li.active");
Creating Elements: You can create new elements using the document.createElement()
method.
1const newParagraph = document.createElement("p");
2newParagraph.textContent = "This is a new paragraph.";
3
4// Append the new paragraph to the document
5document.body.appendChild(newParagraph);
Modifying Elements: You can modify existing elements using various properties, such as textContent
, innerHTML
, style
, and setAttribute()
.
1// Set the text content of a <p> element
2const paragraph = document.getElementById("paragraph");
3paragraph.textContent = "This is a modified paragraph.";
4
5// Set the HTML content of a <div> element
6const div = document.getElementById("div");
7div.innerHTML = "<p>This is a new paragraph.</p>";
8
9// Set the style of a <h1> element
10const heading = document.getElementById("heading");
11heading.style.color = "red";
12heading.style.fontSize = "24px";
13
14// Set an attribute of a <img> element
15const image = document.getElementById("image");
16image.setAttribute("src", "new-image.jpg");
17image.setAttribute("alt", "New Image");
Removing Elements: You can remove elements using the removeChild()
method.
1// Remove a <p> element
2const paragraph = document.getElementById("paragraph");
3document.body.removeChild(paragraph);
These are the basics of the Document Object Model (DOM) in JavaScript. Understanding these concepts will help you create dynamic and interactive web pages.
Additionally, you can use libraries and frameworks like jQuery, React, and Angular to simplify DOM manipulation and make it more efficient. These libraries and frameworks provide higher-level abstractions and optimized implementations for common DOM manipulation tasks.
Selecting and manipulating DOM elements
In JavaScript, you can select and manipulate DOM elements using various methods. Here are some common DOM manipulation techniques:
Selecting Elements: You can select elements in the DOM using various methods, such as document.getElementById()
, document.getElementsByClassName()
, document.getElementsByTagName()
, and document.querySelector()
.
1// Select an element by ID
2const header = document.getElementById("header");
3
4// Select elements by class name
5const paragraphs = document.getElementsByClassName("paragraph");
6
7// Select elements by tag name
8const links = document.getElementsByTagName("a");
9
10// Select the first <p> element with class "intro"
11const intro = document.querySelector(".intro");
12
13// Select all <li> elements with class "active"
14const activeItems = document.querySelectorAll("li.active");
Creating Elements: You can create new elements using the document.createElement()
method.
1const newParagraph = document.createElement("p");
2newParagraph.textContent = "This is a new paragraph.";
3
4// Append the new paragraph to the document
5document.body.appendChild(newParagraph);
Modifying Elements: You can modify existing elements using various properties, such as textContent
, innerHTML
, style
, and setAttribute()
.
1// Set the text content of a <p> element
2const paragraph = document.getElementById("paragraph");
3paragraph.textContent = "This is a modified paragraph.";
4
5// Set the HTML content of a <div> element
6const div = document.getElementById("div");
7div.innerHTML = "<p>This is a new paragraph.</p>";
8
9// Set the style of a <h1> element
10const heading = document.getElementById("heading");
11heading.style.color = "red";
12heading.style.fontSize = "24px";
13
14// Set an attribute of a <img> element
15const image = document.getElementById("image");
16image.setAttribute("src", "new-image.jpg");
17image.setAttribute("alt", "New Image");
Removing Elements: You can remove elements using the removeChild()
method.
1// Remove a <p> element
2const paragraph = document.getElementById("paragraph");
3document.body.removeChild(paragraph);
These are the basics of selecting and manipulating DOM elements in JavaScript. Understanding these concepts will help you create dynamic and interactive web pages.
Additionally, you can use libraries and frameworks like jQuery, React, and Angular to simplify DOM manipulation and make it more efficient. These libraries and frameworks provide higher-level abstractions and optimized implementations for common DOM manipulation tasks.
For example, in jQuery, you can select elements using the $()
function and manipulate them using various methods.
1// Select an element by ID
2const header = $("#header");
3
4// Set the text content of a <p> element
5const paragraph = $(".paragraph");
6paragraph.text("This is a modified paragraph.");
7
8// Set the HTML content of a <div> element
9const div = $("div");
10div.html("<p>This is a new paragraph.</p>");
11
12// Set the style of a <h1> element
13const heading = $("h1");
14heading.css("color", "red");
15heading.css("font-size", "24px");
16
17// Set an attribute of a <img> element
18const image = $("img");
19image.attr("src", "new-image.jpg");
20image.attr("alt", "New Image");
21
22// Remove a <p> element
23const paragraph = $("p");
24paragraph.remove();
These are just a few examples of how you can select and manipulate DOM elements in JavaScript and jQuery. There are many more methods and techniques available for more complex use cases.
Handling events
In JavaScript, you can handle events by attaching event listeners to DOM elements. An event listener is a function that is called when a specific event occurs, such as a user clicking a button or loading a web page. Here are some common event handling techniques:
Event Listeners: You can attach event listeners to DOM elements using the addEventListener()
method.
1// Attach a click event listener to a button
2const button = document.getElementById("button");
3button.addEventListener("click", function() {
4 console.log("Button was clicked.");
5});
6
7// Attach a load event listener to the window object
8window.addEventListener("load", function() {
9 console.log("Window was loaded.");
10});
Event Object: When an event occurs, an event object is passed to the event listener function. The event object contains information about the event, such as the type of event, the target element, and any data associated with the event.
1// Attach a click event listener to a button
2const button = document.getElementById("button");
3button.addEventListener("click", function(event) {
4 console.log("Button was clicked:", event.type, event.target);
5});
Event Bubbling: Events in the DOM bubble up the DOM tree, meaning that an event triggered on a child element will also trigger the same event on its parent elements. You can prevent event bubbling using the event.stopPropagation()
method.
1// Attach a click event listener to a parent element
2const parent = document.getElementById("parent");
3parent.addEventListener("click", function() {
4 console.log("Parent was clicked.");
5});
6
7// Attach a click event listener to a child element
8const child = document.getElementById("child");
9child.addEventListener("click", function(event) {
10 console.log("Child was clicked.");
11 event.stopPropagation();
12});
Event Delegation: Event delegation is a technique where you attach a single event listener to a parent element and handle events triggered by its child elements. This can improve performance and simplify event handling.
1// Attach a click event listener to a parent element
2const parent = document.getElementById("parent");
3parent.addEventListener("click", function(event) {
4 if (event.target.tagName === "BUTTON") {
5 console.log("Button was clicked.");
6 }
7});
These are the basics of handling events in JavaScript. Understanding these concepts will help you create dynamic and interactive web pages.
Additionally, you can use libraries and frameworks like jQuery, React, and Angular to simplify event handling and make it more efficient. These libraries and frameworks provide higher-level abstractions and optimized implementations for common event handling tasks.
For example, in jQuery, you can attach event listeners using the on()
method.
1// Attach a click event listener to a button
2const button = $("#button");
3button.on("click", function() {
4 console.log("Button was clicked.");
5});
6
7// Attach a load event listener to the window object
8$(window).on("load", function() {
9 console.log("Window was loaded.");
10});
These are just a few examples of how you can handle events in JavaScript and jQuery. There are many more methods and techniques available for more complex use cases.
In addition to event listeners, you can also use event attributes in HTML to handle events directly in the markup. However, this approach is generally discouraged in modern web development due to its limitations and the benefits of separating concerns between markup and script.
1<button onclick="console.log('Button was clicked.')">Click me</button>
This approach can be useful for simple use cases, but for more complex scenarios, it is recommended to use JavaScript event listeners.
Creating and modifying HTML elements
1In JavaScript, you can create and modify HTML elements using methods. Here are some common:
Creating Elements: You can create new elements using the .createElement()
.
1const newParagraph = document.("p");
2newParagraph.text = "This is a new paragraph.";
3
4// Append the new paragraph to document
5document..appendChild(newParagraph);
Modifying Elements: You can modify existing elements using various properties, such as textContent
, innerHTML
, style
, and setAttribute()
.
1// Set the text content of a <p> element
2const paragraph = document.getElementById("paragraph");
3paragraph.textContent = "This is a modified paragraph.";
4
5// Set the HTML content of a <div> element
6const div = document.getElementById("div");
7div.innerHTML = "<p>This is a new paragraph.</p>";
8
9// Set the style of a <h1> element
10const heading = document.getElementById("heading");
11heading.style.color = "red";
12heading.style.fontSize = "24px";
13
14// Set an attribute of a <img> element
15const image = document.getElementById("image");
16image.setAttribute("src", "new-image.jpg");
17image.setAttribute("alt", "New Image");
Creating and Modifying Elements Using Templates: You can use template literals and the insertAdjacentHTML()
method to create and modify HTML elements more efficiently.
1// Create a new <div> element with a <p> element inside
2const template = `
3 <div>
4 <p>This is a new paragraph.</p>
5 </div>
6`;
7
8// Insert the new <div> element after the existing <div> element
9const div = document.getElementById("div");
10div.insertAdjacentHTML("afterend", template);
Creating and Modifying Elements Using InnerHTML: You can use the innerHTML
property to create and modify HTML elements more efficiently.
1// Create a new <div> element with a <p> element inside
2const template = `
3 <div>
4 <p>This is a new paragraph.</p>
5 </div>
6`;
7
8// Set the innerHTML of a <div> element
9const div = document.getElementById("div");
10div.innerHTML = template;
Creating and Modifying Elements Using jQuery: You can use jQuery to create and modify HTML elements more efficiently.
1// Create a new <p> element
2const newParagraph = $("<p>").text("This is a new paragraph.");
3
4// Append the new paragraph to the document
5$("body").append(newParagraph);
6
7// Set the text content of a <p> element
8const paragraph = $("#paragraph");
9paragraph.text("This is a modified paragraph.");
10
11// Set the HTML content of a <div> element
12const div = $("#div");
13div.html("<p>This is a new paragraph.</p>");
14
15// Set the style of a <h1> element
16const heading = $("#heading");
17heading.css("color", "red");
18heading.css("font-size", "24px");
19
20// Set an attribute of a <img> element
21const image = $("#image");
22image.attr("src", "new-image.jpg");
23image.attr("alt", "New Image");
24
25// Create a new <div> element with a <p> element inside
26const template = `
27 <div>
28 <p>This is a new paragraph.</p>
29 </div>
30`;
31
32// Insert the new <div> element after the existing <div> element
33const div = $("#div");
34div.after(template);
These are the basics of creating and modifying HTML elements in JavaScript. Understanding these concepts will help you create dynamic and interactive web pages.
Additionally, you can use libraries and frameworks like React, Angular, and Vue.js to simplify HTML element creation and make it more efficient. These libraries and frameworks provide higher-level abstractions and optimized implementations for common HTML element creation and modification tasks.
For example, in React, you can create and modify HTML elements using JSX, a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files.
a new <p> element in JSX const newParagraph = <p>This is a new paragraph.</p>;
// Render the new paragraph to the documentReactDOM.render(newParagraph, document.getElementById(“”));
// Set the text content of a <p> element using a React component class Paragraph extends React.Component { render() { return <p>This is a modified paragraph.</p>; } }
// Render the Paragraph component to the document ReactDOM.render(<Paragraph />, document.getElementById(“paragraph”));
// Set the HTML content of a <div> element using a React component class Div extends React.Component { render() { return ( <div> <p>This is a new paragraph.</p> </div> ); } }
// Render the Div component to the document ReactDOM.render(<Div />, document.getElementById(“div”));
// Set the style of a <h1> element using a React component class Heading extends React.Component { render() { return <h1 style={{ color: “red”, fontSize: “24px” }}>Heading</h1>; } }
// Render the Heading component to the document ReactDOM.render(<Heading />, document.getElementById(“heading”));
// Set an attribute of a <img> element using a React component class Image extends React.Component { render() { return <img src=”new-image.jpg” alt=”New Image” />; } }
// Render the Image component to the document ReactDOM.render(<Image />, document.getElementById(“image”));
// Create a new <div> element with a <p> element inside using a React component const template = (
<div> <p>This is a new paragraph.</p> </div> );
// Render the template to the document ReactDOM.render(template, document.getElementById(“div”));
1
2These are just a few examples of how you can create and modify HTML elements in JavaScript and React. There are many more methods and techniques available for more complex use cases.
3
4In addition to the methods and techniques mentioned above, you can also use other libraries and frameworks like Angular and Vue.js to create and modify HTML elements more efficiently. These libraries and frameworks provide higher-level abstractions and optimized implementations for common HTML element creation and modification tasks.
5
6For example, in Angular, you can create and modify HTML elements using Angular templates and components.
7
8```html
9<!-- Create a new <p> element in an Angular template -->
10<p>This is a new paragraph.</p>
11
12<!-- Set the text content of a <p> element using an Angular component -->
13<p>{{ modifiedText }}</p>
14
15<!-- Set the HTML content of a <div> element using an Angular component -->
16<div [innerHtml]="newHtml"></div>
17
18<!-- Set the style of a <h1> element using an Angular component -->
19<h1 [style.color]="'red'" [style.fontSize.px]="24"></h1>
20
21<!-- Set an attribute of a <img> element using an Angular component -->
22<img [src]="newSrc" [alt]="newAlt">
23
24<!-- Create a new <div> element with a <p> element inside using an Angular component -->
25<div [innerHtml]="newDiv"></div>
These are just a few examples of how you can create and modify HTML elements in JavaScript and Angular. There are many more methods and techniques available for more complex use cases.
In Vue.js, you can create and modify HTML elements using Vue templates and components.
1<!-- Create a new <p> element in a Vue template -->
2<p>This is a new paragraph.</p>
3
4<!-- Set the text content of a <p> element using a Vue component -->
5<p>{{ modifiedText }}</p>
6
7<!-- Set the HTML content of a <div> element using a Vue component -->
8<div v-html="newHtml"></div>
9
10<!-- Set the style of a <h1> element using a Vue component -->
11<h1 :style="{ color: 'red', fontSize: '24px' }"></h1>
12
13<!-- Set an attribute of a <img> element using a Vue component
Asynchronous JavaScript
Introduction to asynchronous programming
Asynchronous programming is a programming paradigm that allows a program to perform multiple tasks concurrently, rather than sequentially. In JavaScript, asynchronous programming is commonly used to handle tasks that may take a long time to complete, such as fetching data from a server or waiting for user input.
Here are some common techniques for asynchronous programming in JavaScript:
Callbacks: A callback is a function that is passed as an argument to another function and is called when the task is complete.
1function fetchData(callback) {
2 // Simulate a long-running task
3 setTimeout(() => {
4 callback("Data fetched.");
5 }, 2000);
6}
7
8fetchData((data) => {
9 console.log(data); // Output: Data fetched.
10});
Promises: A Promise is an object that represents the eventual completion or failure of an asynchronous operation. A Promise can be in one of three states: pending, fulfilled, or rejected.
1function fetchData() {
2 return new Promise((resolve, reject) => {
3 // Simulate a long-running task
4 setTimeout(() => {
5 resolve("Data fetched.");
6 }, 2000);
7 });
8}
9
10fetchData().then((data) => {
11 console.log(data); // Output: Data fetched.
12});
Async/Await: Async/Await is a syntax sugar for Promises that allows you to write asynchronous code that looks like synchronous code.
1async function fetchData() {
2 // Simulate a long-running task
3 await new Promise((resolve) => setTimeout(() => resolve("Data fetched."), 2000));
4}
5
6fetchData().then((data) => {
7 console.log(data); // Output: Data fetched.
8});
These are the basics of asynchronous programming in JavaScript. Understanding these concepts will help you write efficient and performant code for handling long-running tasks.
Additionally, you can use libraries and frameworks like Axios, Fetch API, and Bluebird to simplify asynchronous programming and make it more efficient. These libraries and frameworks provide higher-level abstractions and optimized implementations for common asynchronous programming tasks.
For example, in Axios, you can use the axios
function to fetch data from a server asynchronously.
1axios.get("https://jsonplaceholder.typicode.com/posts")
2 .then((response) => {
3 console.log(response.data);
4 })
5 .catch((error) => {
6 console.log(error);
7 });
These are just a few examples of how you can use asynchronous programming in JavaScript. There are many more methods and techniques available for more complex use cases.
Promises and async/await
Promises and async/await are two common techniques for asynchronous programming in JavaScript. Here’s a more detailed explanation of both:
Promises: A Promise is an object that represents the eventual completion or failure of an asynchronous operation. A Promise can be in one of three states: pending, fulfilled, or rejected. A Promise is created using the Promise
constructor, which takes a single argument, a function called the executor. The executor function takes two arguments, resolve
and reject
, which are both functions. The resolve
function is called when the asynchronous operation is successful, and the reject
function is called when the asynchronous operation fails.
Here’s an example of using Promises to fetch data from a server:
1const fetchData = () => {
2 return new Promise((resolve, reject) => {
3 // Simulate a long-running task
4 setTimeout(() => {
5 resolve("Data fetched.");
6 }, 2000);
7 });
8};
9
10fetchData().then((data) => {
11 console.log(data); // Output: Data fetched.
12});
In this example, the fetchData
function returns a new Promise that simulates a long-running task using the setTimeout
function. When the timeout is complete, the Promise is fulfilled with the value “Data fetched.”. The then
method is called when the Promise is fulfilled, and the callback function is passed the fulfilled value as an argument.
Async/Await: Async/Await is a syntax sugar for Promises that allows you to write asynchronous code that looks like synchronous code. An async function is a function declared with the async
keyword, and it always returns a Promise. The await
keyword is used to wait for a Promise to be fulfilled or rejected before continuing execution.
Here’s an example of using async/await to fetch data from a server:
1const fetch = async () => {
2 // Simulate a long-running task
3 new Promise((resolve => setTimeout(() => resolve("Data fetched."), 2000));
4 return "Data fetched.";
5};
6
7fetchData().then((data) => {
8 console.log(data); // Output: Data fetched.
9});
In this example, the fetchData
function declared with the async
keyword, making it async function. The await
keyword is used to wait for the Promise returned by the new Promise
constructor to be fulfilled or rejected before continuing execution. When the Promise is fulfilled, the fetchData
function returns the fulfilled value “Data fetched.”. The then
method is called when the Promise returned by the fetchData
function is fulfilled, and the callback function is passed the fulfilled value as an argument.
Async/await makes asynchronous code look more like synchronous code, making it easier to read and understand. However, it’s important to note that async/await is just a syntax sugar for Promises and relies on Promises under the hood.
Here’s an example of using async/await with the fetch
API to fetch data from a server:
1const fetchData = async () => {
2 const response = await fetch("https://jsonplaceholder.typicode.com/posts");
3 const data = await response.json();
4 return data;
5};
6
7fetchData().then((data) => {
8 console.log(data);
9}).catch((error) => {
10 console.log(error);
11});
In this example, the fetchData
function uses the fetch
API to fetch data from a server. The await
keyword is used to wait for the Promise returned by the fetch
function to be fulfilled or rejected before continuing execution. When the Promise is fulfilled, the response
variable is assigned the fulfilled value, which is a Response object. The json
method is called on the Response object to extract the JSON data, and the await
keyword is used again to wait for the Promise returned by the json
method to be fulfilled or rejected before continuing execution. When the Promise is fulfilled, the data
variable is assigned the fulfilled value, which is the JSON data. The fetchData
function returns the fulfilled value data
. The then
method is called when the Promise returned by the fetchData
function is fulfilled, and the callback function is passed the fulfilled value as an argument. The catch
method is called when the Promise is rejected, and the error is logged to the console.
These are just a few examples of how you can use Promises and async/await in JavaScript. There are many more methods and techniques available for more complex use cases. It’s important to note that Promises and async/await are just two of many techniques for asynchronous programming in JavaScript, and the choice of which technique to use depends on the specific use case and personal preference.
Fetch API for making HTTP requests
The Fetch API is a built-in JavaScript interface for making HTTP requests. It’s a modern alternative to the older XMLHttpRequest interface and provides a simpler and more powerful way to make HTTP requests in JavaScript.
Here’s an example of using the Fetch API to fetch data from a server:
1fetch("https://jsonplaceholder.typicode.com/posts")
2 .then((response) => {
3 return response.json();
4 })
5 .then((data) => {
6 console.log(data);
7 })
8 .catch((error) => {
9 console.log(error);
10 });
In this example, the fetch
function is called with the URL of the server as an argument. The fetch
function returns a Promise that resolves to a Response object. The then
method is called on the Promise, and the callback function is passed the Response object as an argument. The json
method is called on the Response object to extract the JSON data, and the then
method is called again to handle the JSON data. The catch
method is called when the Promise is rejected, and the error is logged to the console.
The Fetch API provides several options for customizing the HTTP request, such as specifying the HTTP method, headers, and body. Here’s an example of using the Fetch API to send a POST request with a JSON payload:
1const data = {
2 title: "New Post",
3 body: "This is a new post.",
4 userId: 1,
5};
6
7fetch("https://jsonplaceholder.typicode.com/posts", {
8 method: "POST",
9 headers: {
10 "Content-Type": "application/json",
11 },
12 body: JSON.stringify(data),
13})
14 .then((response) => {
15 return response.json();
16 })
17 .then((data) => {
18 console.log(data);
19 })
20 .catch((error) => {
21 console.log(error);
22 });
In this example, the fetch
function is called with the URL of the server and an options object as arguments. The options object specifies the HTTP method as “POST”, the Content-Type
header as “application/json”, and the JSON payload as the body. The json
method is called on the Response object to extract the JSON data, and the then
method is called again to handle the JSON data. The catch
method is called when the Promise is rejected, and the error is logged to the console.
These are just a few examples of how you can use the Fetch API in JavaScript. There are many more options and techniques available for more complex use cases. It’s important to note that the Fetch API is just one of many interfaces for making HTTP requests in JavaScript, and the choice of which interface to use depends on the specific use case and personal preference.
Handling errors and timeouts
In JavaScript, errors and timeouts are common issues that can occur when working with asynchronous code. Here are some common techniques for handling errors and timeouts in JavaScript:
Handling Errors: Errors can occur when a Promise is rejected or an exception is thrown in asynchronous code. To handle errors, you can use the catch
method of a Promise or the try-catch
statement.
Here’s an example of using the catch
method to handle errors in Promises:
1fetch("https://jsonplaceholder.typicode.com/posts")
2 .then((response) => {
3 return response.json();
4 })
5 .then((data) => {
6 console.log(data);
7 })
8 .catch((error) => {
9 console.log(error);
10 });
In this example, the catch
method is called on the Promise returned by the fetch
function. The callback function passed to the catch
method is called when the Promise is rejected, and the error is logged to the console.
Here’s an example of using the try-catch
statement to handle errors in asynchronous code:
1async function fetchData() {
2 try {
3 const response = await fetch("https://jsonplaceholder.typicode.com/posts");
4 const data = await response.json();
5 console.log(data);
6 } catch (error) {
7 console.log(error);
8 }
9}
10
11fetchData();
In this example, the try-catch
statement is used to handle errors in the fetchData
function. The try
block contains the asynchronous code that may throw an exception, and the catch
block is called when an exception is thrown. The error is logged to the console.
Handling Timeouts: Timeouts can occur when a task takes too long to complete, causing the program to become unresponsive or frozen. To handle timeouts, you can use the setTimeout
function.
Here’s an example of using the setTimeout
function to handle timeouts in Promises:
1function fetchData() {
2 return new Promise((resolve, reject) => {
3 const timeoutId = setTimeout(() => {
4 reject(new Error("Timeout"));
5 }, 5000);
6
7 fetch("https://jsonplaceholder.typicode.com/posts")
8 .then((response) => {
9 clearTimeout(timeoutId);
10 return response.json();
11 })
12 .then((data) => {
13 resolve(data);
14 })
15 .catch((error) => {
16 reject(error);
17 });
18 });
19}
20
21fetchData().then((data) => {
22 console.log(data);
23}).catch((error) => {
24 console.log(error);
25});
In this example, the fetchData
function returns a Promise that simulates a long-running task using the setTimeout
function. The setTimeout
function is used to set a timeout of 5 seconds, after which the Promise is rejected with a timeout error. The clearTimeout
function is called when the Promise is fulfilled to clear the timeout. The catch
method is called when the Promise is rejected, and the error is logged to the console.
These are just a few examples of how you can handle errors and timeouts in JavaScript. There are many more methods and techniques available for more complex use cases. It’s important to note that handling errors and timeouts is crucial for writing robust and reliable asynchronous code in JavaScript.
JavaScript Libraries and Frameworks
Introduction to popular JavaScript libraries and frameworks
JavaScript has a large and active ecosystem, with many libraries and frameworks available for various use cases. Here are some popular JavaScript libraries and frameworks:
React: React is a JavaScript library for building user interfaces. It was developed by Facebook and is now maintained by Facebook and a community of individual developers and companies. React allows you to build reusable UI components and efficiently update the UI when data changes.
Angular: Angular is a TypeScript-based open-source framework for building web applications. It was developed by Google and is now maintained by Google and a community of individual developers and companies. Angular provides a complete solution for building web applications, including routing, forms, and state management.
Vue.js: Vue.js is a JavaScript framework for building user interfaces. It was developed by an ex-Google engineer and is now maintained by a community of individual developers and companies. Vue.js allows you to build reusable UI components and efficiently update the UI when data changes.
jQuery: jQuery is a fast, small, and feature-rich JavaScript library for manipulating the DOM and handling events. It was developed by John Resig and is now maintained by a community of individual developers and companies. jQuery provides a simple and consistent API for working with the DOM, regardless of the browser.
Axios: Axios is a promise-based HTTP client for the browser and Node.js. It was developed by Matt Zabriskie and is now maintained by a community of individual developers and companies. Axios provides a simple and consistent API for making HTTP requests and handling responses.
Lodash: Lodash is a utility library for working with arrays, numbers, objects, and strings. It was developed by John-David Dalton and is now maintained by a community of individual developers and companies. Lodash provides a collection of reusable functions for working with common data structures and data manipulation tasks.
D3.js: D3.js is a JavaScript library for creating data visualizations. It was developed by Mike Bostock and is now maintained by a community of individual developers and companies. D3.js allows you to create interactive and dynamic visualizations using HTML, SVG, and CSS.
These are just a few examples of popular JavaScript libraries and frameworks. There are many more libraries and frameworks available for various use cases, such as testing, state management, and performance optimization. It’s important to note that the choice of which library or framework to use depends on the specific use case and personal preference.
Using jQuery for DOM manipulation
jQuery is a popular JavaScript library for manipulating the DOM and handling events. It provides a simple and consistent API for working with the DOM, regardless of the browser. Here’s an example of using jQuery to select and manipulate DOM elements:
1<!DOCTYPE html>
2<html>
3 <head>
4 <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
5 <script>
6 $(document).ready(function () {
7 const $paragraph = $("p");
8 $paragraph.text("This is a new paragraph.");
9 });
10 </script>
11 </head>
12 <body>
13 <p>This is an existing paragraph.</p>
14 </body>
15</html>
In this example, the jQuery library is loaded using a script tag. The $(document).ready
function is called when the DOM is fully loaded. The $
function is used to select the <p>
element, and the text
method is used to change the text content of the <p>
element.
Here’s an example of using jQuery to handle events:
1<!DOCTYPE html>
2<html>
3 <head>
4 <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
5 <script>
6 $(document).ready(function () {
7 $("#button").click(function () {
8 $("#paragraph").text("Button was clicked.");
9 });
10 });
11 </script>
12 </head>
13 <body>
14 <button id="button">Click me</button>
15 <p id="paragraph">This is an existing paragraph.</p>
16 </body>
17</html>
In this example, the jQuery library is loaded using a script tag. The $(document).ready
function is called when the DOM is fully loaded. The $
function is used to select the <button>
element, and the click
method is used to attach a click event listener to the <button>
element. When the button is clicked, the text content of the <p>
element is changed to “Button was clicked.”
These are just a few examples of how you can use jQuery for DOM manipulation and event handling in JavaScript. jQuery provides many more methods and techniques for working with the DOM, such as animations, AJAX requests, and form handling. It’s important to note that jQuery is just one of many libraries and frameworks available for DOM manipulation and event handling in JavaScript, and the choice of which library or framework to use depends on the specific use case and personal preference.
Building web applications with React or Angular
Building web applications with React or Angular requires a different approach compared to traditional JavaScript. Here’s a brief overview of building web applications with React and Angular:
React: React is a JavaScript library for building user interfaces. It allows you to build reusable UI components and efficiently update the UI when data changes. Here’s an example of building a simple web application with React:
- Create a new React project using Create React App or another tool.
- Define a new React component using the
React.createClass
orclass
keyword. - Render the component using the
ReactDOM.render
function.
Here’s an example of a simple React component:
1import React from "react";
2import ReactDOM from "react-dom";
3
4class HelloWorld extends React.Component {
5 render() {
6 return <h1>Hello, world!</h1>;
7 }
8}
9
10ReactDOM.render(<HelloWorld />, document.getElementById("root"));
In this example, a new React component called HelloWorld
is defined. The component renders a <h1>
element with the text “Hello, world!”. The ReactDOM.render
function is used to render the HelloWorld
component to the DOM.
Angular: Angular is a TypeScript-based open-source framework for building web applications. It provides a complete solution for building web applications, including routing, forms, and state management. Here’s an example of building a simple web application with Angular:
- Create a new Angular project using the Angular CLI or another tool.
- Define a new Angular component using the
@Component
decorator. - Render the component using the Angular router or the
bootstrapModule
function.
Here’s an example of a simple Angular component:
1import { Component } from "@angular/core";
2
3@Component({
4 selector: "app-hello-world",
5 template: "<h1>Hello, world!</h1>",
6})
7export class HelloWorldComponent {}
In this example, a new Angular component called HelloWorldComponent
is defined. The component renders a <h1>
element with the text “Hello, world!”. The @Component
decorator is used to define the component’s metadata, such as the selector and template.
These are just a few examples of how you can build web applications with React or Angular. Both React and Angular provide many more features and techniques for building complex web applications, such as state management, routing, and form handling. It’s important to note that building web applications with React or Angular requires a different approach compared to traditional JavaScript, and requires a solid understanding of the framework’s concepts and best practices.
Integrating JavaScript with other tools in bioinformatics workflows
Integrating JavaScript with other tools in bioinformatics workflows can be a powerful way to enhance the functionality and usability of these tools. Here are some common ways to integrate JavaScript with other tools in bioinformatics workflows:
Integrating with RESTful APIs: Many bioinformatics tools provide RESTful APIs for interacting with their data and functionality. JavaScript provides several libraries and frameworks for making HTTP requests and handling responses, such as Axios, Fetch API, and jQuery. By integrating with RESTful APIs, you can use JavaScript to retrieve and manipulate data from bioinformatics tools, and display the results in a user-friendly way.
Here’s an example of using Axios to make a GET request to a RESTful API:
1axios.get("https://api.example.com/data")
2 .then((response) => {
3 console.log(response.data);
4 })
5 .catch((error) => {
6 console.log(error);
7 });
In this example, the axios.get
function is used to make a GET request to the RESTful API at “https://api.example.com/data“. The then
method is called when the request is successful, and the response data is logged to the console. The catch
method is called when the request fails, and the error is logged to the console.
Integrating with Command Line Interfaces (CLIs): Many bioinformatics tools provide CLIs for interacting with their data and functionality. JavaScript provides several libraries and frameworks for executing command line commands and handling output, such as Node.js child_process
module and execa
library. By integrating with CLIs, you can use JavaScript to automate and simplify the execution of bioinformatics tools, and display the results in a user-friendly way.
Here’s an example of using the child_process
module to execute a command line command:
1const { exec } = require("child_process");
2
3exec("command-line-tool", (error, stdout, stderr) => {
4 if (error) {
5 console.error(`exec error: ${error}`);
6 return;
7 }
8 console.log(`stdout: ${stdout}`);
9 if (stderr) {
10 console.error(`stderr: ${stderr}`);
11 }
12});
In this example, the exec
function is used to execute the command line tool “command-line-tool”. The callback function is called when the command is executed, and the output is logged to the console.
Integrating with Web Services: Many bioinformatics tools provide web services for interacting with their data and functionality. JavaScript provides several libraries and frameworks for working with web services, such as SoapUI and Postman. By integrating with web services, you can use JavaScript to automate and simplify the interaction with bioinformatics tools, and display the results in a user-friendly way.
Here’s an example of using SoapUI to test a web service:
- Create a new SoapUI project using the SoapUI application.
- Define a new REST or SOAP request using the SoapUI interface.
- Send the request and view the response in the SoapUI interface.
In this example, SoapUI is used to test a web service. The request and response are displayed in the SoapUI interface, and can be used to interact with the web service from JavaScript.
These are just a few examples of how you can integrate JavaScript with other tools in bioinformatics workflows. By integrating with RESTful APIs, CLIs, and web services, you can use JavaScript to enhance the functionality and usability of bioinformatics tools, and provide a user-friendly interface for interacting with these tools. It’s important to note that integrating with other tools requires a solid understanding of the tool’s API and documentation, and may require additional setup and configuration.
Best Practices and Security
Writing clean and maintainable code
Writing clean and maintainable code in JavaScript is important for ensuring the long-term success and sustainability of your projects. Here are some best practices for writing clean and maintainable code in JavaScript:
Use a Consistent Coding Style: A consistent coding style makes your code easier to read and understand. Follow a coding style guide, such as Airbnb’s JavaScript Style Guide or Google’s JavaScript Style Guide, to ensure consistency in your code. Use a linter, such as ESLint or JSLint, to enforce the coding style guide and catch errors and warnings.
Write Small and Focused Functions: Small and focused functions are easier to understand and test. Break down large functions into smaller, more manageable functions that perform a single task. Avoid using global variables and functions, and instead use local variables and functions.
Use Meaningful Names: Meaningful names make your code easier to read and understand. Use descriptive variable and function names that accurately reflect their purpose and behavior. Avoid using abbreviations and acronyms, and instead use full words and phrases.
Comment Your Code: Comments make your code easier to understand and maintain. Use comments to explain the purpose and behavior of your code, and to provide context and background information. Avoid using comments to explain what the code is doing, and instead use comments to explain why the code is doing what it’s doing.
Test Your Code: Testing makes your code more reliable and maintainable. Write unit tests for your code using a testing framework, such as Jest or Mocha, to ensure that it behaves as expected. Test your code in isolation, and avoid testing implementation details.
Use Version Control: Version control makes your code more manageable and maintainable. Use a version control system, such as Git or Mercurial, to track changes to your code and collaborate with others. Use descriptive commit messages to explain the changes you’ve made.
Keep Your Code DRY: Don’t Repeat Yourself (DRY) makes your code more concise and maintainable. Avoid duplicating code, and instead extract common functionality into reusable functions or modules.
These are just a few best practices for writing clean and maintainable code in JavaScript. By following these best practices, you can ensure that your code is easy to read, understand, and maintain, and that it behaves as expected. It’s important to note that writing clean and maintainable code requires a commitment to quality and a disciplined approach to development.
Debugging techniques
Debugging is the process of identifying and fixing bugs in your code. Debugging techniques in JavaScript can help you quickly and efficiently identify and fix bugs in your code. Here are some common debugging techniques in JavaScript:
Use console.log
: console.log
is a simple and effective way to print values and messages to the console. Use console.log
to print the values of variables and expressions, and to trace the flow of your code.
Here’s an example of using console.log
to debug a variable:
1const x = 5;
2console.log(x); // Output: 5
Use Debugging Tools: Debugging tools, such as the Chrome DevTools or Firefox Developer Edition, provide advanced debugging features, such as breakpoints, step-by-step execution, and variable inspection. Use debugging tools to step through your code, inspect variables and expressions, and identify bugs.
Here’s an example of using the Chrome DevTools to set a breakpoint:
- Open the Chrome DevTools using the F12 key or right-clicking on the page and selecting “Inspect”.
- Navigate to the “Sources” tab.
- Select the JavaScript file and line of code where you want to set the breakpoint.
- Click the line number to set the breakpoint.
- Reload the page and interact with the code.
- When the breakpoint is hit, inspect the variables and expressions.
Use Debugging Statements: Debugging statements, such as debugger
, allow you to pause the execution of your code and inspect variables and expressions. Use debugging statements to pause the execution of your code at a specific point, and inspect variables and expressions.
Here’s an example of using the debugger
statement to debug a function:
1function square(x) {
2 debugger;
3 return x * x;
4}
5
6square(5); // Pauses the execution of the code at the `debugger` statement.
Use Assertions: Assertions, such as assert
, allow you to check that your code behaves as expected. Use assertions to check that your code returns the expected values and meets the expected conditions.
Here’s an example of using the assert
function to check that a function returns the expected value:
1function square(x) {
2 return x * x;
3}
4
5assert(square(5) === 25, "The square function does not return the expected value.");
These are just a few debugging techniques in JavaScript. By using these debugging techniques, you can quickly and efficiently identify and fix bugs in your code. It’s important to note that debugging requires a systematic and disciplined approach, and may require a combination of techniques to effectively identify and fix bugs.
Additionally, it’s important to write clean and maintainable code, as described in the previous answer, to minimize the number of bugs in your code and make debugging easier.
Security considerations for JavaScript applications
Security is an important consideration for JavaScript applications, as JavaScript is a client-side language and is therefore exposed to potential security threats, such as cross-site scripting (XSS) and cross-site request forgery (CSRF). Here are some security considerations for JavaScript applications:
Input Validation: Input validation is the process of checking user input for correctness and security. Validate user input to ensure that it meets the expected format and values, and to prevent security threats, such as XSS and CSRF. Use libraries, such as express-validator
or joi
, to validate user input.
Here’s an example of using express-validator
to validate user input:
1const { body, validationResult } = require("express-validator");
2
3app.post(
4 "/login",
5 [
6 body("username").isLength({ min: 4, max: 16 }),
7 body("password").isLength({ min: 8, max: 32 }),
8 ],
9 (req, res) => {
10 const errors = validationResult(req);
11 if (!errors.isEmpty()) {
12 return res.status(400).json({ errors: errors.array() });
13 }
14 // Handle the login request.
15 }
16);
In this example, the express-validator
library is used to validate the username
and password
input. The isLength
method is used to check that the input meets the expected length. The validationResult
function is used to check for errors and return a JSON response with the errors.
Content Security Policy (CSP): Content Security Policy (CSP) is a security feature that allows you to restrict the sources of content that can be loaded by your application. Use CSP to prevent security threats, such as XSS and CSRF. Use the Content-Security-Policy
HTTP header to define the CSP.
Here’s an example of using CSP to restrict the sources of content:
1app.use((req, res, next) => {
2 res.setHeader(
3 "Content-Security-Policy",
4 "default-src 'self'; script-src 'self' https://trustedscripts.com; style-src 'self' https://trustedstyles.com"
5 );
6 next();
7});
In this example, the Content-Security-Policy
HTTP header is used to restrict the sources of scripts and styles. The default-src
directive is used to restrict the sources of all content. The script-src
and style-src
directives are used to restrict the sources of scripts and styles, respectively.
HTTPS: HTTPS is a secure communication protocol that encrypts the communication between the client and server. Use HTTPS to prevent security threats, such as eavesdropping and man-in-the-middle attacks. Use the https
module in Node.js or the https
protocol in the browser to implement HTTPS.
Here’s an example of using HTTPS in Node.js:
1const https = require("https");
2const fs = require("fs");
3
4const options = {
5 key: fs.readFileSync("key.pem"),
6 cert: fs.readFileSync("cert.pem"),
7};
8
9https.createServer(options, (req, res) => {
10 // Handle the HTTPS request.
11}).listen(443);
In this example, the https
module in Node.js is used to create an HTTPS server. The options
object is used to specify the SSL certificate and key. The createServer
function is used to create the HTTPS server, and the listen
method is used to start listening for HTTPS requests.
These are just a few security considerations for JavaScript applications. By following these security considerations, you can ensure that your JavaScript applications are secure and protected against potential security threats. It’s important to note that security is an ongoing process, and requires regular updates and monitoring to ensure that your applications remain secure.
Code reviews and testing
Code reviews and testing are important practices for ensuring the quality and reliability of JavaScript code. Code reviews and testing can help you identify and fix bugs, improve code quality, and ensure that your code meets the expected requirements and specifications. Here are some best practices for code reviews and testing in JavaScript:
Code Reviews: Code reviews are the process of examining and evaluating code for correctness, quality, and maintainability. Conduct code reviews to ensure that your code meets the expected quality and maintainability standards, and to identify and fix bugs. Use a code review checklist, such as the Airbnb JavaScript Style Guide or Google’s JavaScript Style Guide, to ensure consistency in your code. Use a code review tool, such as GitHub Pull Requests or Bitbucket Pull Requests, to facilitate the code review process.
Here’s an example of using GitHub Pull Requests to conduct a code review:
- Create a new branch for your changes.
- Make changes to the code.
- Create a pull request for the changes.
- Assign reviewers to the pull request.
- Review the code and provide feedback.
- Address the feedback and make changes.
- Merge the pull request.
Testing: Testing is the process of checking that your code behaves as expected. Write unit tests for your code using a testing framework, such as Jest or Mocha, to ensure that it meets the expected behavior and requirements. Test your code in isolation, and avoid testing implementation details. Write tests for all critical functionality, and ensure that your tests cover all edge cases and scenarios.
Here’s an example of using Jest to test a function:
1test("square function", () => {
2 expect(square(5)).toBe(25);
3});
4
5function square(x) {
6 return x * x;
7}
In this example, the test
function from Jest is used to define a test for the square
function. The expect
function is used to check that the square
function returns the expected value. The toBe
matcher is used to check that the returned value is equal to the expected value.
These are just a few best practices for code reviews and testing in JavaScript. By following these best practices, you can ensure that your code is of high quality, reliable, and meets the expected requirements and specifications. It’s important to note that code reviews and testing require a commitment to quality and a disciplined approach to development.
Additionally, it’s important to use a testing strategy, such as Test-Driven Development (TDD) or Behavior-Driven Development (BDD), to guide your testing efforts and ensure that your tests are comprehensive and effective.
Finally, it’s important to use continuous integration (CI) and continuous deployment (CD) tools, such as Travis CI or CircleCI, to automate the testing and deployment process, and to ensure that your code is tested and deployed regularly and consistently.
Throughout the course, it’s important to emphasize the practical applications of JavaScript in bioinformatics, such as data visualization, web-based tools, and automating workflows. Providing real-world examples and exercises will help students see the relevance of JavaScript to their field and motivate them to learn.
Additionally, encouraging students to collaborate and share their work with each other can help build a supportive learning community and foster a growth mindset. Providing resources for further learning and exploration, such as online tutorials, documentation, and forums, can also help students continue to develop their skills beyond the course.